Python List sort() Method
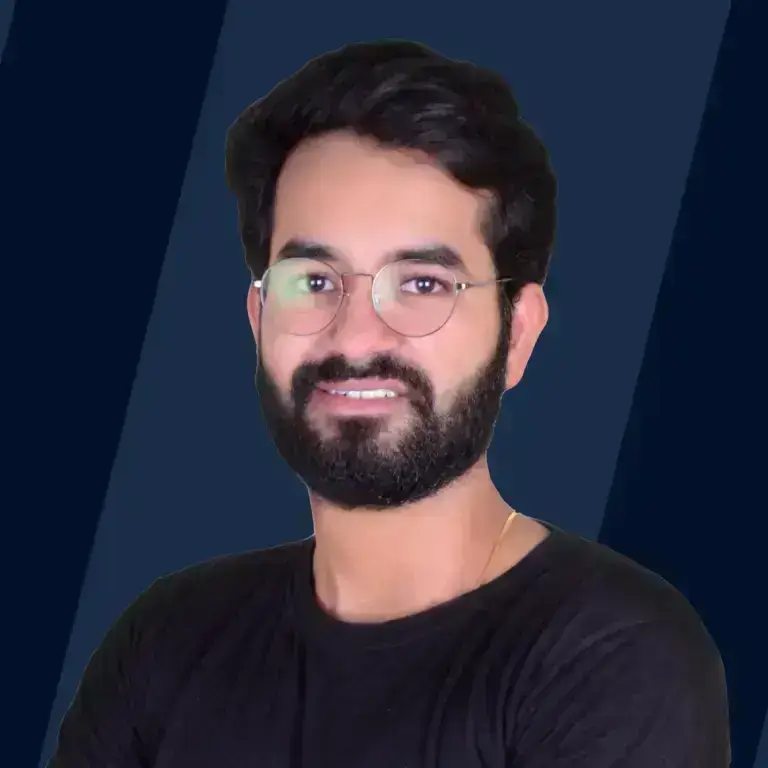
Sorting a list in Python is simple, allowing your code to run more efficiently. The sorted() function is the essential player in this scenario. It neatly puts the components of your list in ascending order, and you may change it to descending order if necessary. Use this code snippet: sorted_list = sorted(your_list). Alternatively, for descending order, sorted_list_desc = sorted(your_list, reverse=True). This simple yet effective Pythonic way keeps your lists properly organized, saving you coding challenges.
Python List sort() Syntax
Before learning about how to sort a list in Python, let us learn about the syntax of the sort() function in Python which we will use.
Sorting a list is a fundamental function in Python that every Python programmer should understand. Knowing how to sort lists might help you organise data for analysis or prepare output for improved reading. In this article, we'll look at the many ways Python provides for sorting lists effectively.
In Python, you can sort a list using the built-in sorted() function or by using the sort() method. Both methods offer flexibility and ease of use. The key difference lies in how they handle the original list. While sorted() creates a new sorted list, leaving the original list unchanged, sort() modifies the original list in place.
The sort() method is invoked on a list and can be applied to both numerical and string elements. The syntax is as follows:
Sorting lists in Python is an important skill that may greatly increase your programming productivity. Whether you use the sorted() function or the sort() method, knowing these fundamental principles provides the groundwork for more complicated sorting issues you may face on your Python journey. Practice and experimenting will improve your skills, so don't be afraid to plunge in and start sorting!
Python List sort() Parameters
Let us now look at various parameters of the sort() method in Python before learning about how to sort a list in Python.
- your_list: Replace this with the list you are required to sort.
- key (optional): This option allows you to provide a custom function that determines the sorting order. For example, you may utilize the key argument with a lambda function to sort by certain criteria.
- reverse (optional): If set to True, the list is sorted in descending order; otherwise, it is sorted in ascending order.
To sort in descending order, just alter the method call: your_list.sort(reverse=True). Use the key parameter to create unique sorting logic according to your requirements.
