Python Program to Get Current Date in List
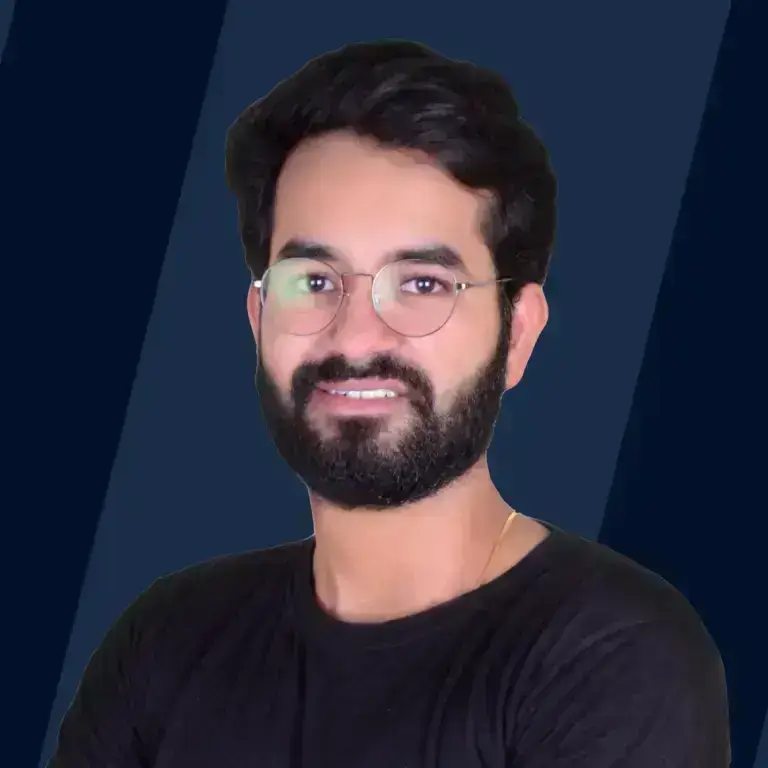
Overview
In this article, we will explore how to get the current date in Python. There are some methods to get the current date in Python, like date.today(), datetime.now(), and now().date(). A date is an important component of any website, database, or application, as it indicates the time and day that a program was created, website information was stored, or the application version was released. When a customer uses a website or program, the software records their data with a specific date and time. As such, the date and time are important in the world of software.
Get Current Date Using Python
In this article, we will be taking a look at how to get the current date in Python.
Introduction
There might be times when you want to get the current date and use it in your program. For example, you might want to create a file with the current date in its name. Or you might want to log the current date and time to the console.
Whatever the reason, there are different ways to get the current date in Python. In this article, we will take a look at some of these ways.
Time, as well as Date, are not data types in Python. There is a module termed DateTime which can be loaded to operate with both the date as well as the time. There is no requirement to import the Datetime package outside because it is built into Python. The DateTime module has some methods for obtaining the current date and time.
Prerequisites
To follow this guide, you will need:
- Python 3 installed on your local machine.
- Access to the terminal/command line.
- A text editor. If you don't have one installed, we recommend using Visual Studio Code.
Different Ways to Get Current Date in Python
There are a few different ways to get the current date in Python. We will go through some of these ways in this section.
- Python gets today's date using date.today() method
- Python gets current date in different formats using today.strftime() method.
- The now().date() method in Python can also be used to utilized to get the current date as well as time.
Using date.today()
The date.today() method returns the current date as a date object containing the present day's date.
Syntax
Parameters
This method doesn't take any parameters.
Return
The current local date is returned as an output.
Example
Let's take a look at an example.
Code:
Output:
Output Explanation:
In this example, we imported the date class from the datetime module. We then used the date.today() method to get the current date and printed it to the console.
As you can see, the current date is printed as 2022-10-24.
Using datetime.now()
The datetime.now() method returns the current date and time as a datetime object.
Syntax
datetime.now()
Return
Returns the current date and time.
Attributes of now() method
The now() method has the following attributes:
- now().year: Returns the year
- now().month: Returns the month
- now().day: Returns the day
- now().hour: Returns the hour
- now().minute: Returns the minute
- now().second: Returns the second
- now().microsecond: Returns the microsecond
Example1
Let's take a look at an example.
Code
Output
Output Explanation:
In this example, we imported the datetime class from the datetime module. We then used the datetime.now() method to get the current date and time and printed it to the console.
As you can see, the output is printed as 2020-05-28 16:51:23.026663. This includes the date, time in the format of hour:minutes:seconds
Exmaple2
Let's take a look at an example
Code:
Output
Output Explanation:
In this example, we imported the datetime class from the datetime module. We then used the datetime.now() method to get the current date and time and printed it to the console. now() provides a set of attributes, and each attribute represents a particular unit of the current timestamp. Using .year provides the current year, using .month provides the current month, the .day provides the current day, and so on.
Using now().date() Method
A now().date() method is used to access the local or current date of the system by importing the datetime module in Python. If you only want the date and not the time, you can use the now().date() method. This method returns the current date.
Syntax
datetime.now().date()
Parameters
This method doesn't take any parameters.
Return
Returns the current date.
Example
Let's take a look at an example.
Code
Output
Output Explanation:
In this example, we imported the datetime class from the datetime module. We then used the datetime.now().date() method to get the current date and printed it to the console.
As you can see, the output is printed as 2022-10-24. This is the current date.
Date and Time Formats in Python
In Python, you can use different ways to format the date and time. For example, you can use the strftime() method.
The strftime() method takes in a format string and returns a formatted date and time string.
The following are the many date and time formats that can be used to obtain the proper representation of datetime in Python by supplying the parameters to the strftime () method.
Syntax
datetime.now().strftime(format)
Parameters
The format parameter is the format string that specifies the format of the outputted date and time string.
Return
Returns a formatted date and time string.
Example 1
Let's take a look at an example.
Code
Output
Output Explanation:
In this example, we imported the datetime class from the datetime module. We then used the datetime.now().strftime() method to get the current date and time as a string. The format string we used was "%d/%m/%Y". This formats the date as day/month/year.
Example 2
Let's take another example.
Code
Output
Output Explanation:
In this example, we again imported the datetime class from the datetime module. We then used the datetime.now().strftime() method to get the current date and time as a string. The format string we used was "%a, %d %B %Y". This formats the date as the day of the week, day of the month, month, and year.
Conclusion
In this article, we have looked at how to get the current date in Python in different ways. We have also looked at how to format the date and time.
- The date.today() method returns the current date as a date object containing the present day’s date.
- The datetime.now() method returns the current date and time as a datetime object.
- A now().date() method is used to access the local or current date of the system by importing the datetime module in Python.
- In Python, you can use different ways to format the date and time.
- The strftime() method takes in a format string and returns a formatted date and time string.