How to Open File with Java?
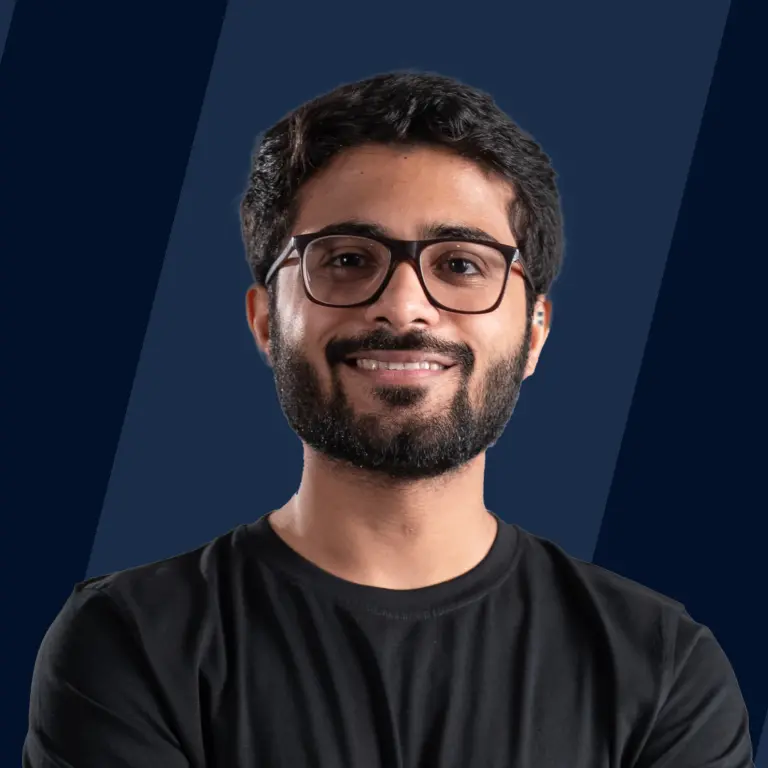
Overview
The programs run inside the main memory. But main memory is often cleared to make room for more programs to execute. So it becomes crucial for programmers to save and retrieve information from secondary storage. At this point, how to open files with Java and read them becomes crucial.
Introduction to How to Open Files with Java
How to open a file with Java has two interpretations. These are:
- You can launch a file with an associated application. For example, opening a text file (.txt extension) in a text editor (like Notepad in the case of Windows). You can achieve this through Java's Desktop class.
- You can also read a file's contents inside a Java program. It includes reading and printing the content on a console or utilizing it somewhere within the program. The four Java classes enabling this are:
- FileInputStream
- BufferedReader
- FileReader
- Scanner
Another way to efficiently read a file is by using the java.nio package introduced in JDK 4. The java.nio package is more efficient than others because it provides a non-blocking way of I/O.
Using Java Desktop Class
Unlike Java, the Desktop class is platform-dependent and it is a part of java.awt package. It is because the mechanism of registering, accessing, and launching the associated application is platform-dependent. Supported operations include:
- Launching the user-default browser to show a specified URI
- Launching the user-default mail client with an optional mailto URI
- Launching a registered application to open, edit or print a specified file.
Java Desktop class contains a method open() to launch a file with an associated application registered on the native desktop to handle it. If the specified file is a directory, the file manager of the current platform is launched to open it. It takes a File object as an argument, and its method signature is:
It throws the following exceptions:
- NullPointerException - If the file is null.
- IllegalArgumentException - If the specified file doesn't exist.
- UnsupportedOperationException - If the current platform does not support the Desktop.Action.OPEN action.
- IOException - If the specified file has no associated application or the associated application fails to be launched.
- SecurityException - If a security manager exists and its SecurityManager.checkRead(java.lang.String) method denies read access to the file, or it denies the AWTPermission("showWindowWithoutWarningBanner") permission, or the calling thread is not allowed to create a subprocess.
Let's see through an example how to open file with Java Desktop class.
Example:
Output: This will open a text editor showing the contents of the test-file.txt as follows:
Explanation: In the above example, we create a File object by passing the location as an argument. Then before using the instance obtained from Desktop.getDesktop() to open a file, we have to check whether the support for the Desktop class is present for the current system. If so, we get the Desktop instance through the getDesktop() method and use it to open a file by passing the File object created initially. This opens an associated application that can handle the file.
Using Java FileInputStream Class
Java FileInputStream class is used to open and read a file. It is meant for reading streams of raw bytes. It is achieved through the constructor. The signature of the class constructor is:
It throws the following exceptions:
- FileNotFoundException - if the file does not exist, or if the file is a directory, or for some other reason cannot be opened for reading.
- SecurityException - if a security manager exists and its checkRead method denies read access to the file.
Let's see how to open a file with Java FileInputStream class. Example:
Output:
Explanation: Here, we again create a File object. We utilize the FileInputStream class by creating its object through the parameterized constructor that accepts a file object. Then we read the file byte-by-byte until the end of the file (EOF) is reached. The EOF is represented by -1. The read method helps in doing so. It reads a single character and returns its value as an integer from 0 to 65,535. The input stream is closed through the close() method when the reading operation is complete.
