Java HttpClient
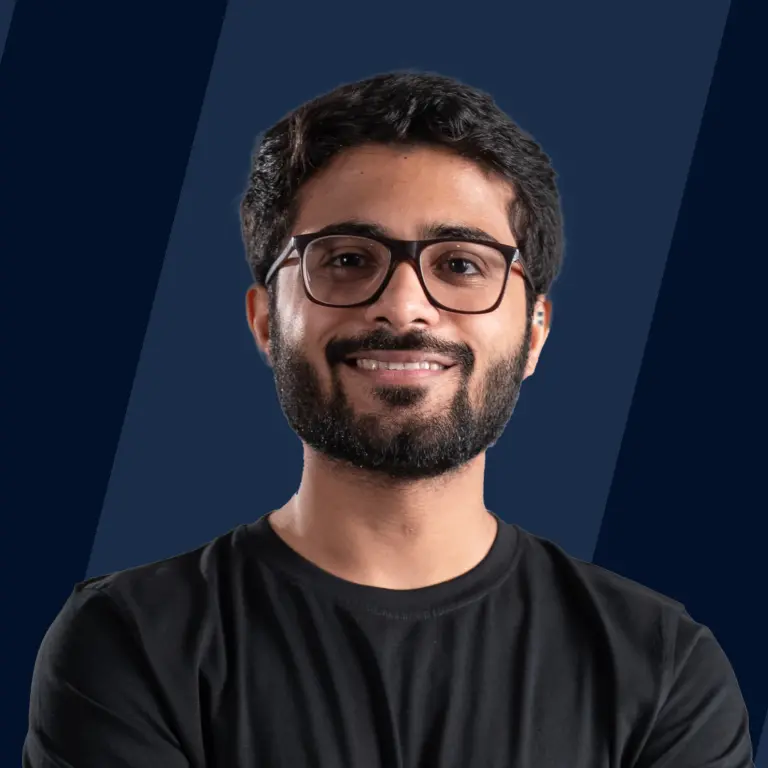
Overview
HTTP or Hypertext Transfer Protocol is a client-server protocol in the application layer. It involves a client sending a request to a server for data exchange. The server then sends a response back to the client. We can use HTTP to send HTML and API payloads in a standard format (XML or JSON). Since HTTP helps communication between applications via REST APIs, Java applications require a client to invoke such APIs. The HttpClient Java class is one of the native classes in Java 11 and above, which can be used as a client to send requests via HTTP and connect applications.
Introduction to Java HttpClient
The Java HttpClient was introduced in Java 9 as an incubator module and later standardized in Java 11 as part of JEP 321. It replaces the previously available low-level legacy Java API called HttpUrlConnection API, which was not feature-rich and user-friendly.
Some of the important features of HttpClient Java class are:
- Unlike legacy API, it is fully asynchronous and also supports synchronous mode of operation
- Supports HTTP/1.1, HTTP/2 and Web Socket
- Handling of request and responses as reactive-streams
- Provides support for cookies
The client, by default, sends the request using HTTP/2. But, if the server doesn't supports, it is downgraded to HTTP/1.1.
Along with all these features as a native support, there are following core classes and interfaces involved:
- The HttpClient Java class, java.net.http.HttpClient
- The HttpRequest Java class, java.net.http.HttpRequest
- The HttpResponse Java interface, java.net.http.HttpResponse
- The WebSocket Java interface, java.net.http.WebSocket
HttpRequest
HttpRequest is the type for objects representing the request one wants to send. The builder provides various methods to set states on per-request basis like the request URI, the request method (default is GET unless explicitly set), specific request headers, etc. Each of the setter methods like GET, PUT, POST, DELETE, setHeader, etc modifies the state of the builder and returns the same instance. The build method returns a new HttpRequest each time it is invoked. Once built an HttpRequest is immutable, and can be sent multiple times.
Declaration
HttpClient Java Class is part of the java.net.http package. It is an abstract class. We can send requests and retrieve responses through its instance. Also, builder, as its name suggests, is used in building an HttpClient. In the case of HttpClient Java Class, it is HttpClient.Builder.
