Read, Write, Parse JSON File Using Python
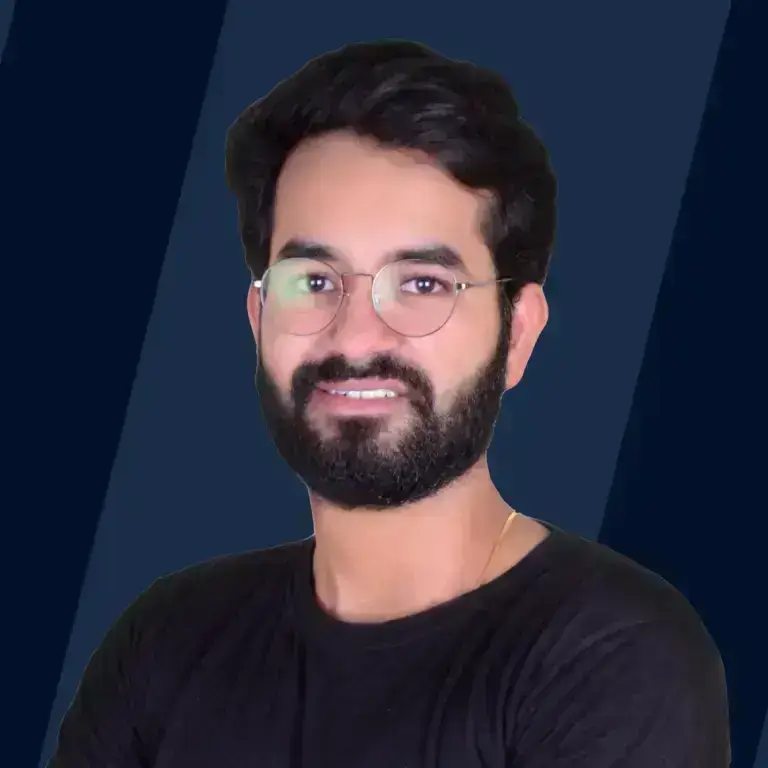
Say you want to transmit data from the front end of your website to the server, process the data, and send it back to the client side so that the users can view the results of your processing. Or, you want to write an application that requires the best of Python and JavaScript, and you want to be able to communicate data between both. All this is possible using JSON!
What is JSON?
JSON stands for JavaScript Object Notation and is a file format that stores data. JSON files are basically like Python dictionaries but on steroids. They help in storing data and enable communication with servers during deployment. More than a single programming language is often required to solve a problem, and JSON objects are a bridge between them. This article will explore how to parse JSON files with Python’s built-in JSON module.
How to Read JSON Files?
We’ll implement the code to open and read the json file
- Open the json file using the open() function
- Use the json.load() function and pass the file object
- Proceed by using the results of json.load() as a normal Python dictionary, and print the contents!
Here’s a sample json file. (example.json)
Code:
Output:
The output shows us the contents of the JSON file, which were obtained after traversing it line by line.
Note: Traversal depends on the JSON file, so you must know how it is structured. For example, a different file may have another parameter called gender, which must be accounted for in the code. A generalized code that traverses all JSON files can be developed using the type() function in Python, so give that a try!
How to Parse JSON?
Parsing refers to reading data (in various formats like strings, dictionaries, etc), analyzing it, and drawing useful inferences.
We’ll implement all the above functions to solve the following problems, as it is best to learn this topic via examples.
Problem 1
Parse the above example file after deserializing it with the json.load( ) function and count the lines to store the students' addresses. Store these values in a list with the person’s name and print the list.
Algorithm:
- Load the JSON file into a file object and use the json.load() function to deserialize it into a Python dictionary. Store this Python dictionary in a variable named jsonData.
- Loop over all people in jsonData and store their names and the number of address lines in variable data. Append data to result.
- Print the result.
Code:
Output:
The appropriate names and the number of lines used to save the address are displayed!
Problem 2
Open example.json with the json.loads( ) function and using it, create a new JSON file that stores only the mappings between the students’ names and their phone numbers.
Algorithm:
- Load the JSON file into a file object and read its contents with the file.read() function returns a string containing the file’s contents.
- Use the json.loads() function to convert this string object into the required Python dictionary and store the result in a variable jsonData.
- Create an empty dictionary phoneRecord. This will store the result
- Loop over all names in the jsonData and store the names of the people and their phone numbers as key-value pairs in the phoneRecord dictionary.
- Use the json.dump() function to create a new JSON file that contains the phone records.
Code:
Contents of output.json
Output:
We have successfully created a database that stores the names of all people along with their phone numbers!
