How Can a Call to Overloaded Function Be Ambiguous?
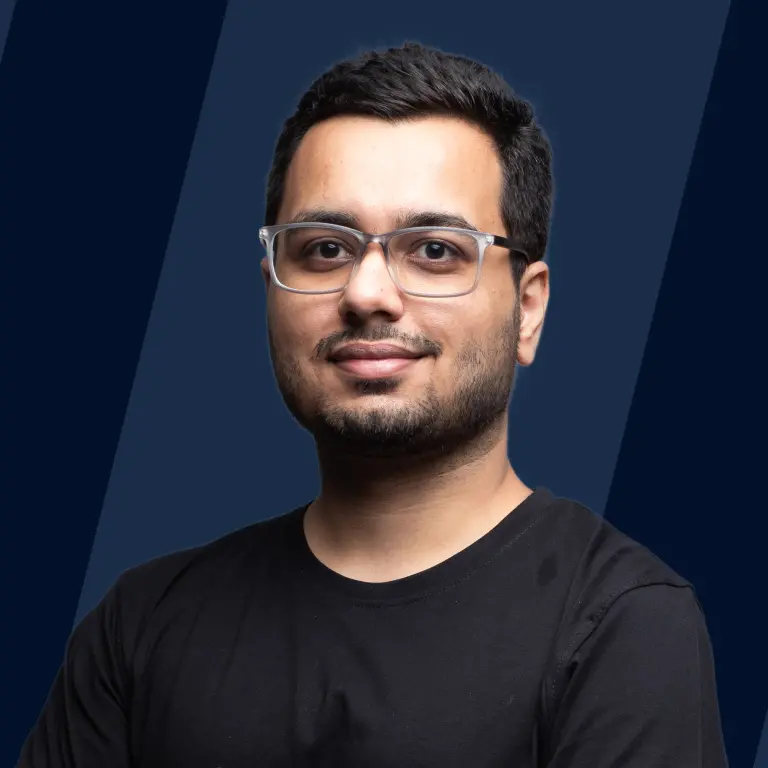
Function overloading is a paramount feature of Object Oriented Programming in C++. A function in C++ can be called overloaded when it has two or more definitions within a program that either accepts different numbers of arguments or different types of arguments. The conditions for two or more functions to be overloaded in C++ are:
- The functions need to have the same declaration and a different number of arguments.
- The functions need to have the same declaration and different order of arguments.
- The functions need to have the same declaration and different types of arguments.
Now, before we head towards discussing how can a call to an overloaded function be ambiguous, let's learn What are ambiguous statements in function overloading?
- Ambiguous statements are error-generating; the programs containing ambiguity will not compile.
So the question is: How can a call to an overloaded function be ambiguous?
The primary reason behind the ambiguity during a function call of an overloaded function in C++ is automatic type conversion. When a function call cannot find the function definition with the same parameter type, it converts it. This sometimes creates a situation where the function cannot decide which function to call, thus causing ambiguity.
Note: The above processes are detailed in further article sections.
When a Function is Said to Be Overloaded?
Function overloading is a feature of object-oriented programming where two or more functions can have the same name but different arguments.
A function in C++ is said to be overloaded when the declaration or the name of a function is overloaded with different kinds of jobs based on the arguments it is passed.
A function in C++ is overloaded when it has two or more definitions within the function with the same name but:
- Different numbers of arguments.
- Different types of arguments.
Whenever we call an overloaded function in C++, it decides which function definition to reach out to based on the arguments passed. The following is the pictorial representation of function call of overloaded functions in C++:
Examples of Overloaded Functions
In this section, we will go through some examples of overloaded functions in C++:
Overloading the Type of Argument
In this example, we will overload the divide function such that it will call different function declarations for different types of arguments passed to it.
Code:
Output:
Explanation of the Example:
In the above example, we have defined two functions with the same name, divide. The first divide function takes two arguments of type int, while the second one takes two arguments of type double. Since both functions have the same declaration and different types of arguments thus, the function divide is overloaded.
Now, when divide(10, 5) is called, since its arguments are both of integer type thus, it will call the first divide function with both integer type arguments, whereas when divide(10.0, 3.0) is called since its arguments are both of double type thus it will call the second divide function with both double type arguments.
Overloading the Number of Arguments
In this example, we will overload the displayName function such that it will call different function declarations for different numbers of arguments passed to it.
