How Does One Convert Date Object to a String?
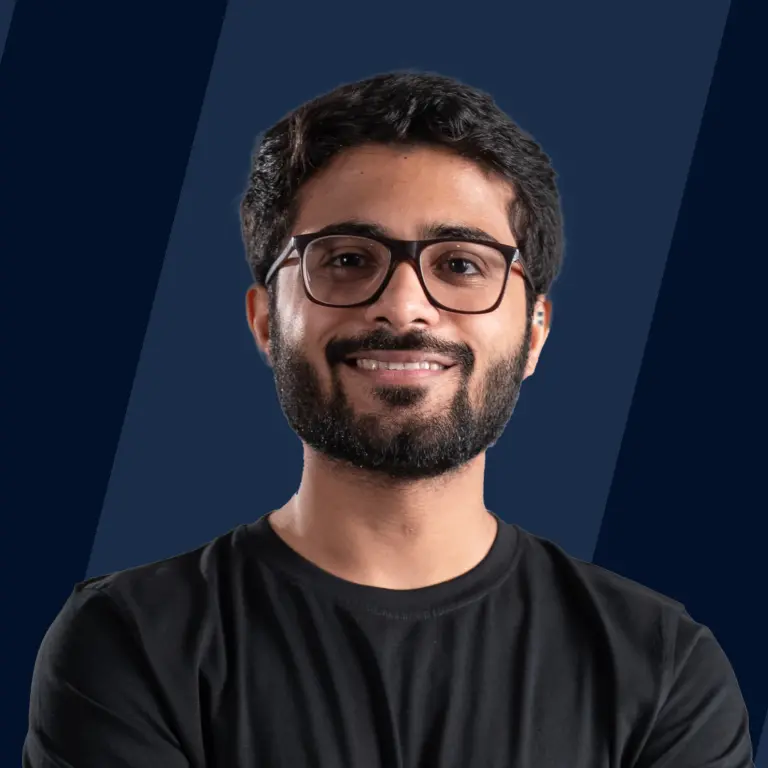
Overview
Converting a date format of a given date to a string is one of the everyday programming tasks
This article will teach about DateFormat classes like SimpleDateFormat and the java.time.format.DateTimeFormatter from the new Date and Time API in JDK 8.
How to Convert Date to String in Java ?
In the function String_to_date, which as the name suggests helps to convert date objects to string, we use a dd/MM/yyyy format, the format can be changed by modifying the pattern in SimpleDateFormat
Let’s now see an implementation of Date object to String conversion where the current date is taken as an input and converted into a string. There are two formats in which we can get the output string SimpleDateFormat.
Output :
Method - 1 : Using the SimpleDateFormat Class
The SimpleDateFormat class can be used to change the date format for cases where we’re not running JDK 8 and are consecutively supposed to stick with an old date and calendar API
-
To create a date format with an old pattern :
-
To convert String to date using the old format :
-
To create a DateFormat with the new pattern :
-
To convert the Date object to String using Dateformatter of the new pattern :
We now have a String in the needed format. We need to be careful with the pattern we specify since the letters are case-sensitive where yyyy-mm-dd is not the same as yyyy-MM-dd here m is for minute whereas M is for a month.
Let's take a look at the Constructors of the Class SimpleDateFormat
We'll make use of the format() method of the SimpleDateFormat class in this example.
Let's create an instance of it by using our date format :
Let’s use the format() method of the SimpleDateFormat class and create its instance using the date format :
Let’s see another simple example for formatting date in Java using the java.text.SimpleDateFormat class.
Output :
SimpleDateFormat class however, is not considered thread-safe and thus it shouldn’t be shared in a "multithreading" environment.
Multithreading :
is a feature in Java that allows concurrent execution or 2 or more sections of a program for maximum CPU utilization. A thread is a part of such a program.
Method - 2 : Using the Abstract DateFormat Class
We just saw the implementation of SimpleDateFormat which is a subclass of the abstract DateFormat class which provides different methods to format date and time.
Abstract DateFormat class can be used to achieve the following output :
We, then pass style patterns where MEDIUM is for the date and SHORT is for the time in this case.
Let’s see another simple example for formatting dates in Java using the java.text.DateFormat class class.
