Function Overriding in C++?
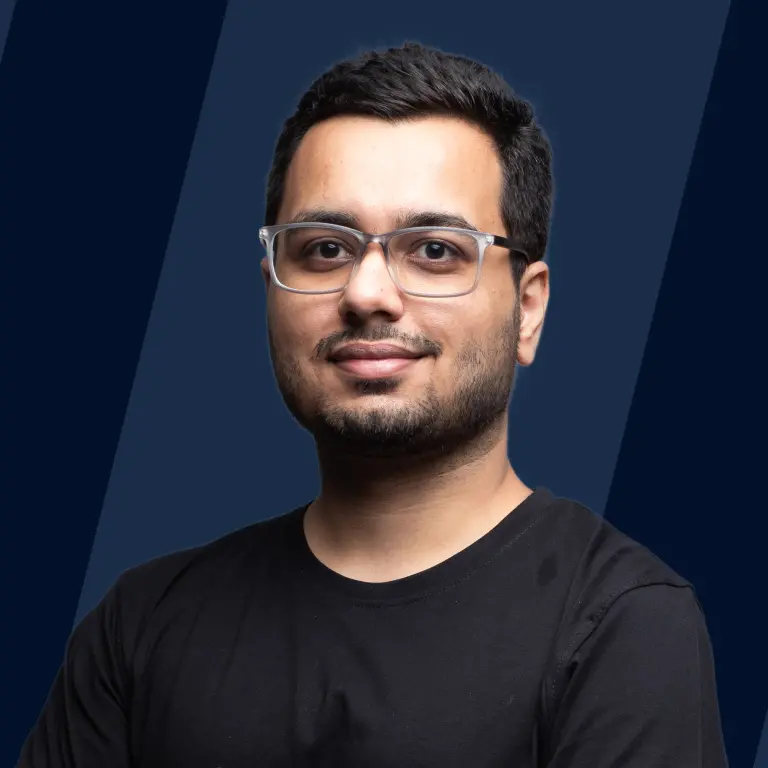
Defining a Function
Imagine we are writing a code to store the result of the final exams of a class. while we are at it, we encounter a situation where we are given the marks scored by the student and we have to display the percentage. Now, this can be done by the following mathematical calculation: (marks_scored/total_marks)*100, where marks_scored is the marks scored by the student, and total_marks is the total marks in the test.
Thus the above calculation will return the percentage for the student. Now suppose there are 100 students in the class, thus we will have to write the same piece of code 100 times to get the percentage for each student. This will be time-taking as well as fill the code with repetitive blocks.
A function in C++ is defined as a group of statements that together can perform a task. By default, every C++ program has at least one function, that is main(). We can declare functions in C++ and divide up our code into separate functions.
A function declaration tells the compiler about a function's name, return type, and parameters. A function definition provides the actual body of the function.
Following are the elements of function declaration in C++:
1. General Syntax
The following is the general syntax of a function in C++.
2. Return Type
The return type of a function is the type of data that is returned upon the function call. The return type of a function is the same as the datatype.
A void type function does not return anything.
Example:
In the above code, the func1 will return ans which will be of int data type, whereas the func2 will not return anything.
3. Function Name
The function name is the salutation given to a function using which it is called in further programs. The function names in C++ are user-defined.
The following are some conventions that a developer needs to follow while naming a function in C++:
- A function name in C++ should not start with a number
- A function name in C++ should not be separated from space. A general practice is to write names in camel case (e.g. funcName) or separate names using underscore (e.g. func_name).
4. Parameters
A parameter is a named variable passed into a function. Parameter variables are used to import arguments into functions.
The C++ function can be called either with zero, or more than zero (i.e. multiple) parameters.
5. Function Body
The function body contains the code section of the function. It is comprised of two curly braces {...}.
6. Calling a Function
Now that we've learned about the functions in C++, let us see how to call a function in C++.
- Calling a void function in C++: A void function in C++ is called by simply calling the function name followed by brackets. In case the function contains parameters, we should pass the values of the parameters in the same order.
- Calling a non-void function in C++: A non void function in C++ is a function that returns a certain data type. Thus when a non-void function is called, it is similar to calling a void function, i.e. by simply calling the function name followed by brackets, but the difference in the result of the function call should be stored in a value of the same data type. In case the function contains parameters, we should pass the values of the parameters in the same order.
What is Function Overriding in C++?
Before we get into function overriding in c++, let us have a brief overview of inheritance in c++.
In C++, inheritance is a process in which one object acquires all the properties and behaviors of its parent object automatically. In such a way, you can reuse, extend or modify the attributes and behaviors which are defined in other classes.
