Fruitful() Functions in Python
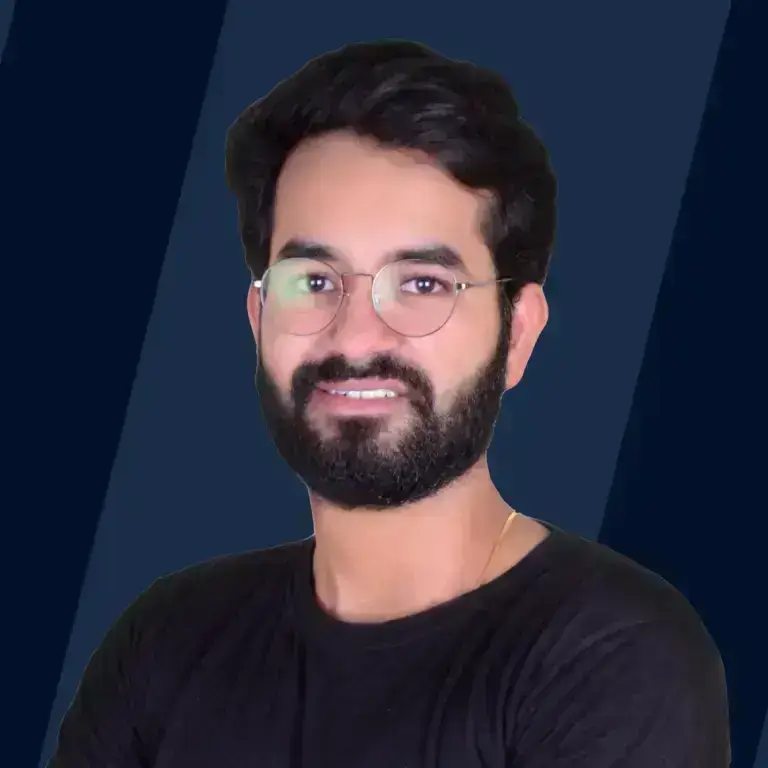
Overview
Fruitful Python functions are those that yield a result after computation. Unlike void functions, which perform a job without returning a result, productive functions add to a program's overall logic by generating an output. These routines include reusable pieces of code, which improves code modularity and maintainability.
What is a Fruitful Function in Python?
In Python, a fruitful function is like a powerhouse that not only performs a task but also hands back the required piece of information when it's done – like a loop that keeps on giving. Picture it as a function that not only does its job but also brings back a result that you can use elsewhere in your code.
Let's break it down with an example. Consider a function called multiply_numbers that takes two parameters, multiplies them together, and returns the result. Here's how it might look in Python:
Now, you can use this function, passing it two integers, and it will not only execute the multiplication but also return the result:
In this scenario, running multiply_numbers(5, 7) returns 35, which is kept in the product variable. So, a productive function does more than just complete a job; it also returns a result that may be captured and used elsewhere in your code. It's like receiving a two-for-one deal: the function accomplishes its job, and you get a good return. That's the magic of Python's productive functions!
Dead Code
Dead code in Python refers to lines or blocks of code that exist in a program but are never executed. It's like having a dusty old book on your shelf that you've never opened. On the other hand, a fruitful function is one that not only serves a purpose but also adds value to your code.
Consider this Python example:
In this example, the calculate_cube function is dead code because it is never called by the main function. It's like having a recipe for a meal you've never made. Removing dead code is critical for keeping programs clean and efficient.
So, the next time you analyze your code, keep an eye out for any dead code. It not only creates clutter, but it may also cause misunderstanding. Accept productive functions that add value to your program's logic. It's like ensuring that every component in your code works efficiently!
Example of Fruitful Function in Python
In Python programming, productive functions stand out as captivating magicians that weave magic into our code. Imagine a function that not only performs duties but also bestows wonderful gifts on us; this is the core of a productive function.
Consider this: you construct a function, and instead of simply doing a collection of activities, it gracefully returns a result. That outcome, my buddy, is the product of the function's efforts, and it might range from a simple integer to a complicated data structure.
Let's look at this example to help you understand the concept. Consider a function that determines the square of a given number:
Here, the function calculate_square accepts an integer as input, conducts the magical squaring operation, and kindly returns the squared result. Voila! You've just watched a productive function in action.
Fruitful functions improve code clarity while simultaneously encouraging reusability, making them a useful tool in Python development. So, embrace the magic, unleash the power of useful functions, and watch your code spring to life with elegance and efficiency.
Void Functions
In Python, void functions and fruitful functions perform various roles in programming, enhancing code efficiency and clarity. Let's look at each.
Void functions, as the name implies, do not return anything. They do a series of activities and run commands without creating any results. These functions are critical for code modularization, reusability, and improved code organization. Consider this void function, which outputs a greeting message:
Fruitful functions, on the other hand, are intended to return a value and provide the caller with information. These routines are very handy when you want a result for further processing or presentation. Here's a straightforward illustration of a useful function that adds two numbers:
Understanding when to use void or fruitful functions allows programmers to design more modular and efficient code, which improves the clarity and maintainability of their Python programs.
Parameters in Fruitful Functions in Python
In Python, understanding parameters in fruitful functions is key to crafting efficient and dynamic code. Parameters serve as variables that get values when the function is invoked, enabling us to tailor the function's behaviour. Let us go into this essential topic.
In a vast array of fruitful functions, parameters act as input channels, allowing your functions to process a wide range of inputs. They behave as placeholders, anxiously awaiting values when the function is called. Consider a function for calculating the area of a rectangle. Here, length and width are parameters that require particular values to get a valid outcome.
