Program to Find the Frequency of Characters in a String in Java
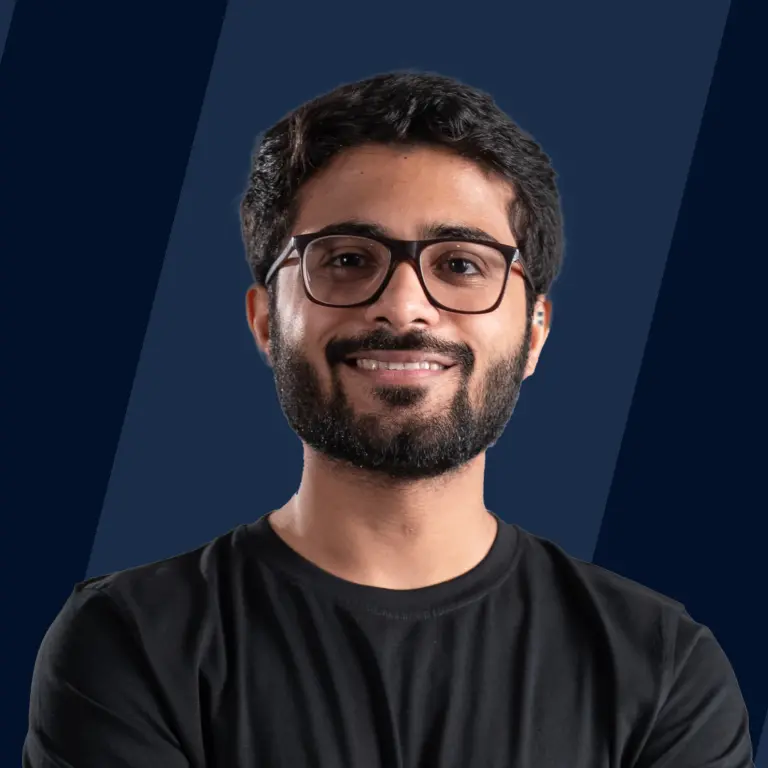
To determine character frequencies in a string, create an array, freq, of the string's length. Iterate through the string, comparing each character with others, and increment the corresponding freq element. Afterward, traverse freq to display character frequencies. For instance, the frequency of 'p' in "Picture perfect" is 2.
There are multiple ways to find the number of times each character appears in a string. They are listed below:
- Using counter array
- Using HashMap
- Using HashMap’s computeIfPresent and computeIfAbsent
Example: Find Frequency of the Specified Character
Here, we will see a simple example to count the occurrence of a particular character in the given string. It is the brute force approach by traversing the entire string and counting the number of times the specified character appears in the string.
Input: String str = “Scaler by InterviewBit” char ch = ‘e’
Output: 3
Algorithm: To achieve this, we need to follow the steps given below:
- Initialize the int variable freq as 0 which stores the frequency of the specified character.
- Traverse through each character in the string using the charAt() function and compare it with the specified character.
- If the specified character is present or matches, we will increment freq by 1.
- After iterating over the entire string, print the total occurrence of the specified character in the given string.
Time Complexity: O(n), where n is the number of characters present in the string. Auxiliary Space: O(1).
Let’s see the java program below to find the total occurrences of a particular character in the given string.
Output:
Explanation: The total number of times the character 'e' appears in the string "Scaler by InterviewBit" is calculated here. The int variable freq is used to store the frequency of the specified character. After iterating over the entire string, it prints three, i.e., the total number of ‘e’ present in the string.
Example: Find Frequency of All Characters using the Counter array
Here, we will see a simple example to count the total occurrence of each character presents in the given string using an array.
Input: String str = “welcome”
Output: c - 1 e - 2 l - 1 m - 1 o - 1 w - 1
Algorithm: To achieve this, we need to follow the steps given below:
- Create a counter array frequency of size 256 length. (The extended ASCII character set uses 8 bits, representing 256 characters.)
- As you iterate over the String, increase the value by 1 in the array at a character-based index. ( Here, traversing through each character in the string is done using the charAt() method, and we explicitly convert it into int datatype using (int) str.charAt(i). This will give us the ASCII value of the character, and then we will pass it to the frequency array and increase its value by 1. For example, if a character 'a' appears in the string, it will be frequency[97]++ as the ASCII value of 'a' is 97. This is how we increment the value in the array at an index based on the character.)
- Now, iterate through the counter array frequency and print the characters along with their frequency if frequency[i] is not 0. ( Here, the characters whose frequency is 0 mean they do not appear in the string. To print the only characters that appear in the string, we must consider the frequency of characters that are not 0.)
Iterate over the entire string, and do the same for the remaining characters as shown in the above graphic.
Time Complexity: O(n), where n is the number of characters present in the string. Auxiliary Space: O(1), as there are only 256 characters.
Let’s see the java program below to count the number of times each character appears in the given string.
Output:
Explanation: The total number of times each character appears in the string "Scaler by InterviewBit" is calculated here. The counter array arr stores the frequency of each character present in the string. After iterating over the entire string, it prints the character, along with its frequency. Here, the order in which the characters are getting printed is in ascending order. Also, the output includes ( - 2), which means the string contains two spaces.
If you want to print the characters, along with their frequencies in the order as they appear in the string and ignore the spaces, we need to follow the steps given below:
- Create a counter array freq of size same as the length of the string.