Problem 3
Modify the example.json file to store the student addresses in a single string instead of a list of address lines.
Algorithm:
- Load the JSON file into a file object and use the json.load() function to deserialize it into a Python dictionary. Store this Python dictionary in a variable named jsonData.
- Loop over the names in jsonData and store the address lines in the address.
- Create an empty string addressString and loop over the address lines in address. Concatenate the text in each address line with addressString.
- Replace the address lines list with addressString.
- Open a new instance of the same file in write mode and use the json.dump() function to store the contents of jsonData in the file.
Code:
Contents of example.json (displaying only the address portions)
The file example.json now contains the entire address of all the people in a single string. This makes it easy to read for future purposes.
Python Convert to JSON String
When working with data in Python, one of the most common needs is to convert Python data structures into a JSON format for easy interchange with web applications, APIs, or other data storage and transmission mechanisms. The json module in Python provides a simple method, json.dumps(), for this purpose. This method is particularly useful for converting Python dictionaries, analogous to JSON objects, into JSON strings.
The json.dumps() method takes a Python dictionary and returns a string representation of that dictionary in JSON format. It ensures that all the keys and values in the dictionary are converted into a format that is compliant with JSON standards.
Example
Let's consider a simple example where we have a Python dictionary containing some user data and want to convert it to a JSON string.
Code:
Expected Output:
In this example, the json.dumps() method serializes the user_data dictionary into a JSON-formatted string. Note how Python's True, False, and None are converted to true, false, and null in JSON, respectively. Also, the array in Python (list) is converted to JSON.
This conversion method is vital in scenarios where Python applications must send data to web services or store data in a format that different programming languages can easily interpret.
Writing JSON to a File
Writing JSON data to a file is a common task in Python, especially when dealing with data persistence or data exchange between different systems. The json module in Python allows you to convert data structures to JSON strings using json.dumps() and provides a convenient way to write JSON data directly to a file using json.dump(). This method is beneficial when storing structured data in a file for later retrieval by either the same application or a different one.
Example
Let's consider an example where we have a Python dictionary containing some user data, and we want to write this data to a JSON file.
Code:
Explanation:
- We first import the json module.
- The user_data dictionary holds the data we want to write to the file.
- We open a file named user_data.json in write mode ('w'). If the file doesn't exist, it will be created.
- We write the dictionary to the file using the json.dump() method. The indent parameter is optional but recommended when you want the file to be human-readable, as it formats the JSON output with indented levels.
Resulting File Content (user_data.json):
Conclusion
To wrap up our exploration of handling JSON files in Python, here are some key takeaways:
-
Python's built-in json module provides a powerful yet simple interface for working with JSON data. It can handle many tasks, from converting basic data types to complex custom objects into JSON format and vice versa.
-
By using json.dumps() and json.dump(), Python makes it straightforward to serialize Python objects into JSON strings and write them to files.
-
Python's JSON tools offer flexibility in formatting and structuring the JSON output. Features like custom separators, indentation, and sorting keys ensure that the JSON data is machine-readable and human-friendly.
-
Python's json module provides clear error messages for common issues like serialization errors or file handling, which aids in robust and error-resistant coding.
-
The skills to read, write, and parse JSON files in Python are applicable in many domains, including web development, data analysis, automation scripts, and more, making it a valuable addition to any Python programmer's toolkit.
See Also
FAQs
Q: How to install JSON in Python?
A: Since Python’s JSON module is inbuilt, you do not need to install anything! Just have a working installation of Python, and you are good to go.
Q: How to format the output json format file while using json.dump( )?
A: If you use json.dump( ), usually, the entire file gets written into a single line, which is correct syntactically but doesn’t look appealing/readable. By changing the indent argument in json.dump( ), we can get the desired output
In Problem 2, replace the line,
With the line,
The output.json file now looks like this
The phone number database now looks cleaner and well-formatted!
Q: What is the json.dumps() function?
A: The json.dumps() function takes a Python dictionary and converts it to a Python string. This is useful in cases where string manipulation is used heavily. Usage:
Where Data is the Python dictionary you want to convert.
Q: How would this be used in real-life scenarios?
A: Well, one instance where you can use JSON files and the json python module is when you are working on a Flask or Django project, and when you need a way to communicate with Javascript that runs on the client’s web browser.
This application works because the user will submit a form (let’s say, his email or comments/feedback about something). This form can then be stored in a json object in JavaScript and then sent to the server side, which is built in Python.
Then, you could use the json module to deserialize this json file in the backend and process it. After processing the file, if you want to display the results, you could again use json.dumps() and sends the file to the user’s browser, where JavaScript will process and display the output information.
Pretty fantastic, right?