Graph Algorithms
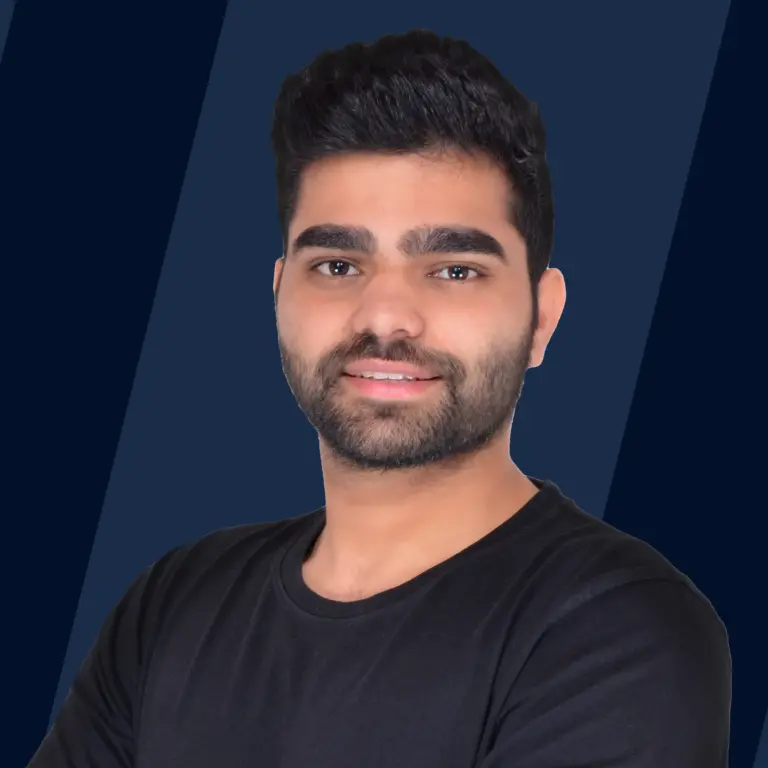
Graph algorithms are vital for analyzing interconnected data structures, enabling tasks like pathfinding and connectivity analysis in social networks and GPS systems. From route optimization to recommendation engines, they drive insights and optimizations across various domains. Their applications extend to fields like bioinformatics and logistics, showcasing their broad relevance in modern computing and problem-solving.
What is a Graph?
A graph comprises a finite collection of vertices or nodes and a series of edges linking these vertices. Vertices are considered adjacent if they share a connecting edge.
Below are some fundamental concepts related to graphs:
- Order: Denotes the total count of vertices within the graph.
- Size: Represents the total number of edges present in the graph.
- Vertex Degree: Indicates the number of edges incident to a particular vertex.
- Isolated Vertex: Refers to a vertex that lacks connections to any other vertices within the graph.
- Self-Loop: Occurs when an edge connects a vertex to itself.
- Directed Graph: A graph where edges possess directionality, indicating start and end vertices.
- Undirected Graph: Characterized by edges lacking directional attributes.
- Weighted Graph: Features edges with assigned weights.
- Unweighted Graph: Contains edges without associated weights.
Breadth First Search (BFS)
Breadth-first search (BFS) is a fundamental graph traversal algorithm used to explore nodes in a graph. It systematically visits all nodes at a certain depth before moving on to the next level. By employing a queue data structure, BFS ensures that nodes are visited in a breadthward motion, making it particularly useful for finding the shortest path in unweighted graphs.
Algorithm of BFS
- Initiate the process by appending any vertex from the graph to the rear of the queue.
- Extract the front item from the queue and include it in the list of visited nodes.
- Generate nodes for the adjacent vertices of the current node and insert those that have not yet been visited.
- Repeat steps two and three until the queue becomes empty.
Pseudocode of Breadth First Search Algorithm
Complexity: 0(V+E) where V is vertices and E is edges.
Applications of BFS
- Shortest Path Finding: Breadth-First Search (BFS) in graph algorithms is utilized to find the shortest path between two vertices in an unweighted graph.
- Network Analysis: BFS aids in traversing and analyzing networks, such as social networks or the internet, to understand connectivity patterns.
- Minimum Spanning Tree (MST): BFS is utilized to construct a Minimum Spanning Tree, ensuring the most cost-effective connection of all vertices in a graph, commonly applied in network design and optimization.