The builder configures the state for every new client. The state could be like one or more of these:
- The preferred protocol version (HTTP/1.1 or HTTP/2)
- Whether to follow redirects
- Proxy information
- Authenticator
Finally, an HttpClient, once built, can send multiple requests. Therefore, for all the requests sent, it provides the following:
- Configuration information
- Resource Sharing
HttpRequest objects represent requests built using HttpRequest.Builder in a similar fashion to HttpClient.Builder. As we already know, each request sent results in a response from the server. So, we need to supply a HttpResponse.BodyHandler while sending a request to handle the response body (if any). HttpResponse represent responses, and once received, the following becomes available:
- Headers
- Response Code
- Body (usually)
Reading the response body bytes depends on the type, T, of the response body.
The requests are sent either Synchronously (send) or Asynchronously (sendAsync).
Nested Class Summary of Java HttpClient
Class | Description |
---|---|
static interface HttpClient.Builder | It builds HTTP Clients. |
static class HttpClient.Redirect | Defines the automatic redirection policy. |
static class HttpClient.Version | Represents the HTTP protocol version. |
Constructor Summary of Java HttpClient
Constructor | Description |
---|---|
protected HttpClient() | It is responsible for HttpClient creation. |
Constructor Detail
protected HttpClient()
The constructor is used to create an instance of HttpClient. It is a protected constructed which means it cannot be directly used by the user to create a client. Instead, it is utilized by classes present inside the same package.
Method Summary of Java HttpClient
Method | Description |
---|---|
abstract Optional | It returns an Optional containing the Authenticator configured for the client. |
abstract Optional | It returns an Optional containing the connect timeout duration for the client. |
abstract Optional | It returns an Optional containing the client's CookieHandler. |
abstract Optional | It returns an Optional containing the client's Executor. |
abstract HttpClient.Redirect followRedirects() | It returns the policy for following redirects for the client. |
static HttpClient.Builder newBuilder() | It creates a new HttpClient builder which helps in configuring various options. |
static HttpClient newHttpClient() | It returns a new HttpClient with default settings. |
WebSocket.Builder newWebSocketBuilder() | It creates a new WebSocket builder. It is optional operation. |
abstract Optional | It returns an Optional containing the ProxySelector configured for the client. |
abstract | It sends the request synchronously using this client. It is blocking, i.e., waits until the response is available. |
abstract | It sends the request asynchronously using the client. A handler is supplied for handling the response body. |
abstract | It is the overloaded method which accepts an additional parameter - push promise handler. Push Promise is a new feature in HTTP/2. |
abstract SSLContext sslContext() | It returns the client's SSLContext. |
abstract SSLParameters sslParameters() | It returns a copy of the client's SSLParameters. |
abstract HttpClient.Version version() | It returns HTTP protocol version preferred for this client. |
Method Detail
1. newHttpClient Method
It returns a new HttpClient with default settings. It is equivalent to newBuilder().build().
By default, settings are:
- GET request method
- HTTP/2 protocol version preference
- Redirection policy of NEVER
- The default proxy selector
- The default SSL context.
Implementation Note: When we build an HttpClient instance, the system-wide default values are retrieved. There is no effect of changing these values on the already constructed HttpClient Java class instance. For example, calling ProxySelector.setDefault(ProxySelector) or SSLContext.setDefault(SSLContext) does not affect already-built clients.
2. newBuilder Method
It creates a new HttpClient Java class instance builder. The returned object is of type HttpClient.Builder.
3. cookieHandler Method
It returns an Optional containing the client's CookieHandler. If no CookieHandler was set in this client's builder, then the Optional is empty.
4. connectTimeout Method
It returns an Optional containing the connect timeout duration for the client. If the connect timeout duration was not set in the client's builder, then the Optional is empty.
5. followRedirects Method
It returns the follow redirects policy for this client. By default, the follow redirects policy is NEVER.
6. proxy Method
It returns an Optional containing the ProxySelector configure for the client. The Optional is empty if no proxy selector was set in this client's builder.
Note: The HttpClient Java class instance may still have a non-exposed default proxy selector for sending HTTP requests even though the method returns an empty optional.
7. sslContext Method
It returns the client's SSLContext. The default context is returned if no SSLContext was set in the client's builder.
