fseek in C
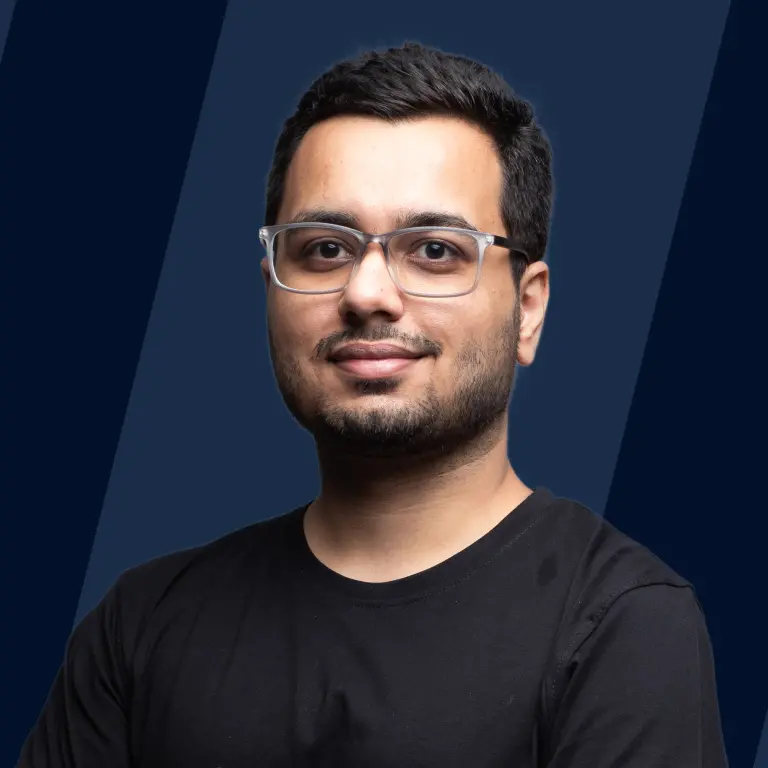
Overview
Sometimes in our program, we are in a use case of working with different files, C language provides us with a variety of different functions to ease this task. One of such functions is fseek(). The function fseek() is a standard library function in C language, present in stdio.h header file. It is used to move or change the position of the file pointer which is being used to read the file, to a specified offset position.
Syntax of fseek() in C
Parameters of fseek() in C
The function fseek in c receives three parameters
- filePointer : pointer to the FILE object that identifies the stream, which we have to modify.
- offset : number of bytes to shift the position of the FILE pointer, relative to its current origin to determine the new position.
- origin : determines the current position of the FILE pointer from where the offset needs to be added ie. it indicates the contemporary position of the file pointer from where the specified offset value will be added, in order to move the position of the file pointer using the fseek() function.
The parameter origin can have the following three values
- SEEK_END : denotes the end of the file
- SEE_SET : denotes the starting of the file
- SEEK_CUR : denotes the current position of the file pointer.
Return Value of fseek() in C
On successful execution the function fseek in C return 0 ie. if the function fseek() was successfully able to move the pointer, it returns 0, else if some error or failure has occurred, it will return a non-zero value.
Example
In the below example, we are moving the file pointer to 4 offset from its current position which is SEEK_SET, meaning the start of the file, and then we printing the file pointer's location using the ftell() function.
The input file contains
Output:
Explanation -
- We are declaring a file pointer filePointer and are opening the test.txt file in read mode (assuming the file exists).
- Then using the ftell() function we are printing the position of the file pointer ie. where in the file, the file pointer is currently pointing to, with respect to the start of the file.
- Now using fseek(), we are setting the origin as SEEK_SET (meaning the start of the file) and are saying to move the file pointer 4 bytes from here ie. move the file pointer 4 bytes from the start of the file.
- Finally we are again printing the situational current location of filePointer using the ftell() function.
