C++ getchar() Function
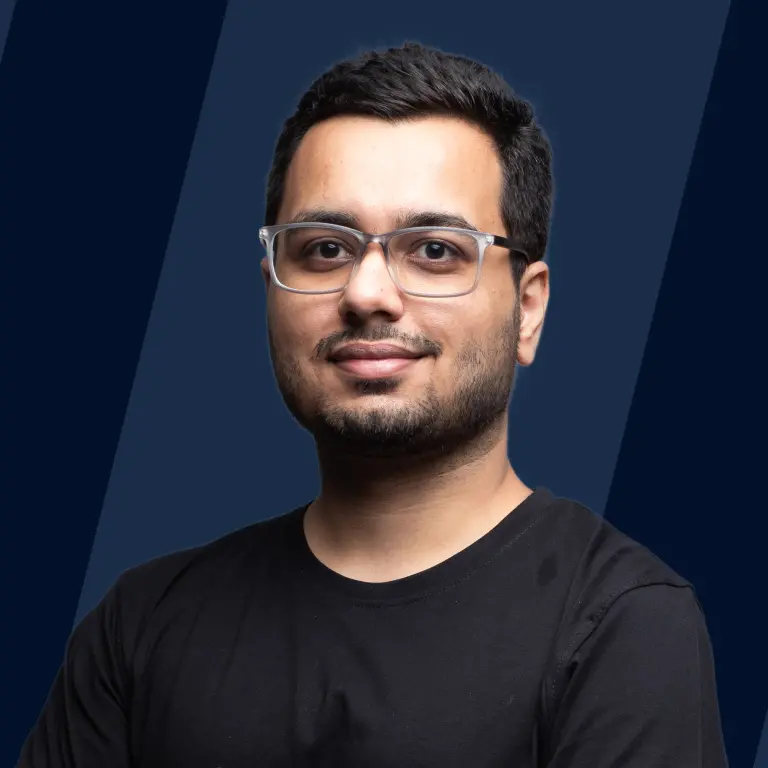
Overview
The getchar() function in C++ reads a character as input from the user. The function will stop the execution of the programming until the Enter key(\n) is pressed from the keyboard. Due to this functionality, the getchar() function is also called a blocking function.
Syntax of C++ getchar()
The syntax of getchar() function is,
The cstdio header must be included to use the getchar() function in C++ programming.
No parameters are required for the getchar() function in C++.
Return Value of C++ getchar()
The getchar() function returns the ASCII value of the entered character. If the data type to which the result from getchar() will be stored is of type char`, then the ASCII value will be converted to the corresponding character. If the data type is of type int, the values will be stored as ASCII values.
In case of failure, an EOF(End of file) is returned. If the error is caused by EOF, the EOF indicator is set for the feof() function, and if other errors cause the failure, the error indicator is set for the ferror() function through the standard input and output(stdin).
The ASCII(American Standard Code for Information Interchange) format represents characters as numbers for operating systems. We can represent 128 characters using the ASCII format, which includes smaller and upper-case alphabets, numbers, and special characters. The ASCII is currently used as a subset of the UTF-8 format, which can be used to represent more characters.
The feof() function is used to find whether the end of the file is reached while performing operations in a file or file stream.
The ferror() function is used to find if the specified file or file stream has any errors.
Exceptions of C++ getchar()
The call to the getchar() function can only be terminated by the Enter key or the \n character. Due to this behavior, we can't use a cin function next to the getchar() function because when more than one character is entered as input for the getchar() function, the character next to the first character will be stored in the buffer and will be given as input to the cin function.
The following example illustrates this,
In the above example, when more than one character is given as input for the getchar() function, the second character will be automatically taken as input for the cin function.
The output for the above example is,
An input string, "c++" is given for the getchar() function which leads to the second character,+ acting as input for the cin function, and the cout function prints the second character as output. This makes the cin function unable to use.
The getchar() function is an old function derived from the C programming language and is not the best way to get input from the user through stdin.
The getchar() function can be replaced with the getc(stdin) function, as both functions perform the same action.
How Does C++ getchar() Work?
- The getchar() function receives the character from stdin and returns either the ASCII value of the character or the character itself depending on the data type of the receiver variable. If the variable is of type int, the ASCII number of the input character is returned, and if the variable is of type char, then casting is performed internally to convert the ASCII number to the respective character and return the character or
- The getchar() function will block code execution until the Enter key is pressed.
- The first character is only stored using the function, and the character entered next to the first character and before the function ignores the Enter key.
Examples
The getchar function can be used inside a while loop to get a series of characters as input from standard input(stdin). The following example illustrates this.
In the above example, the input received from the getchar() function is stored in the character_array. It is important to note that multiple getchar() functions are used to read a series of characters. The \n character is used to represent a new line or the Enter key and marks the end of the loop and the getchar() function.
The output for the above example is,
The getchar() function can also be used to find the ASCII value of a character. The following example illustrates this,
In the above example, the variable to which the results of the getchar() function are stored is of type int. Therefore, the ASCII values of the entered characters are stored in the variable ASCII_value_of_character. The ASCII numbers can be converted to respective characters by casting to the char data type.
The condition in the while loop is similar to the previous example. The number 10 is the ASCII value of the \n character.
The output for the above example is,
We have provided four input characters and expect four lines of output, but there are surprisingly five lines of output. The last line of output is due to the \n character stored in the ASCII_value_of_character variable. This character is stored by pressing the Enter key, which produces the \n character in the last loop. The cout function on printing the new line character leads to an additional line gap. We can see the ASCII value of 10 indicating the presence of the \n character in the last line of output.
The getchar() function can be used to pause program execution until the Enter key is pressed. This property can be utilized to create a pause effect. The following code example uses the getchar() function to pause the code execution after a line is printed,
The above function utilizes the blocking property of the getchar() function and gives a pause effect in the printing of output. The second line will only be displayed in the output after pressing the Enter key.
The output will be,
Conclusion
- The getchar() function receives a character as input from the stdin.
- A getchar() function pauses code execution and is called a blocking function.
- The function can only be terminated by pressing the Enter key or the \n character.
- The function returns the ASCII values of the characters, which can be cast externally or internally to get the original character.
- A cin function can't be used consecutively next to the getchar() function.
- A string can be received as input using a series of getchar() functions.
- The getchar() function is an old function derived from the C standards, and there are many alternatives to this function.