What is Global Variable in C?
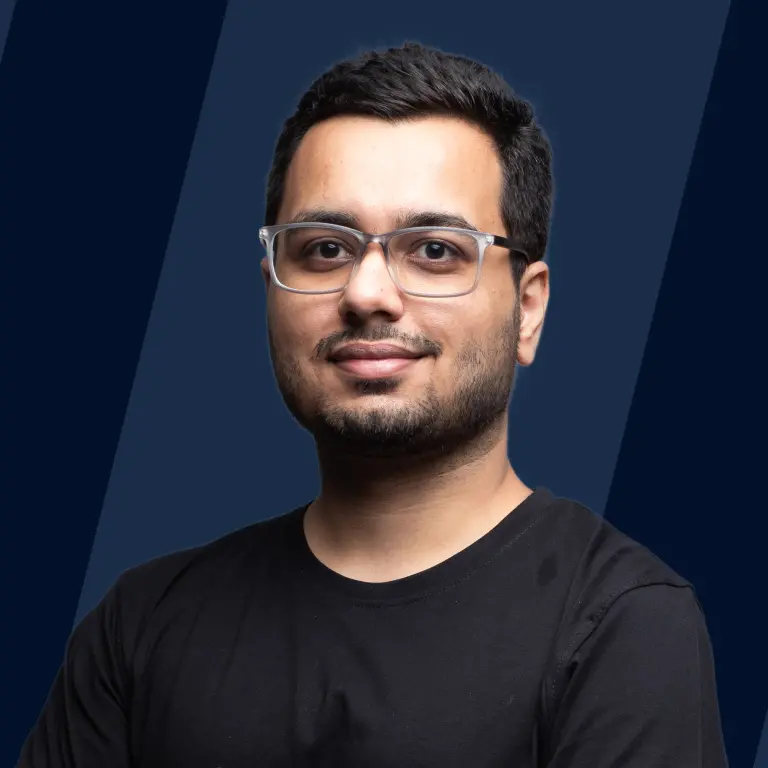
What is a Global Variable in C?
A variable defined outside the scope of all the functions is known as a global variable in C. The global variables have a global scope, hence these variables can be accessed and modified by any function, structure, or in any scope in C. Global variables are defined before the main() function. They can not be defined inside or after the main() function.
When to use Global variables in C?
Global variables can be used to make programs simpler and easy to read. However, it is usually recommended to avoid the usage of global variables. Because any function can modify the value of a global variable, global variables can cause unaccounted problems in our programs.
Some examples of the use of global variables are the implementation of singleton patterns and accessing registers in embedded systems. Global variables can also store constant values like pi in a program, or if a variable is being used by many functions, then we could declare that variable as global.
How to use Global Variables in C?
Example 1: Let us look at a simple example of global variables to understand how to use them.
Output:
In the above example, we defined a global variable var1. Because var1 was global, we could print its value using both main() and func() functions.
Example 2: Updating the value of a global variable.
Output:
In the above example, we created a global variable pi. We also created a function square() that does not take any argument as its input. However, it can change the value of pi because it is a global variable, and we could access pi in this function. In the main function, we printed the initial value of pi, i.e. 3.140000. Then, we incremented the value of pi and printed the updated value, i.e. 4.140000. Finally, we called the square() function. Because the new value of pi was 4.14, the square() function squared 4.14 and we got 17.139603 as the new value of pi.
Example 3: Redefining a global variable.
Output:
From the above example, we can observe that C does not allow us to redefine the value of a global variable. When we tried to redefine the global variable, we got a redefinition of 'var' error. However, we could change the value of global variables in functions like we did in the previous example.
Example 4: Assigning a global variable to another global variable outside a scope.
Output:
From the above example, one global variable could assign value to another global variable. This means we could not change or redefine the global variables in the global scope, but we could use global variables in the global scope.
Note: We should always initialize global variables with constant values, or the compiler automatically assigns 0 to it.
How to Access Global Variable if there is a Local Variable with Same Name in C?
We know that local variables have higher precedence (priority) than global variables. So, if there is a local variable with the same name as a global variable, we will get access to the local variable when we call the variable name.
We use the' extern' keyword to access the global variable if there is a local variable with the same name. The keyword extern stands for external or we could also use :: scope resolution operator to use global variables.
For example:
Output:
In the above example, we had a global variable and a local variable with the same name var. In the function func(), when we printed var, the value of the local var was printed.
However, when we wrote extern int var inside the new scope, we were able to print the value of the global var, and when we used :: with the variable name, we could also access the global variable.
Advantages of using Global Variables
The following are the advantages of using global variables in C:
- We can access global variables from any function or structure in a C program.
- Global variables are excellent for storing constant values like pi or e.
- Global variables are helpful when a particular variable is accessed multiple times in a program.
- A global variable needs to be declared only once and it remains in scope until the program ends.
Disadvantages of using Global Variables
The following are the disadvantages of using global variables in C:
- The value of a global variable can be changed by any function, which can result in unpredictable outputs.
- Global variables stay in memory until the program is executed. Declaring too many global variables can cause memory issues in our program.
- If global variables are removed due to code refactoring, all programs that use global variables must be updated.
Learn more about C Programming Tutorial
- Click on C Programming Tutorial to learn more about the C Programming Language.
Conclusion
- A global variable is a variable that is defined outside of all the functions.
- Global variables can be accessed and modified by any function in C.
- Global variables can only be defined before the main() function.
- We can not redefine the value of a global variable in a global scope; however, we could access a global variable in the global scope.
- If a local variable and a global variable have the same name, we can access the global variable by using the extern keyword.