Why Declaring a Class Abstract is Useful in Java?
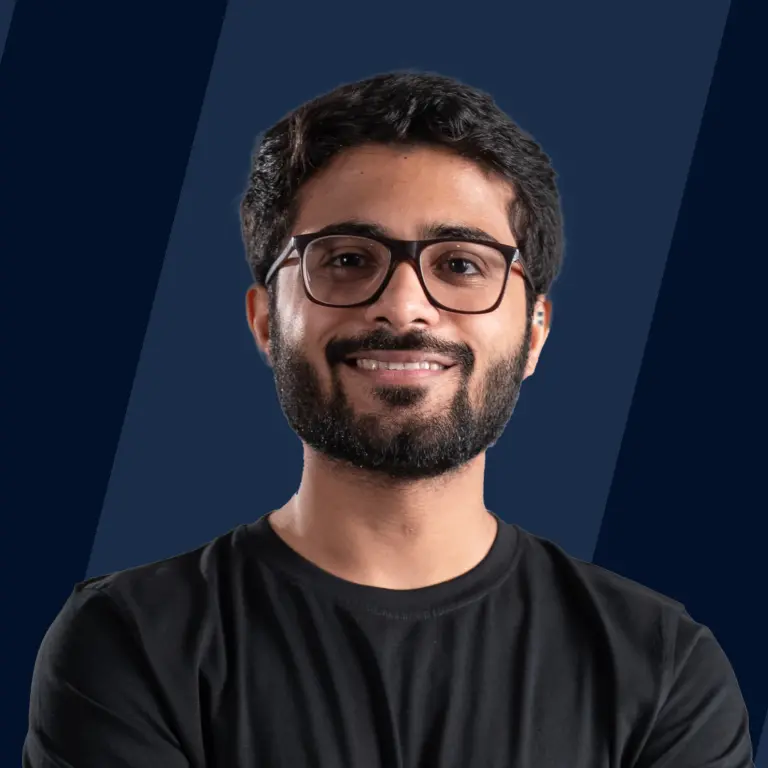
Overview
In Java, classes have methods that have a signature and an implementation (which can be outside the class as well). Sometimes we wish to create methods for which we only know the signature for the time being but need to defer its implementation later. Why would we need to do this? Maybe we know how our class should behave partially; we know how few methods should behave, but the others are a black box to us. We want to give future developers the extensibility to write their whole implementations while following a certain template. Abstract classes help us in declaring such incomplete classes in Java.
You can read more about the implementation of an abstract class in this blog
Why Do We Need an Abstract Class in Java?
An abstract class can be used as a template for other classes. The abstract class will hold common functionality for all classes that extend it. Declaring a class abstract is useful when it doesn't make sense to have objects of that class.
Abstract classes permit providing a partial set of default implementations of methods in a class. They can't be instantiated and used in their current state because they are incomplete, but they can be subclassed to add the missing information in a way that is unique to that implementation, and those subclasses can be instantiated.
Let us understand why we need to create an abstract class by taking a real-life example. Suppose that you are a developer working for a burger joint. As of now, the burger joint serves three types of burgers
- CheeseBurger
- PaneerBurger
- ChickenBurger
Each burger has a patty, cheese, bun, and different toppings based on the type of the burger. All 3 burgers have different patties, the cheese burger has a veg patty, the paneer burger has a patty made of paneer, and the chicken burger has a patty made of chicken. However, the buns in all burgers are the same. The cheese is also the same, but the cheese burger has an extra cheese slice.
You create the following 3 classes to support the creation of these burgers:
CheeseBurger:
PaneerBurger:
ChickenBurger:
While writing the detailed implementations of these methods, you quickly see the following patterns:
- makeBun() and assembleBurger() implementations are the same
- makePatty() has a different implementation in all 3 burgers
- addCheese() is same in PaneerBurger and ChickenBurger but different in CheeseBurger
If we could reuse code by keeping the methods that have a common implementation in one place, wouldn't that be so much convenient?
If we abstract out the common implementations in a separate class and leave the methods for which we do not have a concrete implementation, we can come up with a Burger class as follows -
Note that only makeBun() and assembleBurger() have an implementation. makePatty() and addCheese() do not have one (only their method signature is defined), and hence need to qualified with the keyword abstract. The makePatty() and addCheese() methods are an abstraction to the Burger class. Now the thumb rule is that even if one method is declared abstract, the class needs to be qualified with the abstract keyword
Any class which extends an abstract class and implements all its abstract methods is called a concrete class. Essentially, a concrete class does not have any abstract methods. A concrete class can choose to override the already defined methods, however, defining the abstract methods is a must. Let us now implement one of the concrete burger classes by taking the example of CheeseBurger.
Voila, we have made implementing new burger recipes very easy!
Now let us say we wish to introduce a new line of burgers which have a wheat bun. Even though makeBun() was not an abstract method, if any new burger wishes to make wheat buns, they can still do so by using simple class extension principles
Rules for Using Abstract Class in Java
- An abstract class must be declared with an abstract keyword
- An abstract class must have at least one abstract method. If not then the class is a concrete class
- An abstract class cannot be instantiated. This is because an abstract class is incomplete, the abstract methods have no definition. Only if a class that extends an abstract class is concrete can one instantiate the concrete class
- However, an abstract class can have constructors as well as static methods
- If we need to force the subclasses not to change the implementation of the method we can declare the method as final
Conclusion
- Abstract classes in Java help us to declare a template of how a class should behave and take some decisions on how few methods should be implemented at a later point in time
- By creating abstract classes we can enhance the reusability of code in our applications * Abstract classes should be used when we have multiple classes with some common functionalities and we see a pattern that might repeat in the future