Depth First Search (DFS)
Depth-first search (DFS) is a graph traversal algorithm that explores as far as possible along each branch before backtracking. It employs a stack data structure to maintain a path and explores as deeply as possible before backtracking. DFS is often used in topological sorting, solving maze problems, and detecting cycles in graphs due to its exhaustive nature.
Algorithm of DFS
- Begin by placing a vertex from the graph at the top of the stack.
- Extract the top item from the stack and mark it as visited.
- Generate a list of adjacent nodes for the current vertex and add them to the top of the stack if they haven't been visited yet.
- Keep iterating over steps 2 and 3 until the stack has been fully emptied.
Pseudocode of Depth First Search Algorithm
Applications of DFS
- Locating any route between the graph’s vertices u and v.
- Determining how many connected elements are present in an undirected graph.
- Sorting a given directed graph topologically.
- Finding components with strong connections in the directed graph.
- In the given graph to find cycles or to determine whether the provided graph is bipartite.
Dijkstra's Shortest Path Algorithm
Dijkstra's algorithm is a graph search algorithm used to find the shortest path between nodes in a weighted graph. It operates by iteratively selecting the node with the shortest distance from a source node and updating the distances to its neighboring nodes accordingly. Dijkstra's algorithm is widely used in various applications such as routing protocols in computer networks and navigation systems in transportation.
Algorithm of Dijkstra’s
- Initialize all vertices to infinity, except the source vertex.
- Push the source vertex into a priority queue in the format (distance, vertex), setting its distance to 0.
- Extract the vertex with the minimum distance from the priority queue.
- Update distances after extracting the minimum distant vertex by considering the condition (current vertex distance + edge weight < next vertex distance).
- If a visited vertex is extracted, proceed to the next iteration without utilizing it.
- Continue steps 3 to 5 until the priority queue becomes empty.
Pseudocode of Dijkstra’s Algorithm
Applications of Dijkstra's Algorithm
- Navigation Apps: Dijkstra's algorithm powers mapping services like Google Maps, offering optimal travel routes between locations.
- Networking: Essential for determining minimum-delay paths in computer networks and telecommunications.
- Game Strategies: Utilized in game-playing algorithms to make strategic moves and reach specific objectives efficiently.
- Route Optimization: Key in logistics for optimizing delivery routes, public transport systems, and supply chain management.
Cycle Detection
In the context of graph algorithms, a cycle is defined as a path that both starts and ends at the same vertex. This means that if you follow a path from a vertex and return to the starting point, you've encountered a cycle. Cycle detection involves identifying such loops or chains that include all the nodes visited during traversal. Hence, the essence of cycle detection lies in recognizing and handling these recurring patterns within the graph structure.
Pseudocode of Cycle Detection Algorithm
Applications of Cycle Detection
Cyclic algorithms are utilized in:
- Message-Based Distributed Systems: Facilitating routing and fault tolerance.
- Large-Scale Cluster Processing Systems: Optimizing data processing and resource allocation.
- Deadlock Detection: Ensuring smooth operation in concurrent systems.
- Cryptographic Applications: Managing encrypted messages and keys for secure communication.
Minimum Spanning Tree
A minimum spanning tree (MST) is a subset of edges in a graph that connects all vertices without forming cycles and minimizes the total edge weight. It ensures optimal connectivity while minimizing the sum of edge weights. Multiple MSTs can exist depending on edge weights and connectivity criteria.
Pseudocode of Minimum Spanning Tree
Applications of Minimum Spanning Tree
- Network Design: MST is crucial for designing efficient network connections in graph algorithms.
- Traveling Salesman Problems: MST plays a role in optimizing routes for the traveling salesman problem.
- Minimum-Cost Weighted Perfect Matching: Useful for finding the minimum-cost matching in graph theory.
- Multi-Terminal Minimum Cut Problems: Applied to solve problems related to cutting a graph into subgraphs.
- Image and Handwriting Recognition: MST aids in analyzing patterns and structures in images and handwriting.
- Cluster Analysis: MST assists in grouping data points into clusters for analysis and interpretation.
Topological Sorting
Topological sorting is a method used to arrange the vertices of a directed graph in a linear order. This ensures that for every directed edge from vertex A to vertex B, A precedes B in the ordering. This ordering aids in understanding dependencies and sequences in the graph structure.
