Format Date in JavaScript
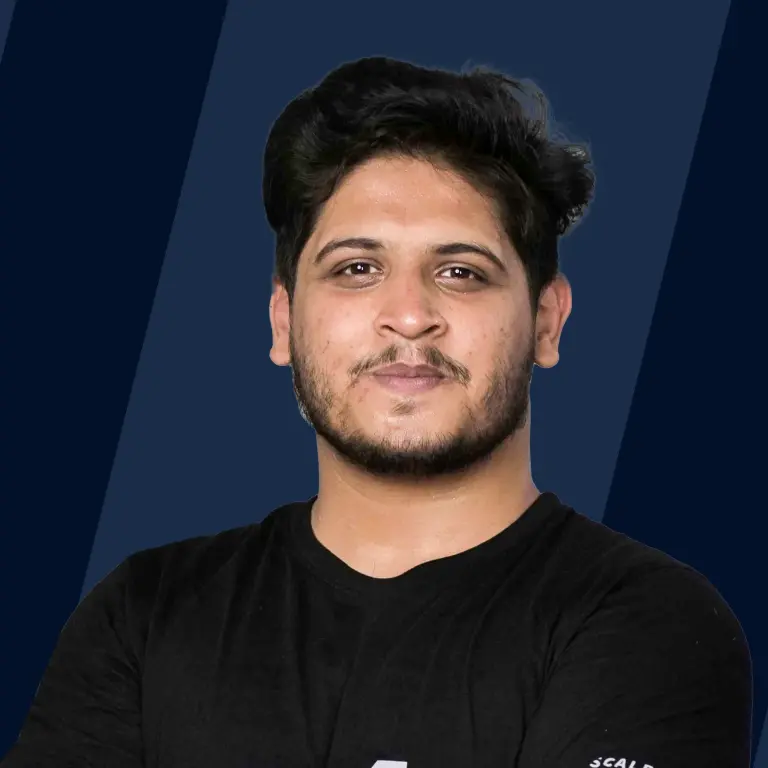
Date formatting in JavaScript involves converting dates between formats, manipulating dates, and extracting specific components like day or time. This is essential for tasks like timezone conversion or presenting dates in a user-friendly manner. JavaScript provides various libraries like Date-fns and Day.js, as well as built-in date objects and functions, to facilitate these operations. This article will explore JavaScript's date formatting methods.
How to Format Dates in JS
The Date object is an inbuilt datatype of JavaScript Language. The Date() object in javascript contains a list of methods that can be used to manipulate the date and time output as required.
The Date() function returns a string representation of the date and time.
Date():
Syntax:
Parameters: It does not take any parameters.
Returns: A string representation of the current date and time in the same way as the toString() method, which will be discussed in the next section. In this case, the date will display the day of the week and month along with the date and time, based on the browser's time zone.
Example:
Output:
Here, Date() function returns the date in string format. The date contains the day of the week and month in the strings as Thu and May respectively. GMT is the timezone of the browser.
Getting Current Date and Time Using JavaScript
JavaScript has a new Date() function that returns a new date object with the current date and timestamp according to the timezone of your browser. new Date(): Syntax:
Parameters: This method does not take any parameters.
Returns: Returns a new date object with the current date and timestamp according to the timezone of your browser. Here the returned value is an object and it is not in string format.
Example:
Output:
As we can see new Date() returns a date object in the variable date and is printed on screen using console.log(date).
Date Formatting in JS
In this section, we will format the date into different string formats using predefined functions.
1. toString() toString() method in javascript is used to display the date object in form of a String. It displays the day, month, and time zone in the date object as a string. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns date object as a string. It displays the day, month, and timezone in the date object as a string similar to the default Date() function.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. The same date is converted into a string using date.toString(). The output section displays the difference between the date object and after its conversion. Once the date object is converted into a string using the toString() method we can see the day of the week, month, and timezone as a string in the output.
2. toTimeString() toTimeString() method in javascript is used to displays only the time from the date object. It display only time and timezone according to the timezone of your browser from the date object. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns time from the date object along with its timezone.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.toTimeString() method is used to get time from the date object as a string. The output time string contains time along with timezone in string format.
3. toUTCString() toUTCString() method displays the UTC(Coordinated Universal Time) date and time of the given date object. UTC is the primary time standard by which the world regulates clocks and time. toUTCString() returns the UTC date rather than the date according to your browser. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns the corresponding UTC Time of the date object in string format.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.toUTCString() is used to get the corresponding UTC time of the date in string format.
4. toISOString() toISOString() method displays the date object in ISO date and time format. ISO is a standard format in which date and time in exchanged worldwide. ISO format has the date in YYYY-MM-DD format followed by time in hours, minutes, and seconds. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns the string which contains the ISO format of the date.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. The date is converted into ISO string format using date.toISOString(). In the output section, we can see the date in ISO format as 'YYYY-MM-DD' followed by 'HH-MM-SS'.
5. toLocaleString() toLocaleString() method takes locale(language in which you want output) as a parameter and returns date and time from the date object in the given language. If no locale is given as a parameter it takes it as 'en-US'. Syntax:
Parameters: This method takes the locale of the desired output language as a parameter. If no locale is given as a parameter then by default en-US is passed.
