What is Used to Create a Thread in Java Multithreading?
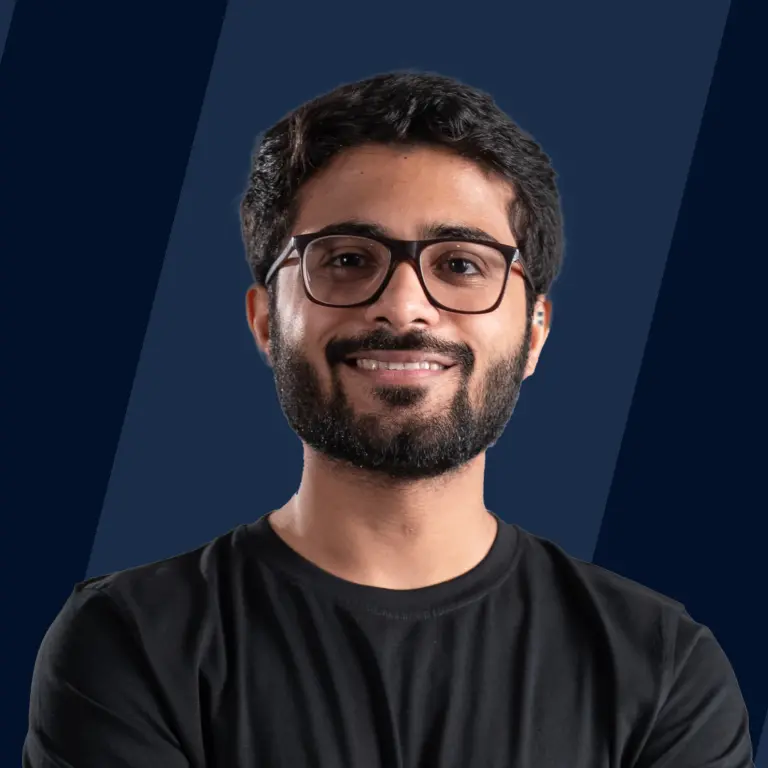
What is Used to Create a Thread in Java Multithreading?
A thread is a lightweight subpart of a process that can run independently and concurrently with other parts of the same process. The execution of one thread is independent of the other threads. However, the failure of one thread can affect the execution of other threads if they are accessing shared resources.
Execution of multiple threads of the same process simultaneously is known as multithreading. Multithreading reduces the time taken by the process for task completion and maximizes CPU utilization. All the threads of a process share a common memory space.
In Java, multithreading a thread can be created by the following two ways:
- By extending the thread class
- By implementing a Runnable interface
Some of the useful methods of Thread Class that we are going to use:
- start() - It starts the execution of the thread in a separate path and then calls the run() method.
- run() - It performs the action determined in the run() method.
- isAlive() - It returns true if the execution of the thread is not terminated, else it returns false.
- join() - It waits for the thread to die.
- sleep() - To suspend a thread for a certain period of time.
Method 1 by extending the Thread class
In java multithreading, a thread can be created by extending the java.lang.Thread class. We first create a class that extends Thread and overrides the run() method. Now thread can be created by creating the object of our newly created class and calling the run() method.
Let us take an example of a method that finds the given target element from the array. As the array is not sorted the only efficient way is to traverse the array. Usually, it takes O(n) time to traverse the array where n is the number of elements in the array. Now, we can use multiple threads to reduce the time complexity to find the element in the array.
Note: In Java, java.lang package is a default package; therefore without importing it explicitly we can access all the classes present in the package.
Output:
We created the class Multiplethreading that extends the Thread class and override the run() method. The run() method traverses the array, and if the element is found, it saves its index in the index variable. In the main method, we divide the array into two, and both threads independently traverse different parts of the array. Lastly, we return the first occurrence of the target element.
As the threads are running parallelly, we can find the element in O(n/2) time complexity even in the worst case. If the number of elements in the array is much larger, we can divide the array is many small parts and achieve even lesser time complexity. Also, we are waiting for both threads to complete using the while loop so that the remaining part of the program doesn't return before the threads finish processing.
Method 2 by Implementing The Runnable Interface (add example)
The another way in java multithreading a thread can be created by implementing the Runnable interface present in java.lang. It is one of the standard Java interfaces that come with the Java platform. It also has a run() method, which is to be overridden by our class.
To create a thread using the Runnable interface,
- our thread class must implement the runnable interface
- the thread class must override the run() method
- the object of the java.lang.Thread class say obj1 and the object of our thread class say obj2.
- passing the obj2 as a parameter to obj1.
Let us take an example of a class implementing a Runnable interface for a library. One thread in the process counts the number of copies of the book available, and the other thread takes the user information for registration.
Output:
In the example above, the Person class extends the Runnable interface. It has three methods, putInfo() just adds details to the class variables, getInfo() asks data from the user, and run() is an overridden method that calls getDetails().
Library class also implements a Runnable interface. It has an array books that stores a list of all the books. Here the run() method counts the number of books present in the library. In the main method, we create objects of the Library class and Person class to call their run() methods, respectively. We wait for the details thread to finish processing before printing the result of the bookCount thread.
Note: Both the thread ran simultaneously, and the result was calculated. We are only printing it late to avoid clashing with the output.
Thread Class vs Runnable Interface
Thread Class | Runnable Interface |
---|---|
It is a class and in Java, a class can only extend one parent class i.e. it doesn't support multiple inheritances. So, we cannot extend any other classes. | It is an interface thus the class can implement multiple interfaces and also extend a parent class as well. |
We cannot reuse the object of the thread. For each new operation, a new object of the class is required. Thus, it uses more memory. | It is possible to reuse the same object to execute multiple threads by implementing a runnable interface. It requires less amount of memory space. |
It is inheritance. | It provides composition i.e. we are not modifying or specifying the behavior of the threads. We are just asking it to run something. |
It has multiple methods that are used to perform actions on the thread. | It has only an abstract method run(). |
Learn more about threads in Java
Conclusion
- In java multithreading a thread can be created in two ways i.e. using the Thread class and using the Runnable interface.
- If our class extends thread class then by creating its objects, we are creating threads. We are required to override the run() method of the Thread class to specific actions to be performed by these threads. Using the start() method we can start a separate path for the execution of the thread and it later invokes the run() method.
- By implementing the Runnable interface, we are allowed to implement multiple interfaces and also extend a parent class, unlike the first method.
- We are required to override the run() method and create an object of the class as well as the Thread class (which can further be reused) to implement multiple threading in java.