How to Get the Current Date in JavaScript?
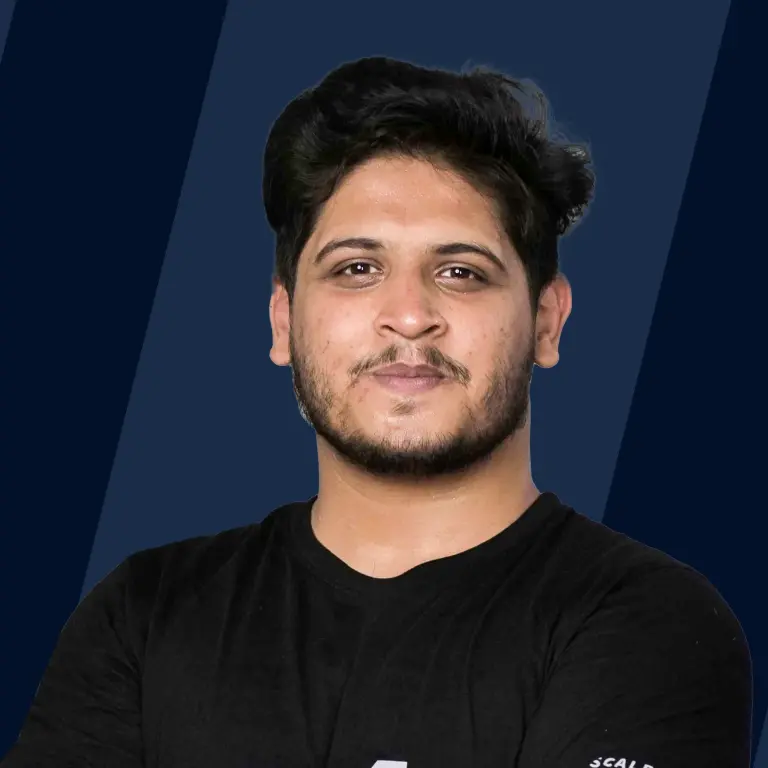
Overview
Dates are integral to programming and crucial for tracking events and organising tasks—especially in JavaScript, which equips developers with tools and libraries to manage dates. Whether you're crafting a calendar, scheduling system, or timestamping blog posts, understanding how to retrieve the current date, year, and month is essential. This guide simplifies these concepts, ensuring you can seamlessly integrate date functionalities into your dynamic web projects.
How to Get the Current Date in JavaScript
In JavaScript, we can get the current date by using the new Date() object.
-
It is an inbuilt data type that works with dates and times.
-
The Date object represents a date and time with millisecond precision within 100 million days before or after 1/1/1970.
-
We can create the Date object by using thenew keyword.
- Syntax:
- Output:
The date object reflects the current system time on the computer on which the browser is running. It is also dependent upon the browser.
- By default, it uses our browser's time zone and displays the date as a full-text string, such as "Wed Aug 17 2022 9:11:53 GMT+0530 (India Standard Time)" that contains the current day, date, time, and time zone.
Let's look at how we can get the current date out of this long string. We will improve its readability by utilizing some JavaScript methods that work with date objects.
How to Use JavaScript Date Methods
The date object supports numerous date methods. To get the current date, we will use the following methods :
-
getFullYear() The method returns the year of the specified date as a four-digit number (YYYY).
Syntax:
Example:
OUTPUT:
-
getMonth() Using the getMonth() method, we can get the month number from a given date object (0-11). It has a zero-based index.
Syntax:
Example:
OUTPUT:
-
If we want to show the month in the format of MM, i.e., 08. The built-in date class doesn't offer formatting features, unlike other libraries. We need to come up with a solution. We can use Javascript's methods on strings like padStart(length, substring). The pad puts before our string with the substring before it until it reaches a certain length. To put 0 before 8, we will pad our string with "0" until it reaches length 2. Since we are using the String methods, we need to typecast the month type number to a String.
We can use the same method for getDate() also.
-
-
getDate() It fetches the date of a month from a given Date object. It returns the day as a number (1-31).
Syntax:
Example:
OUTPUT:
NOTE : To get the current day we will use the getDate() method. The getDay() method returns the day of the week, not the current day number.
Now, let's combine all of these methods to get current date in JavaScript.
Output:
Let's look at some other methods to get current date in javascript.
Using toUTCString()
In addition to the date and time, the toUTCString() method also returns the day of the week as a string in Universal Coordinated Time (UTC).
Syntax:
Example:
Output:
We can use slice() on the toUTCString method to get the current date in Javascript. Since it returns the date object as a string, there is no need to typecast the date.
Output:
Using now()
The method now() returns the milliseconds elapsed since the UNIX epoch. Epoch time represents the number of milliseconds passed since January 1st, 1970.
Output:
To get current date in javascript, we can pass this time to the date object.
Output:
The date object reflects the current system time on the computer on which the browser is running. Use this JavaScript Compiler to compile your code.
How to Use the toJSON() Method
The toJSON() method returns a yyyy-mm-dd format alongside the time format, hh:mm
Output:
The T serves as a literal to separate the date from the time, and the Z denotes zero hour offset also known as Zulu time (UTC).
To get current date in javascript we will use the slice() string method. The slice method extracts the specified string.
Output:
How to Use toLocaleDateString()
The toLocaleDateString() method returns the date object as a string using local conventions. In addition, it has the option to pass the desired language as an argument and has simple formatting options for weekdays, months, and days.
Example a): Let's call the method without any arguments:
Output: The output depends on the locale. It is in the Indian date format.
Example b): We can define a specific locale by passing it as a string argument.
Output:
The en-IN is a locale code for India. We can find the locale codes here.
Example c): We can customize the date using the options arguments.
Only month and weekday can be long or numeric. Day and year can only be numeric:
Output:
How to Use Moment.js
The moment is a javascript library designed for dealing with time and date.
Make sure you install the moment.js package before using it.
The following code shows how to get the current date in javascript using moment.js:
Output:
Moment.js offers a wide variety of date formats which we can choose from its documentation.
Don't miss this opportunity to become a certified JavaScript expert. Enroll now in our JavaScript course and gain a competitive advantage in the programming world.
Conclusion
- JavaScript offers a variety of methods and external libraries to get the current date.
- We can get current date in javascript by using the new Date() object.
- The date object supports numerous date methods like getFullYear(), getMonth(), getDate().
- The toUTCString() method returns the date object as a string in UTC.
- The method now() returns the number of milliseconds passed since January 1st, 1970.
- The toJSON() method returns a yyyy-mm-dd format alongside the time format, hh:mm
.ms. - The toLocaleDateString() returns the date as a string in a specific locale.
- Moment.js is a Javascript library that works with date and time.