Header files in C
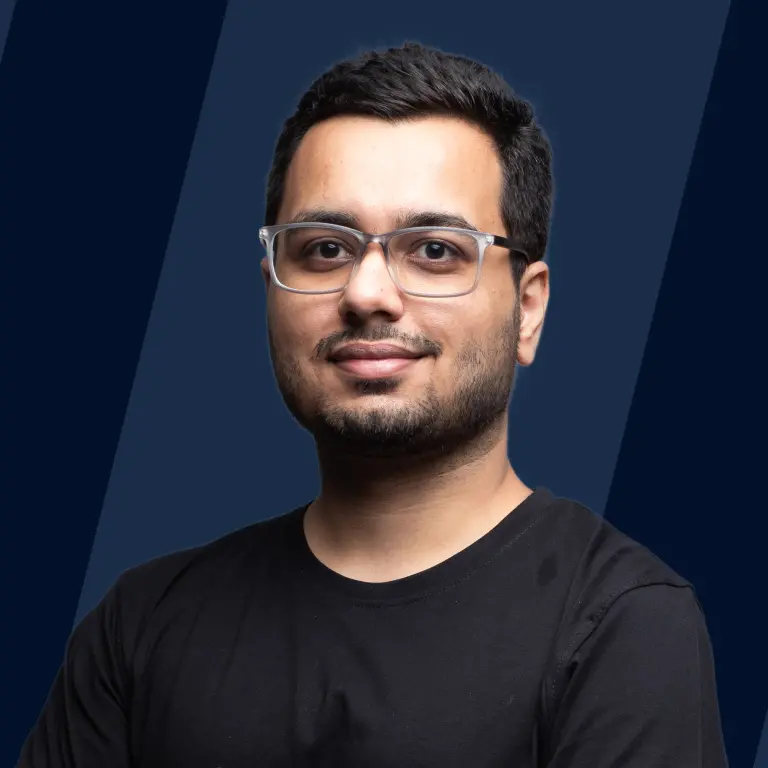
What are those mysterious include statements at the beginning of most C programs? What are header files in C, and why do we need them? Header files contain a set of predefined standard library functions. The .h extension is used for header files in C, and we include a header file in our program using the C preprocessing directive #include.
Syntax of Header Files in C
We have discussed, What is header file in C. Now, we will discuss the syntax of including header files in C, which is straightforward and follows a common convention. Here's the syntax:
In this syntax:
- #include is the preprocessor directive used to include external files in the program.
- < and > are angle brackets that indicate that the header file is part of the system's standard library or located in a directory specified by the compiler's search path.
- header_file_name.h is the name of the header file to be included in the program. The .h extension denotes that it's a header file.
Alternatively, if the header file is located in the current directory or any directory which the user specifies, the syntax would be:
In this case:
- Double quotes " instead of angle brackets, which indicates that the header file is located in the current directory or any specified directory.
- The rest of the syntax remains the same as in the previous example.
Including C header files allows the program to access declarations, macros, functions, and other components defined in those files, enabling modularization and code reuse in C programming.
Example of Header File in C
The below example explains the use of header file in C.
Output
Types of Header Files
C Header files are of 2 types:
Standard Header Files in C
These get installed when the C compiler is installed. If you try to import a system header file, the C compiler will go through the standard files using the set path. System header files are there to ‘inform’ the compiler that standard functions like printf, scanf, and malloc exist.
Standard Header File | Uses |
---|---|
<stdio.h> | Input and output operations such as printf, scanf |
<stdlib.h> | Memory allocation, process control functions |
<string.h> | String manipulation functions |
<math.h> | Mathematical functions |
<time.h> | Time and date functions |
<stdbool.h> | Boolean data type and values |
Example:
Non-Standard Header Files in C
Non-Standard Header File | Uses |
---|---|
<conio.h> | Console input/output functions (non-standard, primarily for MS-DOS) |
<graphics.h> | Graphics functions (non-standard, primarily for MS-DOS) |
<windows.h> | Windows API functions (non-standard, for Windows programming) |
<unistd.h> | UNIX system calls (non-standard, for UNIX-like systems) |
<process.h> | Process control functions (non-standard, for some systems) |
Example:
Create Your Header Files in C
Creating a header file in C involves defining declarations, macros, and other components you want to make available in other C source files. Here's a simple example of creating a header file named myheader.h:
-
Create a Header File (e.g., myheader.h):
-
Include the Header File in Your Source Code:
-
Compile and Run Your Program:
Compile the program using a C compiler:
gcc myprogram.c -o myprogram
Run the executable:
./myprogram
This lets you share joint declarations and macros across multiple C source files, promoting modularity and code reusability.
How to Include Multiple Header Files
Including multiple header files in a C program can be managed efficiently using conditional preprocessor directives to avoid duplicate inclusion. Here's how you can do it:
In this example:
- Each header file inclusion is guarded by preprocessor directives (#ifndef and #endif).
- The macro name (MY_HEADER_FILE_1, MY_HEADER_FILE_2, MY_HEADER_FILE_3) is unique for each header file.
- If, let's say, a header file is included multiple times, the preprocessor checks whether the corresponding macro is defined. If it's not defined, it includes the header file and defines the macro to prevent re-inclusion.
- If the macro is already defined, it skips the inclusion of the header file, avoiding duplication.
Conclusion
- The syntax for including header files using the #include directive.
- There are two types of header files in C: standard header files and non-standard header files.
- Creating custom header files enables programmers to encapsulate declarations, macros, and other components.
- Conditional preprocessor directives such as #ifndef, #define, and #endif are used to prevent multiple inclusions of header files.