In conclusion, the sort() function is a useful tool for easily organising lists in Python. Its simplicity and adaptability make it an indispensable part of your coding toolset. Experiment with various circumstances, and you'll soon be mastering the art of list manipulation with Python's sort() technique.
Examples
Sorting a list is a fundamental operation in Python, and learning how to do it will establish a firm basis for your programming career. In this article, we will look at instances of sorting a list in Python, with an emphasis on putting members in ascending order.
Sort List Entries in Ascending Order
Let us now look at how to sort a list in Python in ascending order.
Suppose you have a list of numbers that you wish to organize in ascending order. Python offers a versatile and simple way to achieve this by utilising the sorted() function.
In this example, the function sorted() accepts the original list, numbers, and produces a new list, sorted_numbers, with the members sorted in ascending order. Running this code will display the sorted list, allowing you to easily verify the outcome.
Explanation
- The built-in Python method sorted() sorts any iterable, including lists.
- It does not alter the original list but rather generates a new sorted list.
- The original list is unaltered.
Additional Choices Python also has a sort() function for lists, which sorts them in place. Here's an example:
In this case, the sort() method modifies the original list, eliminating the need for creating a new variable.
Sort List Items in Descending Order
Let us now look at how to sort a list in Python in descending order.
Sorting a list in descending order involves ordering the components from highest to lowest. Python makes this procedure quite easy. with the sorted() function and the reverse parameter.
Example Code:
In this example, we establish a list named my_list that contains a sequence of integers. We use the sorted() method to sort the components in descending order, setting the reverse option to True. The resultant sorted list is saved in the variable sorted_list_desc. Finally, we print the original and sorted lists to compare.
Sorting lists in descending order is very handy when working with datasets, rankings, or any other situation in which you need to prioritize things from highest to lowest value.
Sort List Items with The Key Argument
Let us now look at how to sort a list in Python with key argument.
Before delving into the complexities of the key argument, let's go over the fundamentals of list sorting in Python. The built-in sorted() function is your go-to tool for this job. By default, it organizes the components in ascending order. For instance:
Output:
The important argument is where the magic occurs. It allows you to define a custom function that determines the sorting order. Assume we have a list of strings representing people's names and wish to arrange them depending on the length of their names.
Output:
The essential argument's strength stems from its adaptability. You can pass any function that accepts a list element as input and returns a result on which to sort. For example, consider sorting a list of tuples depending on the sum of their members:
Output:
Sorting a list using the key parameter in Python is a great technique for adapting the sorting process to your personal needs. Whether you're working with texts, integers, or more sophisticated data structures, the key argument offers a layer of control to guarantee your lists are organized just as you want them. Experiment with various functions to realize the full power of Python's sorting skills!
Conclusion
- When it comes to list sorting, Python's built-in method sorted() works like a charm. Its simplicity conceals a powerful tool that easily puts components in ascending order, allowing developers to concentrate on their logic rather than the complexities of sorting algorithms.
- One standout feature is Python's ability to sort a list in place using the sort() function. This not only saves memory but also gives a rapid and memory-efficient method for rearranging components inside the current list structure.
- Python's sorting capabilities go beyond the basics. Using the key option in the sorted() method, developers may tailor the sorting process to specific criteria. This opens up new possibilities, allowing users to easily sort lists of complicated things.
- The usage of lambda functions in combination with the key argument provides further flexibility in list sorting. This enables developers to design on-the-fly methods suited to particular sorting requirements, therefore increasing code expressiveness and conciseness.
- Python's sorting capabilities are not limited to ascending order. When the reverse argument is set to True, the sorting order is reversed, allowing lists to be arranged in descending order. A simple yet effective spin on the sorting tale.
- Python's sorting algorithms guarantee stability, which means that the relative order of equal elements does not vary. This intrinsic feature is very useful when working with datasets that need a hierarchical or multi-level sorting method.