Program to Get File Extension in List in Python
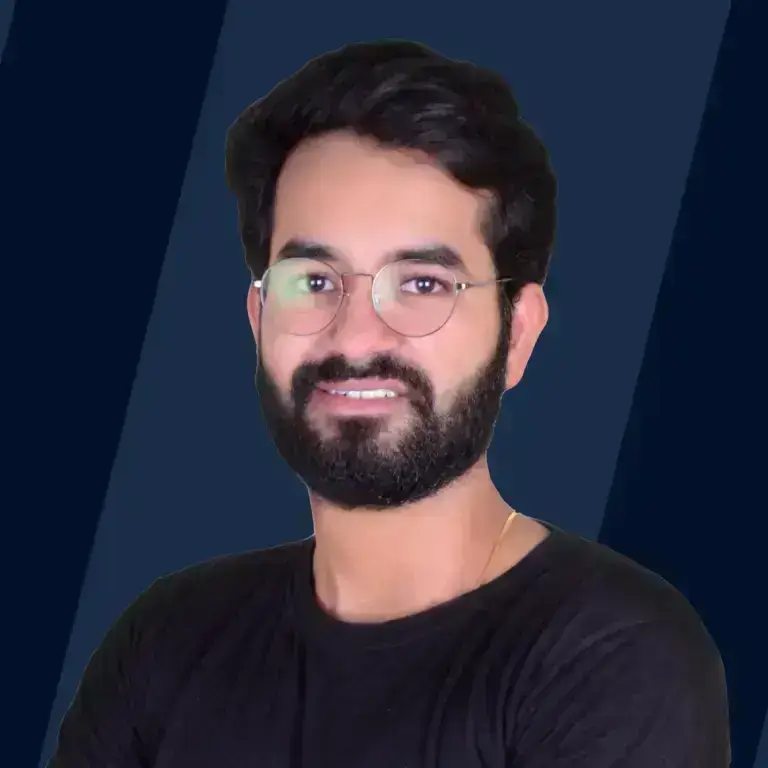
Overview
In any operating system, for every file or folder, there exists a path to that file or folder. But when we look for a file, there always exists an extension for the file. Let us say we are working in Python, therefore we store the file with the .py extension, and if we are working in HTML or CSS files, we will save that file with extensions .html and .css respectively. To get file extension in Python, we first have to find out the path of the file, so for getting the path of a file in Python, there are several libraries like os and pathlib.
Get File Extension in Python
Introduction
Before we get into how to get file extension in Python. First, we will see, what is file extension and how the file is created. In the different operating system, there is different way of creating a file, and after creating a file, we have to define what kind of file it is, whether it's a doc file, Python file, HTML file or xlsx file. So, for defining the extension of that particular file, we have to save that file with its particular name. Let us suppose we need to create a Python file, we gave that file name abc, but when renaming the file with abc, our operating system does not know what is abc, for specifying it as a Python file, we add a suffix after the name of the file that its py for Python. So, our file name will be abc.py, so now our operating system will confirm that it's a Python file.
Now, we need to learn how to extract that file extension py from the path of the file. We will be using Python for extracting the extension of the file. There are several methods:
- Os.path module
- pathlib module
Pre-requisites
The prerequisites need to get the file extension in Python are:
Different Ways to Get File Extension in Python
OS Module
This OS module has extensive functions for interacting with the operating system. This module can be used to easily create, modify, delete, and fetch file contents or directories.
In the OS module, there is a path class that has pre-made useful utility functions to manipulate OS file paths. path class of the OS module includes the opening, saving and updating, and getting the information from file paths.
In os. path, there is a splitext() function that allows you to separate the root and extension of a specified file path. A tuple made up of the root string and the extension string is the function's output. If you want to get file extension in Python, the splitext() function is the way to go.
For e.g ->
path name | root | extension |
---|---|---|
user/Desktop/folder/abc.txt | user/Desktop/folder/abc | .txt |
user/Desktop/folder | user/Desktop/folder/abc | |
user/Desktop/pat.py | user/Desktop/pat | .py |
Syntax:
Parameters:
-
path:
It is an object representing the file path. It can be a string or bytes object representing a path.
Return Type:
This method will return a tuple that contains 2 things, one is the root of the given path and the extension of the file of the path given.
Code:
Output:
Explanation:
In the above piece of code, we have used the os module to find the file extension of the given file path. First, we get our file path, and after the splitext() function available in the os module, which returns a tuple which contains 2 values the root path and extension of the file from the file path. In path2, it doesn't contain any file, so the splitext() function will return a tuple that contains only the root of the file path, and in case of extension, it will return an empty string.
Pathlib Module
Pathlib module in python comes with the standard utility module. It contains classes representing filesystem paths for different operating systems.
Using a path string as a parameter, pathlib.Path() creates a new Path object.
Syntax:
Parameters:
-
path:
It is an object representing the file path. It can be either a string or a byte object representing a path.
Return Type:
It will create the string into the path, and assign some methods to the path like - parent - suffix - name
- parent: will return where initially the file is stored
- suffix: will tell us what is the extension of the file
- name: will return the name of the name specified in the path.
Code:
Output:
Explanation:
In the above piece of code, it uses the pathlib module to get the file extension of the given file path. First, this module creates the given string file path into the pathlib path, then the pathlib path has 3 methods which include parent, name, and suffix. The parent includes the path where the file is stored. The name includes the name of the file with its extension, and the last, suffix includes the file extension of the file in the file path.
Extracting Just Extension Suffix
Till now we have seen the path is having some pointers around which we can extract the extension of the file, but what if we don't want that pointer like ., we have seen above our file extension mostly revolve around . operator, and we have also seen from above codes that we have extracted our file extension along with . dot operator.
But, if we remove the . and extract just the extension suffix such as py, txt, Docx etc. We will be using the pathlib module for this.
Code:
Output:
Explanation:
In this piece of code, we will use both the pathlib, and os modules to get the file extension, but this time will not directly use the mentioned methods. This time we are going to suffix method to extract the file extension. In the case of pathlib, first, we extract the filepath from the pathlib function. In the return we get filepath, for that filepath we will receive a suffix method, when we call filepath.suffix it will return our filepath extension. Similarly in the case of the os module, we are simply using slicing for our extension data.
Conclusion
- In this article, we have seen how to get file extensions in Python.
- We have seen 2 libraries to get file extensions in Python OS and pathlib
- In the OS module we have used the splitext() function to get file extension in Python.
- In the pathlib module, we have seen the suffix method to get file extension in Python.