Output :
Method - 3 : Using the Formatter Class
Java formatter is a utility class which makes it simple to format stream output in java
Since we know the theSimpleDateFormat is not thread-safe we can use formatter class for cases where we require a thread-safe one-liner which is useful for a multi-threaded environment.
Here 1$ indicates one argument that passed to be used with every flag Let's deep dive into what
means
For every method that’s expected to produce a formatted output, we require a format string and an argument list. A format string however might contain a fixed text and one or more embedded format specifiers like in the following example :
the format method’s first argument is the format string, which contains three format specifiers %1$tm, %1$te, %1$tY indicating the way arguments should be processed and the place where they should be inserted in the text, the remaining portion is a fixed text "My Birthday: " and any other spaces or punctuation.
The argument list in the above example is of size one and consists of c as the calendar object
An argument list includes all the arguments that are passed to the method after the format string.
The syntax of format specifiers for types used to represent dates and time is :
The required conversion is a two-character sequence. The first character is t or T
Method - 4 : Using LocalDate.toString() Method
LocalDate is used to represent a date in ISO(International Organization for Standardization) format that isyyyy-MM-dd without time to store dates like birthdays etc
We can create an instance of the current date from the system clock as :
LocalDate can represent a specific day, month, and year by using the of() and parse() method.
We can represent the date using LocalDate as :
Let’s take a look at various API methods provided by LocalDate to obtain various information
To get the current local date and add one day :
To obtain the current date and subtract one month we can do the following Note how it accepts an enum as the time unit :
Let’s parse the date 2022-07-06 to get the days of week and month :
Here, the first return value is an object that represents DayOfWeek and the second return value represents the ordinal value of the month
To test if the current date occurs in a leap year
We can also determine the relationship of a date occurring after or before another date
Date boundaries can thus be obtained from the current given date
Here in the below example, LocalDateTime represents the beginning of the day of the given date and LocalDate represents the beginning of the month
Let's see an example to see how one converts a date object to a string using the LocalDate.toString() method
We first get an instance of LocalDate from the date after which the given date is converted into a string using the toString() method of the LocalDate class and the result is then printed.
Method - 5 : Converting Using Java 8 Date/Time API
The new date and time API was built to be thread-safe, intuitive, and mutable, unlike the old API.
DataTimeFormatter unlike the SimpleDataFormat class is immutable, thread-safe, and simpler to use with various predefined formats
Steps to change the date format of string in Java are the same asSimpleDateFormat and Date classes the only change is we’ll use the DateTimeFormatter and LocalDateTime class
Depending upon the desired pattern one can choose between the DateTimeFormatter class and LocalDateTime
The DateTimeFormatter class formats and parses the data in Java 8 and LocalDateTime representing a date with time in the local timezone.
Let’s see how we can use the DateTimeFormatter class and its format() method with the date patterns as :
To convert the Date object to an Instant object we are required to use a new API
We now need to convert Instantobject to LocalDateTime since our expected string contains both date and time.
We then get our formatted string :
LocalDate class can be used for patterns containing only a date, for converting patterns like yyyy-MM-dd hh:mm: ss to yyyy-MM-dd we can use the LocalDatetime class, let’s see an example.
Even though the steps are the same as that of the SimpleDateFormat class, DataTimeFormatter makes our code more expressive, thread-safe, and immutable, thus whenever we use JDK 8 we need to use the new Date and time API for the code we write related to data functionalities. This is why Date/Time API from Java 8 is considered to be more powerful than the java. util.Date and java.util.Calendar classes
Conclusion
- The formatted string from the date has to be a valid date format according to the Java specification.
- The process of how one converts a data object to a string starts with parsing the string to create data using the current format and then converting it to a string using a new format.
- SimpleDateFormat class can be used to change the date format for cases where we’re not running JDK 8 and are consecutively supposed to stick with an old date and calendar API.
- SimpleDateFormat class, however, is not considered thread-safe and thus it shouldn’t be shared in a multithreading environment.
- We can use formatter class for cases where we require a thread-safe one-liner which is useful for a multi-threaded environment.
- The DateTimeFormatter class formats and parses the data in Java 8 and LocalDateTime represents a date with time in the local timezone.