hash() in Python
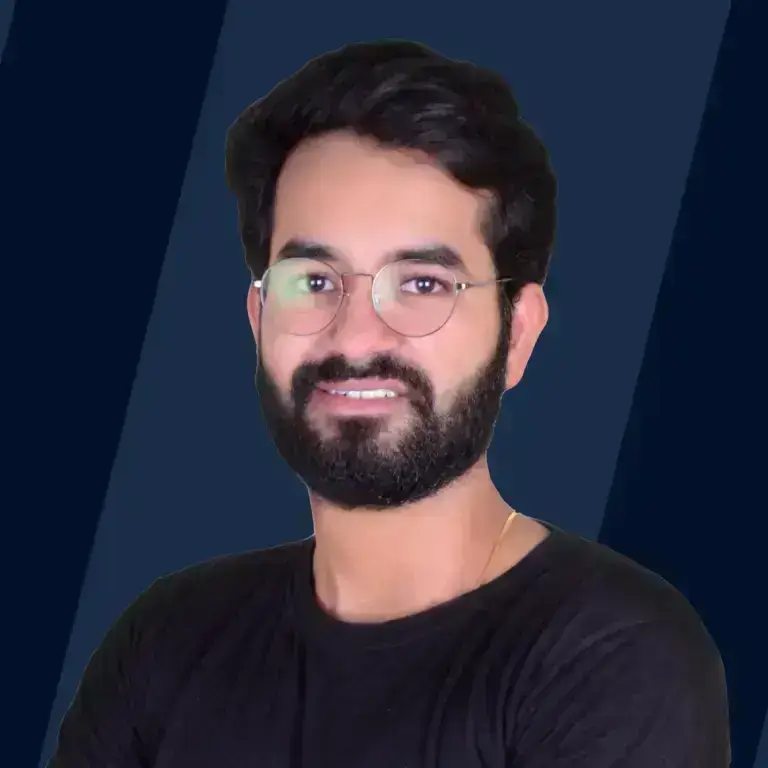
Overview
hash() function in python is used to return a hashed integer value of the object we pass as a parameter into it iff the object is hashable. Generally, the hash values are used to compare the dictionary keys while doing a dictionary lookup.
Syntax of hash() function in Python
Syntax for using the hash() function is as follows:
Parameters of hash() function in Python
hash() function takes one parameter as an input:
- object: an object whose hash value is to be returned. An object can be an integer, string, list, tuple, etc.
Return Values of hash() function in Python
Return Type: <class 'int'>
- hash() function returns an integer value which is the hash of the object passed into the function.
Example of hash() function in Python
Code:
Output:
What is hash() function in Python?
hash() function is used in Python to obtain the object's hash value. The object can be of integer, string, list, tuple type. The integer value is returned from the hash function, which is the hashed value of the object. The hashed values are generally used for the faster comparison between the two objects as the hash values are directly compared rather than comparing each object's value.
How does hash() work on custom objects?
_hash_() method is called internally while we use the hash() function in python. So in order to override the _hash_() method we need to have our own custom definition of _eq_() and _hash_() functions. Below is the example where we can see how we can use the hash() function on custom objects.
Code:
Output:
More Examples
Example 1: Using hash() with integer, string and float
Code:
Output:
Explanation:
As we said, applying the hash() function on integer, string, and float values will fetch the integer value as output, which are the hash values of the original inputs.
Example 2: Using hash() with immutable tuple objects
Code:
Output:
Explanation:
On applying the hash() function on an immutable object, we get the integer value which is the hash value of the entire set.
Example 3: Using hash() with the mutable object
Code:
Output:
Explanation: On applying the hash() function on mutable objects an error is raised saying unhashable type as the list is a mutable object which cannot be hashed using the hash function.
Conclusion
- hash() function is used to fetch the hash values of the python objects.
- hash() function returns the integer value if the hashable object passed in the function as a parameter.
- hash() function calls inbuilt __hash__() function which can be overriden for hashing the custom objects.
- hash() function can be applied to immutable objects.
- hash() function cannot be applied to mutable objects.