8. sslParameters Method
It returns a copy of the client's SSLParameters. The client uses an implementation-specific default set of parameters if no SSLParameters were set in the client's builder.
9. authenticator Method
It returns an Optional containing the Authenticator set on the client. The Optional is empty if no Authenticator was set in the client's builder.
10. version Method
It returns the preferred HTTP protocol version for the client (default value is HttpClient.Version.HTTP_2). Please note that requesting a HTTP/2 through a proxy (set for the client) may use HTTP/1.1 version. It can happen when proxy's implementation doesn't support HTTP/2.
11. executor Method
It returns an Optional containing the client's Executor. If no Executor was set in the client's builder, then the Optional is empty.
Implementation Note: The HttpClient may still have a non-exposed default executor for executing asynchronous and dependent tasks, even though the method returns an empty optional.
12. send Method
It sends the given request synchronously using the client. The returned HttpResponse<T> contains the following response information:
- Status
- Headers
- Body (handled by the given response body handler ).
Parameters: T - The type of response body request - The HttpRequest object responseBodyHandler - HttpResponse.BodyHandler
It can throw following exceptions:
- IOException : When an I/O error occurs during sending or receiving.
- InterruptedException : When the operation of sending or receiving in a thread is interrupted.
- IllegalArgumentException : When the request argument is an invalid request object.
- SecurityException : When access to the URL in the given request is denied.
13. sendAsync Method
It sends the given request asynchronously using the client with the given response body handler.
It is equivalent to sendAsync(request, responseBodyHandler, null). All the parameters are same as in send method of Java HttpClient API.
It throws an IllegalArgumentException when request argument is an invalid request object.
14. sendAsync Method with PushPromiseHander
It is an overloaded sendAsync method. It sends the given request asynchronously using the client with the given response body handler and push promise handler.
It returns a completable future (a CompletableFuture<HttpResponse<T>>), which completes with an HttpResponse<T> (if succesful) that contains the response status, headers, and body.
If any Push Promises are received, then those are handled by the given pushPromiseHandler. A null valued pushPromiseHandler rejects any push promises. The Push Promises are part of a new feature introduced in the HTTP/2 protocol version.
The returned completable future completes exceptionally with:
- IOException - When I/O error occurs during sending or receiving
- SecurityException : When access to the URL in the given request is denied.
Parameters: It has all parameters required for the previous sendAsync method except for an additional parameter, pushPromiseHandler.
This method of the HttpClient Java class throws an IllegalArgumentException if the request argument is an invalid request.
15. newWebSocketBuilder Method
It creates a new WebSocket builder which is an optional operation. It returns a WebSocket.Builder.
Implementation Requirements: The default implementation of the method throws UnsupportedOperationException. Clients obtained through newHttpClient() or newBuilder() return a WebSocket builder.
Implementation Note: Both builder and WebSockets created with it operate in a non-blocking fashion, i.e., their methods do not block before returning a CompletableFuture. Asynchronous tasks execute in this HttpClient's executor. When a CompletionStage returned from Listener.onClose completes, the WebSocket sends a Close message having the same code the received message has and an empty reason.
The method throws an UnsupportedOperationException if the HttpClient does not provide WebSocket support.
Java HttpClient Examples
a. HttpRequest
HttpClient Java class instance uses HttpRequest objects to send the requests. So, let's first explore methods that help create an HttpRequest object suiting a developer's need.
1. Set URI
Another way is to use uri() method:
2. Specify HTTP Method
One can call these methods on HttpRequest.Builder instance:
- GET() - It sets the request method for this HttpRequest builder to GET. Use it for resource retrieval.
- POST(BodyPublisher body) - It sets the request method for this HttpRequest builder to POST. Such a request also requires a request payload (for resource creation) of type HttpRequest.BodyPublisher.
- PUT(BodyPublisher body) - It sets the request method for this HttpRequest builder to PUT. Similar to POST request, a PUT-type request requires a request body for resource updation.
- DELETE() - It sets the request method for this HttpRequest builder to DELETE. Use it for resource deletion.