Using Java BufferedReader Class
Java BufferedReader class also belongs to the java.io package. It is used to read text from a character-input stream. It buffers characters while reading in order to provide efficient reading of characters, arrays, and lines. The most commonly used constructor's signature is:
It creates a buffering character-input stream that uses a default-sized input buffer. The buffer size can also be specified, if required.
Let's see how to open file with Java BufferedReader class.
Example:
Output:
Explanation: As usual, we start by creating a File object. We can also check if the file exists through the exists() method. The BufferedReader class constructor accepts a FileReader object as opposed to the previous examples. It is created and passed as an argument to the constructor. BufferedReader also supports the read() method but has a subtle difference from that of FileInputStream. Here, it reads from a character stream instead of a byte stream. Finally, we close the stream after reading the file.
Using Java FileReader class
Java FileReader class is a convenience class for reading character files. It appropriately assumes the default character encoding and the default byte buffer size. However, you can specify them if you want.
It is meant for reading streams of characters. Some signatures for the constructor are:
The only exception thrown is the FileNotFoundException, if the file does not exist, or if its a directory, or for some other reason cannot be opened for reading.
Let's see how to open file with Java BufferedReader class.
Example:
Output:
Explanation: The FileReader class constructor can directly accept the file location to instantiate an object for reading a file. The read() method of FileReader also returns an integer value that we cast to a character. We use a similar condition in the while loop to read from the file until we reach EOF. The FileReader object is closed after reading the file contents.
Using Java Scanner class
Java Scanner class is a simple text scanner that can parse primitive types and strings using regular expressions.
A Scanner breaks its input into tokens using a delimiter pattern, which by default matches whitespace. The resulting tokens may then be converted into values of different types using the various next methods like next(), nextLine(), nextInt(), etc.
The constructor signature is:
The only exception thrown is the FileNotFoundException, if the source is not found. Let's see how to open file with Java Scanner class.
Example:
Output:
Explanation: In this example, we use a Scanner object to read the file contents. It is similar to how we read contents from the console using Scanner. Instead, we pass a file object to the Scanner constructor to create a connection with the file. The next methods help read and parse the content to the desired type. hasNextLine() method returns a boolean value that helps determine if another line is available to read. If so, we read the line using the nextLine() method that returns a String. Finally, we close the Scanner after reading the file.
Using Java nio Package
Java nio package is the "New I/O", i.e., it gives programmers new high-speed I/O classes and methods. It was introduced in JDK 4 and significantly updated in JDK 7. It follows a buffer-oriented approach and performs non-blocking I/O.
The two static methods of java.nio.Files intended for reading small files are:
readAllLines Method
The readAllLines method introduced in Java 8 can be used to open and read all lines from a file. The bytes from the files are decoded into characters using the UTF-8 charset. It is present inside the java.nio.Files. The method signature is as follows:
The Files.readAllLines returns lines from the file as a list. It recognizes the following as line terminators:
- CARRIAGE RETURN followed by LINE FEED
- LINE FEED
- CARRIAGE RETURN
The thrown exceptions include:
- IOException - If an I/O error occurs reading from the file or a malformed or unmappable byte sequence is read.
- SecurityException - In the case of the default provider, and a security manager is installed, the checkRead method is invoked to check read access to the file.
Let's see how to open a file with Java nio package's readAllLines static method.
Example:
Output:
Explanation: The example shows how we can read a file's contents easily. The static method readAllLines() of the java.nio.Files class accepts a Path object. We create a Path object using the static method get() present in the java.nio.Paths class that accepts a file location. Opening and closing of the file are taken care of by the readAllLines method. It returns a list of strings. Then, we traverse the list through a for-each loop to print its contents.
readAllBytes Method
You can also use the readAllBytes method which is present since JDK 7. It reads all the bytes from a file and returns a byte array. This byte array can also be easily converted into a string using the String constructor.
Exceptions thrown:
- IOException - If an I/O error occurs reading from the stream.
- OutOfMemoryError - If an array of the required size cannot be allocated, for example, the file is larger than 2GB.
- SecurityException - In the case of the default provider, and a security manager is installed, the checked method is invoked to check read access to the file.
Example:
Output:
Explanation: In the example, we use the readAllBytes method similar to the readAllLines method. It reads the file contents in the form of bytes and returns an array of bytes. Such an array of bytes is converted to a string using the String constructor that accepts a byte array. We then print this string to view the actual file content.
Conclusion
- Opening files and reading from them helps retrieve data stored in secondary storage.
- The various Java classes that allow us to open and read files are Desktop, FileInputStream, BufferedReader, FileReader, and Scanner. Java's nio package also provides support for reading from files.
- Desktop class is mainly used to launch a file with an associated application made to handle it.
- FileInputStream class is meant for reading streams of bytes.
- BufferedReader class makes use of buffers to read text from a character-input stream.
- FileReader is a convenience class for reading character files. It is meant for reading streams of characters.
- Scanner class is used as a simple text scanner. It can be used to read files with a default delimiter, or one of your choice. It can also be used to parse primitive values and strings using the next methods.
- Java's nio package introduces a new high-speed I/O capability. Its main features include non-blocking I/O and a buffer-oriented approach. Methods in the Files utility class like readAllLines and readAllBytes provides support to open and read files easily. They are not intended for reading large files.