How to Zoom an Image on Hover Using CSS?
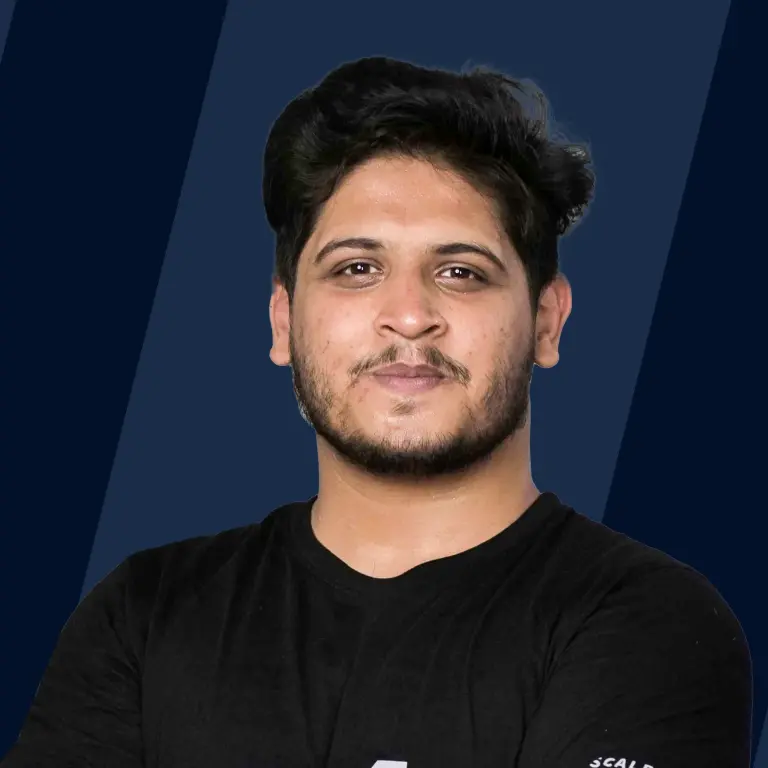
Overview
Zooming an image on hover using CSS is a straightforward and visually appealing effect that can enhance user experience on your website. To achieve this, you'll need to employ CSS properties like transform and transition.
First, select the image you want to zoom and apply the initial styling, usually using a CSS class. Then, set the transition property to control the animation speed. When a user hovers over the image, use the
Pre-requisites
Before you can successfully create a zoom effect on an image using CSS, you should ensure you have the following prerequisites in place:
- HTML Structure: Have a well-structured HTML document with an image element that you want to apply the zoom effect to. Ensure the image is embedded within an appropriate container, like a <div>.
- Image: Prepare the image you want to use. Make sure it is correctly sourced using the <img> tag and that it's appropriately sized for your design.
- Basic HTML and CSS Knowledge: Familiarize yourself with basic HTML and CSS concepts, including HTML tags and attributes, CSS classes and selectors, and CSS properties like width, height, and margin.
- Text Editor: Use a code editor or text editor of your choice, such as Visual Studio Code, Sublime Text, or Notepad++, to write and edit your HTML and CSS code.
- Web Browser: You will need a web browser like Google Chrome, Mozilla Firefox, or Microsoft Edge to preview and test your HTML and CSS code.
- CSS Stylesheet: Create an external CSS stylesheet (e.g., styles.css) or include your CSS styles in a <style> tag within the HTML document. Ensure you link or include this stylesheet in your HTML file.
- CSS Transition Properties: Understand CSS transition properties like transition, transform, and hover, which are essential for creating the zoom effect.
- Text Editor: Familiarity with a text editor for writing and saving your HTML and CSS files.
- Transition Timing: Set the transition property to control the speed and timing function of the zoom effect. This determines how smoothly and quickly the image transitions between its normal and zoomed states.
- Testing: Regularly test the hover effect in different browsers to ensure cross-browser compatibility and responsiveness.
By addressing these prerequisites, you'll be well-prepared to create a smooth and visually appealing image zoom effect using CSS while ensuring compatibility, accessibility, and a polished user experience.
How to Zoom an Image on Mouse Hover Using CSS?
HTML Code
- We start with the standard HTML5 document structure.
- We include a <link> tag in the <head> section to link an external CSS stylesheet named "styles.css."
- Inside the <body>, we create a <div> with a class of "image-container" to hold our image.
- Inside the <div>, we insert an <img> element with a source (src) attribute pointing to the image you want to zoom. We also give it an "alt" attribute for accessibility and add a class of "zoom-image" for styling.
CSS Code
- We start by styling the "image-container" to be an inline block element with relative positioning and hidden overflow. This ensures that the image doesn't overflow its container during the zoom effect.
- The "zoom-image" class is applied to the image. We set its width to 100% to make it responsive, and we use height: auto to maintain the image's aspect ratio.
- We add a transition property to the "zoom-image" class to create a smooth animation when the image transforms.
- The hover effect is defined with the "zoom-image
" selector. When the image is hovered over, we use the transform property to scale it by a factor of 1.2 (20% larger), creating the zoom-in effect.
Complete Code
- In the HTML code, we create a basic HTML document structure. Inside the <body>, there's a <div> with the class "image-container" that wraps an <img> element. Replace "your-image.jpg" with the actual path to your image file.
- In the CSS code, we start by styling the body element to center its content both horizontally and vertically. We also remove default margins and padding.
- The "image-container" class is used to wrap the image. It is set to position: relative to establish a positioning context for the contained image and overflow: hidden to ensure that the image doesn't overflow its container. You can adjust the width and height to determine the size of the image container.
- We apply a transition property to the "image-container" class to create a smooth zoom effect. The transform property is animated over a duration of 0.3 seconds with an ease-in-out timing function.
- On hover (.image-container
), we use the transform property to scale the image to 1.2 times its original size, creating the zoom effect. Adjust the scale factor as needed for your desired level of zoom. - We also apply similar transform and transition properties to the img element within the container, ensuring that the image smoothly zooms in and out.
Conclusion
- HTML Structure: Create a well-structured HTML document with an image element enclosed within a container.
- CSS Stylesheet: Use an external CSS stylesheet or <style> tag to apply styles to your HTML elements.
- Image Container: Wrap the image in a container (<div> in this example) to control the zoom effect.
- CSS Transition: Apply the transition property to the container to create smooth animations.
- Hover Effect: Use the
pseudo-class to target the container when the mouse hovers over it. - Transform Property: Utilize the transform property to scale the image within the container when hovered.