3. Set HTTP Protocol Version
The two HTTP Protocol Versions supported by HttpClient Java API are:
- HTTP/1.1
- HTTP/2 (used by default)
4. Set Headers
Another way is to use header() method:
As we can see, the header() and headers() method is used to set some key-value pairs to the request. These are generally used to set metadata for the requests.
5. Set Timeout
Timeout is the duration for which we wait for a response. If time expires, a HttpTimeoutExeption is thrown.
Please note that is we don't specify timeout duration manually, it is set to infinity, by default.
6. Set Request Body
The request builder methods like POST(BodyPublisher body) and PUT(BodyPublisher body) help add a request body to a request. The API provides implementations of BodyPublisher that implement various useful publishers, such as publishing the request body from a String, or from a file. These implementations can be:
StringProcessor It reads request body from a String. It is created with HttpRequest.BodyPublishers.ofString:
InputStreamProcessor It reads request body from an InputStream. It is created with HttpRequest.BodyPublishers.ofInputStream:
Here, the input stream (which could be a ByteArrayInputStream or any other InputStream) is passed as a Supplier. This ensures that it's creation is lazy.
ByteArrayProcessor It reads request body from a byte array. It is created with HttpRequest.BodyPublishers.ofByteArray:
FileProcessor It reads request body from a file at the given path. It is created with HttpRequest.BodyPublishers.ofFile:
Handling the case where no request body is required
b. HttpClient
HttpClient Java class is used to send the request and receive a response. The responses are received in the form of HttpResponse objects. After sending a request through client, the received response bodies can be handled using following BodyHandlers:
Let's move on to explore some real examples. All the examples discussed follow these steps:
i. Instantiate HttpClient Java class using HttpClient.newBuilder() or HttpClient.newHttpClient(). ii. Instantiate HttpRequest Java class using HttpRequest.newBuilder(). iii. Send a request using appropriate send() method on the instance of HttpClient. iv. Inspect the stored response (result of sending the request to server using client).
1. Sending Requests Synchronously
Synchronous sending of request means that the Java Program waits for the response from the server after sending the request. This blocks the execution of next set of instructions in the program.
GET request
Output:
Explanation: First, we create an HttpRequest object that sends a GET request using a URI. If we do not use the GET() method, then, by default, a GET request is prepared. Next, we create a simple HttpClient instance. Finally, we send the request through the client using the send() method. The send method may throw some pre-defined exceptions. Therefore, we handle it using a try-catch. The synchronous send() method returns a HttpResponse object. The response status code and the body is available in the response object. Here, we obtain a 200 status code with a response body, signifying a successful request. The output obtained may change (in future executions), but the concept will remain the same.
POST
Output:
Explanation: Here, we create a POST-type request and send it via an HttpClient. Generally, for a POST request, a request body is required. Therefore, we make a JSON payload (JSON String) which contains information about the resource to be created in the server. We need to pass this JSON string for creating a request object, so BodyPublishers.ofString() method is used to set this information. This time we configure our HttpClient to use the HTTP/1.1 protocol version (HTTP/2 is the default) with a request timeout duration of two seconds. We obtain a status code 201. It means the resource creation was successful. The request gives us the ID of the newly generated. The server also answers in HTTP/1.1 protocol which is the same as what we defined for the client. But, requests sent to servers that do not yet support HTTP/2 will automatically be downgraded to HTTP/1.1.
2. Sending Requests Asynchronously
Asynchronous sending of a request means that the Java Program does not wait for the response from the server after sending the request. It is a non-blocking way of sending and processing requests where the execution of the next set of instructions continues irrespective of whether a response is obtained from the server or not. The sendAsync() method of the HttpClient Java Class instance can send async requests to a server. It returns an object of CompletableFuture, which contains a HttpResponse instance.
Output:
Explanation: Here, we create another HttpClient Java class instance. It is similar to the previous example. This time instead of send() method, we call the sendAsync() method. This method immediately returns an instance of CompletableFuture. But the HttpResponse is not available instantly. CompletableFuture completes with the HttpResponse when it becomes available. Java 8 introduces CompletableFuture for enhancing asynchronous programming. The thenApply() accepts a Function type lambda expression. It maps the value contained inside CompletableFuture to another. It returns another CompletableFuture object, which now has the mapped value. Then, we call a get() method which returns the result of the mapping method. In our case, we map the HttpResponse to its response body which is a string. Finally, that response body is extracted through the get() method.