The function overriding concept exists due to the inheritance in C++. The function overriding in C++ aids us to use a function with the same name, that is in the child class that is already present in its parent class.
The function overriding in C++ is used to achieve runtime polymorphism. Runtime polymorphism is also known as dynamic polymorphism or late binding. In runtime polymorphism, the function call is resolved at run time. In contrast, to compile-time or static polymorphism, the compiler deduces the object at run time and then decides which function call to bind to the object.
Syntax
The Child class inherits the Parent class thus the func also gets inherited. After that func with a new definition is created within the child.
Example
Output:
Explanation of the example:
In the above example, the Animal class has a sound() method that outputs "Animal sound". Now we are declaring a Lion class that is inheriting the Animal class. In this Lion class, we are again creating a sound() method which will output "Roar".
When the sound() method is called upon 'a' object, it will output "Animal sound". Now When the sound() method is called upon the 'l' object, it will override the sound() method of the Animal class by the method of the Lion class and output "Animal sound".
How Does Function Overriding Work in C++?
As discussed earlier, function overriding in C++ is when a function that is already defined in the parent class is again defined in the derived class or child class. In this section, we will learn how the function overriding in C++ works.
The parent class is the class being inherited from, also called a base class. A child class is a class that inherits from another class, also called a derived class.
When a class is inherited by another class in C++, it also inherits all of its methods. Thus if suppose there is a method defined in the parent class, it can automatically be used in the child class.
When a class is defined, only the specification for the object is defined; no memory or storage is allocated. To use the data and access functions defined in the class. Thus, when a function is called over an object, it checks if the function is defined in the associated class. If the function is defined in the class it would execute the function. In case the function is not defined in the class, since child functions have access to the function of the parent class, it will execute the function body that is defined in the class.
What is the need for Function overriding in C++?
Imagine you are supposed to make classes for two different models of a car. Since both the classes will have a lot of features in common, you have decided to make a parent class Car and inherit those properties into your individual classes.
Now suppose you have two classes Volkswagen and Mercedes that inherit the Car class. The Car class has a function that displays the color of the Car (which by default is white). Now in your individual classes, you have defined methods to get the colorName. * Thus, in this case, we will override the displayColor function to display the color input by the user.
Examples
In this section, we will see the detailed code explanation of function overriding in C++.
Example 1: C++ Function Overriding
Output:
Example 2: Accessing Overriding Function
Example 3: Accessing Overridden Function
Output:
Example 4: Calling Overridden Function Using Pointer
Advantages of Function Overriding
Following are the advantages of function overriding
- The function derived from a parent class can be restructured in a child class thus aiding us to change the function definition according to the requirement.
- Helps in the reusability of the function.
- Helps to maintain the readability and consistency of the code.
How to Access Overridden Functions in C++?
There are two ways to access overridden functions in C++ through the derived class:
- By using pointers
- By using scope resolution operators
By using pointers
We can use pointers to create the reference to the overridden function.
Syntax
By using the scope resolution operator
In order to access Overridden function in C++, we can use the :: (scope resolution operator).
Syntax
Following is the pictorial representation of accessing overridden function.
Function Overloading vs. Function Overriding
The following table shows the difference between function overloading and function overriding:
Function overriding | Function overloading | |
---|---|---|
Inheritance | Function overriding in C++ occurs only when one class is inheriting another class. | Function overloading in C++ can occur without inheritance. |
Function Signature | In function overriding in C++, the signatures of the function must be the same, i.e. if we are overriding a function then the function that is being written must have the same parameters as the one which it is overriding. | In function overloading in C++, the function signature must be different. |
Scope of functions | Since function overriding in C++ is done on a function in a parent class by a function in a child class, thus the scope of the function is always different. * Scope defines where variables can be accessed or referenced*. In the case of function overriding, if a function X is being overridden then the function definition which is overriding the function X is never in the same class as X thus making the scopes different. | in function overloading in C++, the scope of the function is always the same. |
Behavior of functions | The function that is overridden are the functions that have the same names as the original functions but they have different internal implementation. | The functions that are overloaded are primarily used to act differently according to the parameters that are being passed. |
To learn about the difference between function overriding and function overloading please refer to this article.
Conclusion
- Function in C++ is defined as a group of statements that together can perform a task.
- The function overriding in C++ aids us to use a function that is in the child class that is already present in its parent class.
- The function overriding in C++ is used to achieve runtime polymorphism.
- Function overriding in C++ occurs only when one class is inheriting another class.