What is Has-A-Relation in Java?
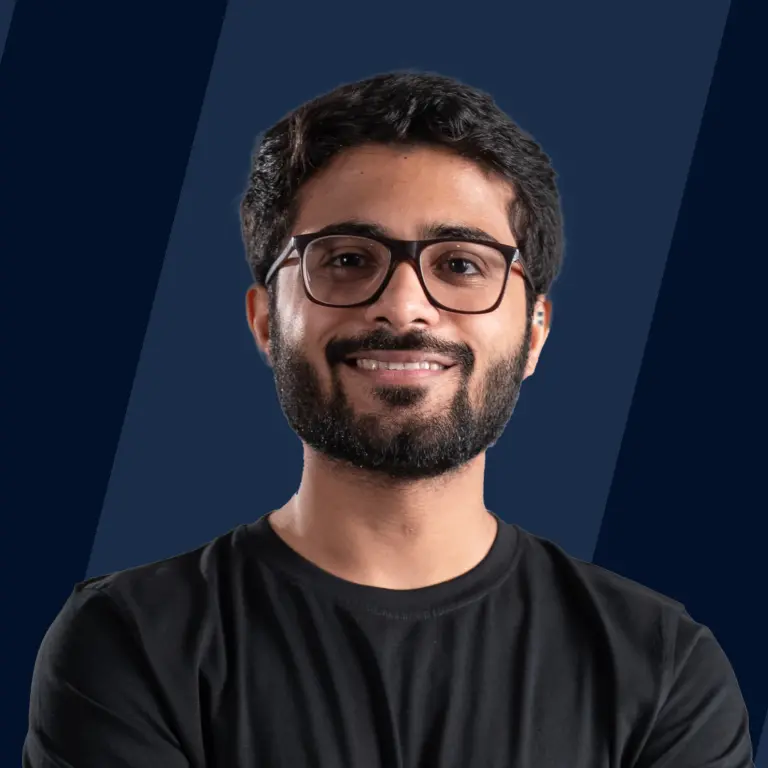
Suppose we declare two different classes in Java. Between those two classes, there can be two types of relationships. This relationship can be either an Is-A relationship or a Has-A relationship. Is-A relationship is achieved by inheritance, and Has-A-relationship can be achieved using composition. Composition is a term where we declare the object of one class as a data member inside another class. In simple words, a relationship where an object has a reference to another instance of the same class or has a reference to an object of another class is called composition or has-a-relation in Java.
To create has-a-relation we need a minimum of two classes, where the instance variable of one class is declared in another class.
Let us see an example in layman's terms that will help you in understanding the above definition.
Examples of Has-A-Relationship
Consider a lady; she can be called a mother only when she has a child. And a child cannot exist without their mother. We can understand the above example by the following diagram.
In the above diagram, we can see that the mother class is derived from the lady class. So this type of relationship is called an Is-a relationship. But our main focus is on the Has-a relationship that exists between mother and child. These two classes are tightly coupled, which means that the existence of one class depends on another class. If the child does not exist, then a lady cannot be called a mother and vice versa.
Implementation
Following is the code for displaying the Is-a relationship. We will be taking the above-discussed example where we will be showing a Has-a relationship between mother and child.
Code
Output
Explanation
As we have discussed, the definition of the has-a relation is where an object has a reference to an object of another class. In the above example, we have a reference of the mother class in the child class. Thus a has-a relationship is established by declaring the instance of the mother class inside the child class.
Comparing Composition and Inheritance: Relationship
- It is easier to change the class in composition than in inheritance. As if we change the properties in the parent class, then all the child class that is inherited from the parent class gets affected by it, but in the case of the has-a relationship changing the properties of one class does not affect another class.
- Static binding is inheritance, while dynamic binding is composition. In static binding, the type of the object is determined at compile-time, whereas in dynamic binding, the type of the object is determined at run time.
- The inheritance of classes is defined at compile-time, whereas object composition is defined at run time.
- Internal details are not supposed to be exposed to each other in object composition, and they interact through their public interfaces, whereas Inheritance exposes both the public and protected members of the base class.
- Access can be restricted in composition, but there is no access control in object composition. It means that in composition, a class can have only some properties from another class, but in the case of inheritance, all the properties (except the private ones) of a parent class can be used in the child class.
- Inheritance violates encapsulation by exposing the details of its parent's implementation to a subclass, whereas Object Composition does not violate encapsulation because objects are accessed entirely through their interfaces.
How to Decide which Type of Relation We Need
Defining relations between objects is one of the most important aspects in the world of object-oriented programming. So, let us see how to decide the relation between objects.
Suppose there is a Jeep and its name is "Mahindra Thar". Then we say that "Mahindra Thar is a Jeep". "Mahindra Thar has a jeep" this sentence does not make any sense. The relationship totally depends upon the phrase we used.
"Mahindra Thar" has an Engine, or "Mahindra Thar" has an AC makes sense rather than "Mahindra Thar" is an engine or "Mahindra Thar" is an AC.
So, if your problem with a phrase containing ".... is a …." words, you should you Is-a a relationship or else use Has-a relationship.
Types of Has-A-Relationship
There are two types of has-a relationships. They are as follows:
- Aggregation
- Composition
Both aggregation and composition are subsets of association. In Java, an association is a connection or relation established between two distinct classes via their objects. The following diagram shows the relationship between association, aggregation, and composition.
Aggregation
Aggregation is a fundamental concept in object-oriented programming. It focuses on the development of a Has-A relationship between two classes. In other words, two aggregated object have their own life cycle, but one of them has a Has-A relationship owner, and a child object cannot belong to another parent object. Also, we can say that aggregation is a one-way relationship between classes. The aggregation has weak bonding between classes.
For example, the library has students. It means that students can still study if there is no library, but if the student does not exist, then the library will be of no use.
Composition
Composition is a more limited version of aggregation. Composition is defined as when one class that includes another class is so dependent on it that it cannot function without the class that is included. For instance, a car cannot exist without its engine. In the same way, the engine won't work for other cars (which means the engine will be useless if a car does not exist). So engine and car are two classes that have a composition relationship.
As a result, the term composition refers to the items that something is made of, and changing the composition of things causes them to change. The composition has strong bonding between classes.
Conclusion
- Association has two types of relationship, i.e., aggregation and composition.
- Aggregation is weak bonding between classes and composition is strong between classes. Also, aggregation is a one-way relationship between classes.
- Inheritance is a tight coupling between classes, and association is a loose coupling between classes.
- Any class having an instance variable that is referring to another class is considered as has-a-relationship.
- Has-a-relation helps in code reusability and also minimizes the duplication of the code.