Exceptions of fseek() in C
The function fseek in c should not be used to compute the size of regular files, as a binary file stream may not meaningfully support the fseek() call with an origin value of SEEK_END because of possible trailing null characters, which means, setting the file position to the end of the file, in a binary file stream like this
may result in some undefined behavior.
Note - If the passed value of offset is greater than the actual number of characters in the file, ie. if the passed offset value is greater than size of the file (in bytes) then also the function fseek() will move the position of the file pointer to the specified offset with respect to the passed origin, irrespective of the number of character entries in the file.
The reason for this is, as the function fseek() is also used to write content into a file from any specified location, and hence any offset value will be considered valid, as the possible case can be, if the user wants to write into a given file from that particular location which is passed as the offset.
What is fseek() in C?
fseek() is a standard library function in the C language majorly used while working with the file streams, it is present in the stdio.h header file. It is used to move or change the position of the file pointer to a specified offset ie. moving the file pointer by a certain value from its current position, and alongwith that, the function can be used to write data at any desired location in the file, we can also use it to compute the file size of non-binary files.
More Examples
1. Calculating the File Size of a Non-Binary File Using the Function fseek in c
In this example, we will be calculating the size of non-binary files using the fseek() function, why only non-binary? Because as explained above, fseek() should be avoided to calculate the size of binary files, as while setting the origin parameter as SEEK_END, the function fseek() may result in some undefined behavior, because of the possible trailing null characters in them.
Here, we are given with a file, and now to calculate its size, we will simply define a file pointer and will make that point to the end of that file using SEEK_END with the offset value as 0, and then using the function ftell() we will get the file pointer's location and that will be the file size.
The input file contains
Output:
Explanation -
- We are declaring a file pointer filePointer and are opening the test.txt file in read mode (assuming the file exists).
- Using fseek(), we are setting the file pointer's location to point to the end of the file with 0 offset ie. now filePointer is pointing to the end of the specified file.
- Now using ftell(), we are determining the current position of the file pointer to compute the file size, as the total number of bytes the file pointer has traveled in its journey of reaching the end of the file will be the required file size.
2. Writing into a File Starting from a Specified Position Using the Function fseek in C
In this example, we will be writing into a file using the fseek() function, starting from a specific location.
Here, we will firstly use the fseek() function to move the file pointer to the location of our choice, and then we will write the data into that file through the file pointer using fputs() function.
File content before executing the code
File content after executing the code
Explanation -
- We are declaring a file pointer filePointer and are opening the test.txt file in w+ mode ie. in both read and write mode (assuming the file exists).
- Then we are writing a string into the file with the help of our filePointer.
Note - This will erase all the currently written data in the test.txt file and after that, it will write the passed string, the reason for happening so is, since the filePointer will be pointing to the start of the file by default and as soon as we instructed it to write a particular string into the file, it will erase all the data from there ie. from where it is pointing to, till the end of the file, and then after it will write the passed string.
- Now, using fseek() we are setting the origin of file pointer again to be start, but this time with an offset value of 8 ie. now the filePointer is pointing to 8 bytes ahead from the start of the file.
- Now again we are writing a string into the file with the help of the filePointer, but as the pointer is currently pointing to 8 bytes ahead of the start, all the data starting from that position to the end of the file will be erased, and the second string will be written there.
- And hence finally we will have the output as : Writing Writing second line into the file.
Conclusion
- fseek() is a file stream standard library function in C language, present in the stdio.h header file, it is used to change the position of file pointers.
- Syntax : fseek(FILE *filePointer, long offset, int origin); It takes three parameters, a FILE pointer to identify the stream, an offset value to determine the number of bytes to shift the position relative to its current origin, and an origin parameter to determine the starting position of the filePointer.
- On successful execution, the function fseek() returns the value 0, else a non-zero value otherwise.
- Using fseek() we can calculate the size of non-binary files, we can write data into a file starting from any specific location, and more.
- Usage of fseek() should be avoided to calculate the size of binary files, because of the possible trail of null characters in them.