Hashmap vs ConcurrentHashMap in Java
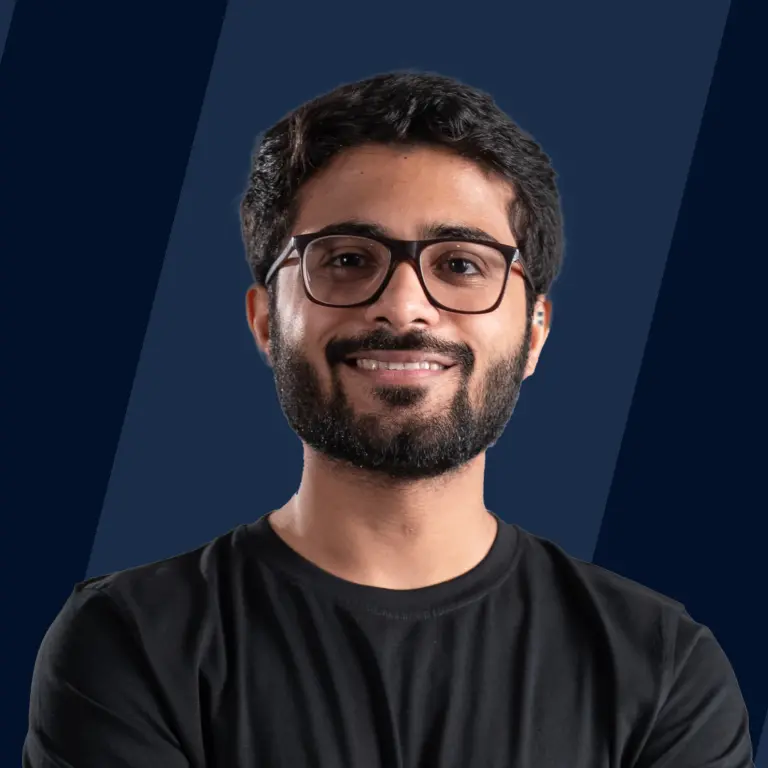
Overview
HashMap is non-synchronized and not thread-safe, offering high performance but potentially leading to ConcurrentModificationExceptions during concurrent iterations. On the flip side, ConcurrentHashMap ensures thread safety to prevent exceptions caused by concurrent modifications, although it does come with a slight performance trade-off due to synchronization. In this article, we will delve into their distinctions.
HashMap in Java
You must've encountered a data structure called HashMap during your time in Java programming. It's like a valuable tool in the Java toolbox, and you can find it neatly packed away in the java.util package.
What does it do?
Well, it's Java's way of letting you create a kind of data storage system where each piece of data has a unique key. Imagine it as a virtual treasure chest where every key leads to a specific piece of information, and that information can be of any type.
Now, here's the catch: those keys must be unique because they're like the secret codes to open the treasure chest. In Java lingo, we call it HashMap<K, V>, where 'K' stands for Key and 'V' stands for Value.
Syntax:
The declaration for java.util.HashMap class is:
The HashMap in Java implements Serializable, Cloneable, Map<K,V> interfaces and it extends AbstractMap<K,V> class.
Parameters:
The HashMap in Java takes two parameters which are as follows:
- K: Data type of unique keys maintained by the map.
- V: Data type of values maintained. Contrary to keys these values need not be unique. It can be duplicate.
Learn more about Hashmap in Java here: HashMap in Java
ConcurrentHashMap in Java
ConcurrentHashMap, residing in the java.util.concurrent package, stands out in the world of Java collections by enhancing performance through a distinct locking approach when compared to Hashtable or Synchronized HashMap. It's like the expert multitasker of maps, ensuring thread safety and enabling multiple threads to collaborate on a single map without causing chaos. When you create one, you have the flexibility to set parameters like its initial capacity, concurrency level, and load factor to fine-tune its behavior.
This class doesn't just stop at being thread-safe; it offers a rich set of methods for secure element insertion and removal, along with powerful bulk operations like foreach(), search(), and reduce(). Plus, it's well-prepared for data transport as it implements the Serializable interface and aligns with the ConcurrentMap standard. In a nutshell, ConcurrentHashMap is your go-to tool for efficient and safe concurrent data management in Java.
Syntax:
ConcurrentHashMap derives from AbstractMap, indicating that AbstractMap is the parent or superclass, while ConcurrentHashMap is the child or subclass. This inheritance relationship implies that all the methods available in AbstractMap can also be employed in ConcurrentHashMap. Additionally, ConcurrentHashMap implements both the ConcurrentMap and Serializable interfaces, making it well-equipped for concurrent data management and serialization.
Know more about ConcurrentHashMap in Java here: ConcurrentHashMap
Examples of HashMap and ConcurrentHashMap
Let's look at some of the examples of HashMap and ConcurrentHashMap to understand it better
Example 1: HashMap
Output
Example 1: ConcurrentHashMap
Output
Within a HashMap, both keys and values can be set to null without issue. However, in a ConcurrentHashMap, assigning null values to either keys or values will result in a Run-time exception called NullPointerException.
Let's see how to implement this concept using both HashMap and ConcurrentHashMap
Example 2: HashMap
Output:
Example 2: ConcurrentHashMap
Output:
HashMap vs ConcurrentHashMap
Parameters | HashMap | ConcurrentHashMap |
---|---|---|
Performance | Comparatively faster in performance than the ConcurrentHashMap. | Comparatively much slower in performance as compared to the HashMap. |
Thread Safe | Not at all thread-safe. | Always remains thread-safe |
Synchronization | It does not stay synchronized because it is not thread-safe in nature. | It stays synchronized because it is thread-safe in nature |
Null Values | Keys and values can be null. | A NullPointerException is thrown if null values or keys are present. |
Type of Iterator | The HashMap iterator operates in a fail-fast manner. If a concurrent modification occurs during iteration, an exception called ConcurrentModificationException is thrown by the ArrayList. | It's essentially fail-safe. Consequently, during iteration, a ConcurrentHashMap never throws such exceptions. |
Occurrence since Java version | 1.2 | 1.5 |
FAQs
Q. Why do keys need to be unique in a HashMap?
A. Keys in a HashMap serve as unique identifiers to retrieve corresponding values. They must be unique because they act like secret codes to access specific information stored on the map.
Q. What are some practical applications of HashMap in Java?
A. HashMaps are versatile and find use in various scenarios, including caching, data indexing, and implementing associative arrays. They are especially handy when you need to quickly look up data based on unique keys.
Q. Are there any performance considerations when using ConcurrentHashMap?
A. Yes, while ConcurrentHashMap offers excellent concurrency support, it's essential to choose appropriate initial capacity, concurrency level, and load factor values to match one's application's needs.
Q. Can I use ConcurrentHashMap in place of synchronized blocks for thread safety?
A. ConcurrentHashMap is a preferred choice over synchronized blocks for concurrent data access since it offers better performance and scalability and simplifies thread-safe operations, making your code more efficient and readable in multi-threaded scenarios.
Conclusion
- In HashMap, null values are allowed for key and values, whereas in ConcurrentHashMap null value is not allowed for key and value, otherwise, we will get a Run-time exception saying NullPointerException.
- All the methods available in AbstractMap can also be employed in ConcurrentHashMap
- ConcurrentHashMap is a preferred choice over synchronized blocks for concurrent data access because it offers better performance and scalability
- HashMap is introduced in JDK 1.2 whereas ConcurrentHashMap is introduced by SUN Microsystem in JDK 1.5.
- Keys in a HashMap serve as unique identifiers to retrieve corresponding values. They must be unique because they act like secret codes to access specific information stored on the map.