3. Head Request and Obtaining Headers From the Response
Output:
Explanation: A GET request without a request body is called a HEAD request. The newHttpClient() method creates a default HttpClient Java Class instance. We create an HttpRequest object using some previously discussed methods. The HEAD type request is built using the request builder method method(), which takes in the name of the request type and information about the request body through one of the implementations of BodyPublishers. BodyPublishers.noBody() is used for a HEAD request because it doesn't include a request body. A response is returned in the form of a HttpResponse object after sending the request through the client. We can call the headers() method to obtain the HttpHeaders object. The map() method of the HttpHeaders object gives us a read-only Map, which is iterated similarly to other Map data structures.
4. Authentication
To perform a sample authentication, we use the URL: https://httpbin.org/basic-auth/Sam/Sam123. We can compare the request URL with https://httpbin.org/basic-auth/{user}/{password}. One can easily replace {user} and {password} with some arbitrary values for testing purposes.
Output:
Explanation: This time, we create an HttpClient Java Class instance by setting up an Authenticator while building it. We pass an object of Authenticator to the authenticator() HttpClient builder method. Here, we use an anonymous inner class to create an Authenticator instance that returns a username of "Sam" and a password of "Sam123". The getPasswordAuthenticator() method returns the required credentials for authentication purposes; hence, we override it. It is clear from the output that the response received signifies a successful authentication took place (200 Status code).
c. HttpResponse
1. Get URI of Response
The URI of a response retrieved from the server may be different. It can happen because of a redirection.
The below example uses the URL https://mockbin.org/redirect/302/1/?to=https://scaler.com/topics to test only one redirection with a status code of 302 to the Scaler Topics website.
Output:
Explanation: The HttpClient Java Class instance is created using its builder methods. The followRedirects() method defines a RedirectPolicy for the client. The default policy is set to RedirectPolicy.NEVER. But here we set the policy to RedirectPolicy.ALWAYS to observe a change in URI when there's a redirection. It is observed in the output that the request and response URI do not match because a redirection has happened after sending the request.
2. Get Headers of a Response
The headers() method, when called on a retrieved HttpResponse object, returns an HttpHeaders object. As discussed in the previous examples, we can also iterate on the HttpHeaders object using the map() method.
3. Get Version of a Response
The version() method can help obtain the HTTP protocol version with which the server has answered. It is useful when we want to detect if the server answers via an HTTP/2 protocol version.
Conclusion
- HttpClient Java API eliminates the dependency on 3rd-party libraries for sending HTTP requests and responses.
- It was introduced in Java 9 & standardized in Java version 11. It requests HTTP resources over the network.
- It is fully asynchronous and also supports synchronous mode.
- The synchronous API blocks until the HttpResponse is available. The asynchronous API returns immediately with a CompletableFuture that completes with the HttpResponse when it becomes available.
- HttpClient Java API supports HTTP/1.1 and HTTP/2 protocol versions.
- There are three nested classes of HttpClient Java class: HttpClient.Builder, HttpClient.Redirect & HttpClient.Version
- Some of the most commonly used of HttpClient are: newHttpClient(), newBuilder(), send() and sendAsync() methods.
- The four basic steps to send requests and retrieve responses from an HTTP server: Creation of HttpClient, HttpRequest, HttpResponse and, finally, sending the request (sync or async) via client by passing in a request object to it and retrieving a response as a result.
- HttpClient Java Class object provides configuration information and resource sharing for all sent requests through it.
- A HttpClient Java object sends a request built through HttpRequest class.
- For each HttpRequest sent, a BodyHandler is specified, which determines the handling of the request body.
- The object of HttpResponse class stores the retrieved response from the server.
- The request body can be set for request methods like POST and PUT. There are various out-of-the-box implementations of BodyPublishers which help define the request body, like StringProcessor, InputStreamProcessor, ByteArrayProcessor & FileProcessor.
- Similarly, the response bodies are taken care of through BodyHandlers like BodyHanders.ofByteArray, BodyHandlers.ofString, BodyHandlers.discarding, and many more.