Returns: Returns date converted according to the given locale as a string.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. The date is converted into the locale string according to the given parameter using date.toLocaleString(). In this case, no parameter is passed hence it takes 'en-US' by default.
6. toLocaleTimeString toLocaleTimeString() method takes locale(language in which you want output) as a parameter and returns time from the date object in the given language. If no locale is given a parameter it takes it as 'en-US'. Syntax:
Parameters: This method takes the locale of the desired output language as a parameter. If no locale is given as a parameter then by default 'en-US' is passed.
Returns: Returns time from the date object in the given language as a string.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.toLocaleTimeString() is used to display time from the date object as per the given locale parameter, in form of string. In this case, no parameter is passed hence it takes 'en-US' by default. The output display time in form of the string according to 'en-US' local conventions with 'AM' and 'PM'.
Date Methods in JavaScript
Extracting a specific part of a date is an important section of format date in JavaScript. In this section, we will extract specific parts from the objects using date methods in JavaScript.
getDate()
getDate() method extracts just date (e.g. 23) from the date object. It returns day as a number (1-31). Syntax:
Parameters: This method does not take any parameters.
Returns: Return just the date i.e. which day of the month from the date object.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getDate() extracts the date from the given date object. In our example, the date is 15.
getDay()
getDay() method extracts which day of the week the date object falls on as a number. Here the representation is 0 means Sunday, 1 means Monday, and so on. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns the day of the week on which the date object falls, beginning with 0 as Sunday.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getDay() extracts the day of the week from the date object starting from Sunday as 0. In this example, the date falls on Sunday hence the function returns 0.
getFullYear()
getFullYear() method extracts the Year from the date object in 'YYYY' format. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns year from the date object in 'YYYY' format.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getFullYear() extracts the year from the given date object which is 2022.
getMonth()
getMonth() method extracts the month from the date object as a number. The number representation of months is 0-11 which means January as 0, February as 1, and so on. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns the month from the date object beginning from January as 0.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getMonth() extracts the month from the date object beginning from January as 0. In our example the month is May hence it returns 4.
getHours()
getHours() method extracts the hours (0-23) from the date object. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns hours according to the 24-Hour clock (0-23) from the date object.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getHours() returns hours from the date object which is 9 in this case.
getMinutes()
getMinutes() method extracts the minutes (0-59) from the date object. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns minutes (0-59) from the date object.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getMinutes() extract minutes from the date object which is 36 in this case.
getSeconds()
getSeconds() method returns the seconds (0-59) from the date object. Syntax:
Parameters: This method does not take any parameters.
Returns: Returns seconds (0-59) from the date object.
Program:
Output:
In the above code, we have created a date object using new Date() and stored it in constant date. date.getSeconds() extracts seconds from the date object which is 43 in this case.
The toDateString() Method in JavaScript
The toDateString() method in JavaScript returns a date from the date object in a specific format explained in this section.
Syntax:
Parameters: This method does not take any parameters.
Returns: Returns date in a specific string format. Details of the format are as follows:
- The weekday name in form of the first three letters.
- The month name in form of the first three letters.
- Day of the month in 'DD' format.
- Year in 'YYYY' format.
Program:
Output:
In the above example, date.toDateString() converts the date object to a string with a specific format. The weekday is displayed here with its first three letters, followed by the month with its first three letters, and finally the date and year. Hence, the output is Sun May 15 2022.
The toLocaleDateString() Method in JavaScript
The toLocaleDateString() method can be used to format date in JavaScript according to customized user requirements. It returns the date object using local conventions like language and format. Date and time are used in different styles across the globe. The local conventions are used such as date format in 'MM-DD-YYY' format or date and time in local languages. This method allows customizing the sections of the date object according to our choice.
Parameters: It takes language code as a first parameter which is used to return output in the specified language. The second parameter toLocaleDateString() takes is options that allow us to customize different parts of the date from the date object. The 4 basic options are as follows:
- weekday - Returns the name of the day of the week in form of short, long, or narrow.
- day - Returns day as a 2-digit number.
- year - Returns year as a 2-digit number.
- month - Returns the name of the month in form of short, long, or narrow depending on how you want it.
- hour - Returns hour as a 2-digit number.
- minute - Returns minute as a 2-digit number.
- second - Returns second as a 2-digit number.
Returns: Returns date in string format according to the given parameters.
Program:
Output:
In the above example, date.toLocaleDateString() method is passed parameters like language 'en-US' and format specifications of other date parts like the day will be numeric, the month will be short or long, and so on. The outputs are displayed according to the parameters given.
Conclusion
- Date formating and manipulation can be done using predefined functions of Date() object in JavaScript.
- We can extract specific parts of the date using the methods of the Date() function object while formatting the date in JavaScript.
- Date can be converted into strings in ISO, and UTC formats using format date in JavaScript.
- We can manipulate the display of the day, weekday, and month of date using the toLocaleDateString() method which is used to format dates in JavaScript.