Parameters are defined by declaring them within the function's parentheses and providing their names and types. These inputs convert simple programs into diverse tools capable of handling a variety of datasets. Python's versatility shows through because it enables default parameter values, which adds an added layer of adaptability.
When you call the function, you pass the actual values (arguments) for these parameters. This dynamic interaction of parameters and arguments enables your functions to respond to individual demands, resulting in code that is both functional and highly reusable.
So, understanding arguments in useful functions allows you to write Python code that is both powerful and customizable, making your programming experience more straightforward and efficient.
Function Composition
Function composition in productive functions is a powerful Python programming technique that enables you to combine many functions to build more complicated and reusable code. It's like building blocks for your code, with each function performing a specific task that can be effortlessly integrated to get the required result.
Assume you have two functions, say multiply_by_two and add_five, each meant to do a certain mathematical operation. Function composition allows you to connect these two functions to form a new function, combined_function. This produces a single function that multiplies an input by two and then adds five to the output.
Using function composition makes your code more modular and easier to maintain. You can reuse existing functions to increase code reusability and efficiency in your programs. This method improves code readability and promotes a clean, organized coding style.
So we can say that, function composition simplifies large processes by dividing them into smaller, manageable functions, resulting in a more structured and maintained codebase in Python programming.
Local and Global Scope
Local scope refers to variables defined within a given function. These variables are specific to that function, providing encapsulation and limiting unintentional interaction with other portions of your program. Global scope, on the other hand, refers to variables that are declared outside of any function and hence available across the codebase.
In the context of fruitful functions, local scope becomes pivotal. Variables specified within the function are only valid during its execution, ensuring a controlled computing environment. When a variable is required outside of the bounds of a given function, global scope is invoked, allowing it to be used across the program.
In simple terms, mastering local and global scope in fruitful functions empowers Python developers to write efficient and organized code. It guarantees that variables are used only when necessary, hence improving code readability and maintainability. So, the next time you begin on a Python coding adventure, keep these scope distinctions in mind for a more efficient and productive development experience.
Recursion
When exploring the field of Python programming, one comes across the fascinating idea of recursion in productive functions. Consider a function calling itself in an infinite cycle of self-discovery. Let's dispel some of the mystery behind this wonderful phenomenon.
In Python, a fruitful function returns a value. When this approach is combined with recursion, it results in a compelling dance of logic and iteration. Consider a function that not only completes a task but also generates additional instances of itself to contribute.
The beauty is in simplicity. Consider a case in which a function takes a recursive path rather than a linear one. It ingeniously breaks a big problem into smaller, more manageable chunks and solves them progressively. Each recursive call adds a layer to the story until the conclusion is revealed.
Syntax highlighting is like shining a spotlight on code, making it look nice and easy to read. The Editor.copyWithSyntaxHighlighting acts like a director, ensuring the code runs smoothly.
In Python, recursion in fruitful functions is not just a programming technique; it's a narrative that unfolds with every recursive call, revealing the elegance of a well-crafted story in code. Take on the enchantment and let your functions tell tales of computation in Python's unique recursive language.
Exception Handling in Fruitful Functions
Exception handling is critical to ensure the resilience of Python programs, particularly when dealing with complex functions. Fruitful functions in Python deliver a result when they are successfully executed; but, what happens when unexpected failures occur? This is where exception handling comes into play.
Consider a scenario where a fruitful function encounters an unforeseen issue, like division by zero or an invalid input. Python has a technique called try-except that allows you to gracefully handle such scenarios. Wrapping the dangerous code in the try block enables the interpreter to identify exceptions. If an exception is thrown, the flow gently switches to the except block, preventing the program from crashing.
Here's a simple example:
In this code snippet, if a ZeroDivisionError occurs, a specific message is printed, and for any other unexpected errors, a generic message is displayed. This approach not only ensures the reliability of your code but also provides valuable insights into potential issues during development and debugging. Exception handling in fruitful functions exemplifies the Pythonic philosophy of graceful degradation in the face of unexpected challenges.
FAQs
Q. What are Fruitful Functions in Python?
A. Fruitful Python functions are those that yield a result after computation. Unlike void functions, which perform a job without returning a result, productive functions add to a program's overall logic by generating an output. These routines include reusable pieces of code, which improves code modularity and maintainability.
Q. How do I Create Fruitful Functions?
A. Creating useful functions entails defining a function using the def keyword, setting arguments, and using the return statement to return a value to the caller code. Users may maximize the effectiveness of successful functions by designing them to receive inputs, process them, and then provide a meaningful output. This improves coding efficiency and readability.
Q. Can Fruitful Functions Return Multiple Statements?
A. Yes, fruitful functions in Python can have many return statements. The function will finish as soon as a return statement is invoked, giving you the freedom to handle many circumstances inside the same function. This adaptability helps developers construct functions that cater to varied contexts, contributing to stronger and more adaptable code.
Conclusion
- Python functions provide a pathway to code modularity. Encapsulating certain operations into functions results in modular building blocks that improve code readability and maintenance. This modularity makes debugging and troubleshooting easier, promoting a smooth coding experience.
- The appealing aspect of reusability is revealed through Python functions. Once created, these pieces of logic become useful tools that may be used anywhere required. This not only reduces redundancy but also promotes the DRY (Don't Repeat Yourself) coding concept, which improves efficiency and saves valuable development time.
- Python functions adapt fluidly to a variety of contexts, accepting multiple inputs and providing appropriate results. This flexibility encourages scalability by allowing your program to develop gracefully as project needs change over time.