- Convert the given string into characters using the toCharArray() method and store it into a character array named ch.
- Iterate through each character in the ch array using a for a loop. Here, two loops are used to count the frequency of each character.
- Outer loop is used to select a character from the ch array and set the value to 1 at the corresponding index in the freq array.
- Inner loop is used to compare the selected character with the remaining characters present in the string.
- If a match is found, increase the value by 1 in the freq array at the corresponding index and set the duplicates of selected characters to '0' to avoid counting visited characters.
- Finally iterate through the freq array, and print the character and their corresponding frequencies.
Time Complexity: , as two loops are used, and where n is the number of characters present in the string. Auxiliary Space: O(n), as the size of the array is the same as the length of the string.
Try the program given below to achieve the above:
Output:
Explanation: Here, the total occurrence of each character present in the string "scaler by interviewbit" is calculated. The order in which the characters and their frequencies are displayed corresponds to the order in which the characters appear in the string. It also ignores the spaces present in the string.
Example: Find Frequency of Character using HashMap
Here, we will see a simple example to count the frequency of each character present in the given string using HashMap.
Input:
String str = “hello”
Output: {e=1, h=1, l=2, o=1}
Algorithm: To achieve this, we need to follow the steps given below:
- Create a HashMap, which will contain the character and its frequency as key-value pairs.
- Iterate over each character in the String.
- If the character is not present in the hashmap, use the put() method to store the character as a key and set its frequency to 1 as a value.
- If the hashmap already contains the character, fetch the existing frequency of the character and store it in the int variable count and increase the count by 1.
- Ignore characters that are equal to ' ' (white spaces) to avoid counting spaces.
- Print the character and the frequency in the same order as the characters are present in the string.
Time Complexity: O(n), where n is the number of characters present in the string. Auxiliary Space: O(n), as in the worst-case scenario all the characters present in the string can be unique.
Let’s see the java program below to find the total number of times each character appears in the given string using HashMap.
Output:
Explanation: Here, the frequency of each character present in the string “java program" is calculated using a hashmap. The hashmap stores the character as key and frequency as value. The characters and their frequencies are printed in the same order as they appear in the string. It doesn't count the spaces present in the string.
Example: Find Frequency of Character using HashMap’s ComputeIfPresent and ComputeIfAbsent
Here, we will see a simple example to count the frequency of each character present in the given string using HashMap’s methods, i.e., computeIfPresent() and computeIfAbsent().
The computeIfAbsent() and computeIfPresent() are very handy operations for adding, removing, and updating elements to/from the collection. Unlike the put() method, the compute*() methods return the current value associated with the key.
Input: String str = “hello world”
Output: {r=1, d=1, e=1, w=1, h=1, l=3, o=2}
Before jumping into the algorithm to find the frequency of characters using HashMap's methods, we need to understand how HashMap's built-in methods, i.e., computeIfPresent() and computeIfAbsent(), work.
computeIfPresent()
Syntax:
It takes two parameters:
- key - key with which the specified value is to be associated
- remappingFunction - a function that determines a new value for the specified key
The computeIfPresent() method returns the new value associated with the specified key or null if the specified key is not present in the hashmap.
computeIfAbsent()
Syntax:
It takes two parameters:
- key - key with which the specified value is to be associated
- remappingFunction - a function that determines a new value for the specified key
The computeIfAbsent() method returns the current (existing or computed) value associated with the specified key or null if the computed value is null. It means that if the specified key is not present in the hashmap, it will return the computed value, and if it is already present, it will return the existing value.
Algorithm: To achieve this, we need to follow the steps given below:
- Create a HashMap, which will store characters and their frequencies as key-value pairs.
- Iterate over each character in the String and ignore the spaces.
- If the character is not present in the hashmap, set its frequency to 1 using the computeIfAbsent() method.
- If the character is already present in the hashmap, increase its frequency by 1, and update it using the computeIfPresent() method. ( While iterating over the string, new characters are added in the hashmap using the computeIfAbsent() method, and frequencies associated with the specified characters are updated using the computeIfPresent() method.)
- Print the character and the frequency in the same order as the characters are present in the string.
Time Complexity: O(n), where n is the number of characters present in the string. Auxiliary Space: O(n), as in the worst-case scenario all the characters present in the string can be unique.
Let’s see the java program below to find the frequency of each character presents in the given string using HashMap's methods, also ignoring the spaces.
Output:
Explanation: Here, the total number of times each character appears in the string “hello world" is calculated using a hashmap’s methods. The characters and their frequencies are printed in the same order as they appear in the string. Also, it doesn't count the spaces present in the string.
Conclusion
- The frequency of characters in the string can be calculated using a counter array or hashmap and its methods.
- While using a counter array, we need to iterate through each character in the string and increase the value in the counter array (at index based on the character) if the character appears again.
- Hashmaps is used to store characters and their frequencies as key-value pairs.
- While using a hashmap, we need to iterate over the string first and the built-in put() method will add new characters as keys or update values associated with the key in the hashmap.
- The hashmap's computeIfPresent and computeIfAbsent methods are also used to find the frequency of characters present in the string.
- Frequency of characters can be used to decrypt a message using Frequency Analysis. For example, as we know that the letter E is the most frequently used letter in English text. The cryptanalyst finds the mostly-used character in the ciphertext and assumes that the corresponding plaintext character is E. After finding a few pairs, the analyst can find the key and use it to decrypt the message.