Pseudocode of Topological Sorting
Applications of Topological Sorting
- Kahn's Algorithm: Utilizes topological sorting to efficiently sort vertices by processing nodes with no incoming edges first.
- DFS Algorithm: Applies Depth-First Search to explore and order vertices, ensuring dependencies are satisfied in the resulting sequence.
- Scheduling: Utilized in task or instruction scheduling to ensure that dependent tasks are executed in the appropriate sequence.
- Dependency Resolution: Valuable in resolving dependencies in software development, determining the order in which components should be compiled or linked to fulfill dependencies accurately.
Graph Coloring
The task of "coloring" a graph's components entails doing so while adhering to a number of limitations and constraints. In other terms, "graph coloring" is the act of giving vertices colors so that no two neighboring vertexes have the same hue.
Chromatic Number: The chromatic number of a graph G represents the minimum number of colors needed to properly color it.
Pseudocode of Graph Coloring
Applications Graph Coloring
The idea of graph coloring is used in the creation of schedules, Suduku, registration allocation, and the coloring of maps.
Strongly Connected Components
Strongly connected components (SCCs) are subsets of vertices in a directed graph where each vertex is reachable from every other vertex within the subset. Identifying SCCs is essential for understanding the connectivity and structure of directed graphs, aiding in various applications such as network analysis and software engineering.
Pseudocode of Strongly Connected Components
Applications of Strongly Connected Components
- Network Analysis: SCCs help in understanding the connectivity and flow within directed networks, crucial for optimizing communication and resource allocation.
- Software Engineering: Used in analyzing dependencies between modules or components, aiding in code refactoring and system design.
- Routing Algorithms: SCCs play a role in designing efficient routing algorithms for directed networks, ensuring optimal data transmission paths.
- Database Management: Utilized in database systems for query optimization and transaction management, improving performance and data integrity.
Maximum Flow
When used as a problem-solving method, the maximum flow algorithm often models the graph after a network flow architecture. As a result, the method for calculating the maximum flow is to identify the flow channel with the highest flow rate. The maximum flow rate is calculated using augmenting routes, where the flow in the sink node equals the total flow based out of the source node.
Pseudocode of Maximum Flow Algorithm
Applications of Maximum Flow Algorithm
- Maximum Flow Algorithms: Ford-Fulkerson, Edmonds-Karp, and Dinic's algorithms are used to solve the maximum flow problem efficiently.
- Flight Crew Scheduling: Helps optimize crew assignments for flights, ensuring efficient coverage and resource allocation.
- Image Segmentation: Utilized in image processing to distinguish foreground and background regions by analyzing flow between pixels or regions.
- Sports Strategy: Applied in sports like basketball to strategize and maximize scores, aiding teams in achieving competitive advantage.
Matching
The edges of a matching algorithm or approach in a graph are those that share absolutely no vertices. When the greatest number of edges and the greatest number of vertices match, the matching is said to be maximum matching. It employs a particular methodology to identify entire matches, as seen in the graphic below.
Pseudocode of Matching Algorithm
Application of Matching Algorithm
- Blossom and Hopcroft-Karp Algorithms: Efficiently solve matching problems in graphs.
- Hungarian Method: Resolves optimization problems using matching principles.
- Stable Marriage Problem: Matches couples based on preferences, ensuring stability.
- Resource Allocation: Allocates resources efficiently, optimizing utilization.
Explore Scaler Topics Data Structures Tutorial and enhance your DSA skills with Reading Tracks and Challenges.
Conclusion
- Vital for Analysis: Graph algorithms are crucial for analyzing interconnected data structures, enabling tasks like pathfinding and connectivity analysis.
- Broad Applications: From route optimization to recommendation engines, they drive insights and optimizations across various domains.
- Relevant in Modern Computing: The relevance of graph algorithms extends to fields like bioinformatics and logistics, showcasing their importance in modern computing.
- Efficient Solutions: Graph Algorithms like BFS, DFS, Dijkstra's, and MST offer efficient solutions for various graph-related problems.
- Optimization and Efficiency: They optimize network design, routing, and resource allocation, enhancing system efficiency.
- Versatile Applications: Used in navigation apps, network analysis, game strategies, and scheduling, highlighting their versatility.