Output:
Explanation of the example:
In the above example, we have defined two functions with the same name, displayName. The first displayName function takes one argument of type string, while the second displayName function takes two arguments of type string. Since both functions have the same declaration and a different number of arguments thus, the function displayName is overloaded.
Now, when displayName("Alice") is called, since it has only one argument of string type, thus it will call the first displayName function, whereas when displayName("Peter", "Parker") is called since it has two arguments of string type thus it will call the second displayName.
What Do We Mean by the Ambiguity of an Overloaded Function?
As discussed earlier, the ambiguity of an overloaded function is when the program encounters a situation such that the compiler cannot choose which function among the overloaded function it should choose upon the function call.
How can a call to an overloaded function be ambiguous?
As discussed earlier, the answer to how can a call to an overloaded function be ambiguous is automatic type conversion in C++.
Suppose in the example discussed in the When a function is said to be overloaded? We are calling display('a'). Here the type of the argument passed is char. Since there is no definition on display available with a single char thus, the argument will be automatically converted into int type, and display(int x) will be called.
The above call happened because, in C++, the char type can be automatically type converted into the int type.
Now let's consider the following situation:
Now, suppose we are calling display('a'). Since the display function with either char or int is not present thus, the display('a') function will call either display(float x) or display(double x). Both double and float are equally valid conversions for char in C++; thus, the compiler cannot prioritize one function declaration over another. Thus, in this case, call to an overloaded function will be ambiguous.
Example of Ambiguity of an Overloaded Function
In this section, we will discuss examples of how can a call to an overloaded function be ambiguous.
Example 1: Function Being Ambiguous Due to Type of the Argument Passed
In this example, we will see how passing a float value as an argument can cause ambiguity.
Code:
Output:
How did the Ambiguity Occur?
In the above example, we have defined two display functions that take arguments as a single int value and a single long value, respectively.
Now, when the display(3.3f) above code will throw an error because the display(3.3f) function call will look for the float function. If not present, it is only promoted to double, but there is no function definition with a double or float parameter type.
Unless explicitly specified, all floating-point literals are automatically of type double in C++. In this ambiguity, the float type variable is implicitly converted to a double type. If there is no overloaded function of float or double type and the function is called with a float value, then the program will throw an error.
Example 2: Function being Ambiguous due to the Number of Arguments Passed
Output:
How did the Ambiguity Occur?
In the above example, we have overloaded the sum function. Thus when sum(100) is called in the main function, the compiler gets confused about whether to call the sum(int x) with one argument or to call sum(int x, int y = 0) with two arguments and one default argument. This causes ambiguity.
How to Remove the Ambiguity of an Overloaded Function?
Now that we have learned how can a call to an overloaded function be ambiguous, we will learn how to remove the ambiguity of an overloaded function in this section.
The following are the ways to remove ambiguity from a function:
- By typecasting the argument: If the ambiguity of an overloaded function has occurred due to the type of the argument, then we can typecast the argument passed to either one of the defined functions.
- By removing ambiguity-generating function: We can remove ambiguity by removing the functions that create ambiguity.
- By altering the arguments: We can change the arguments of overloaded functions such that ambiguity doesn't occur.
Let's apply the above methods and remove the ambiguity from the examples discussed in the above sections:
Example 1:
- Removing ambiguity by typecasting the argument:
Code:
Output:
Explanation of the Example:
In the above example, we have type-casted the float value 3.3f to an integer. Thus when display((int)3.3f) is called, the compiler will call the display(int x); thus, the ambiguity gets removed.
- By removing ambiguity generating function
Code:
Output:
Explanation of the Example:
In the above example, we have removed the display function with long as an argument and added a display function with double as an argument. Thus when display(3.3f) is called, the compiler will call the display(double x); thus, the ambiguity gets removed.
Example 2:
- By altering the argument
Output:
Explanation of the example:
In the above example, we have removed the default y = 0 from the second sum function. Thus, when sum(100) is called, it will call the sum function that takes one argument. Thus the ambiguity is resolved.
Learn More about the Concepts of C++ in Detail
- To learn more about C++ concepts in detail please visit this link.
Conclusion
- A function is overloaded when it has two or more definitions that accept different numbers or types of arguments.
- Ambiguous statements are error-generating; the programs containing ambiguity will not compile.
- A call to an overloaded function be ambiguous due to automatic type conversion.
- Ambiguity can be removed by typecasting the argument, removing the ambiguity function, or altering arguments.