In Python, What is Returned When Evaluating [n for n in range(10) if n % 2]?
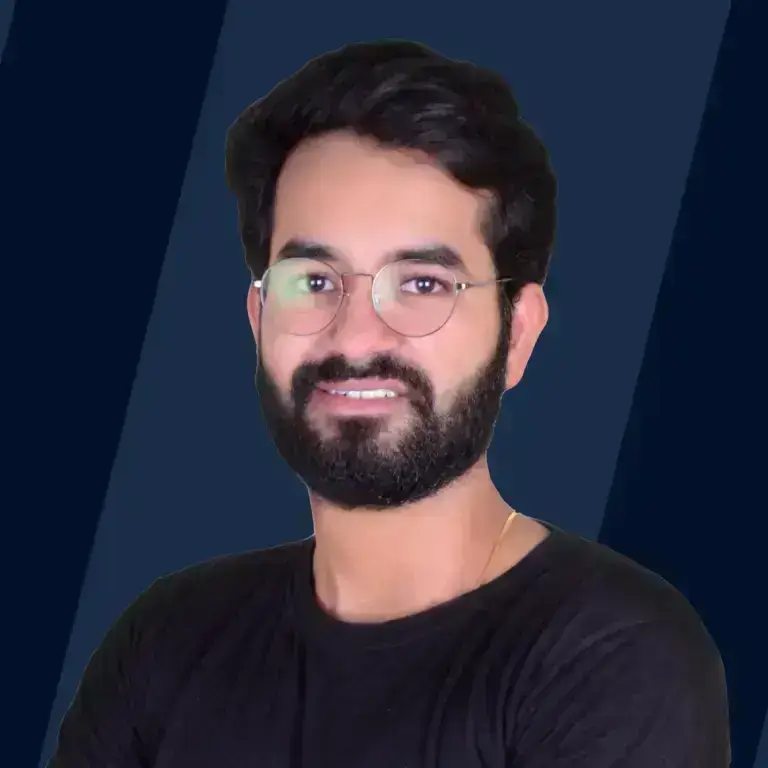
In Python, What is Returned When Evaluating [n for n in range(10) if n % 2]?
The expression [n for n in range(10) if n % 2] is an example of list comprehension. We use a for loop and an if statement together in this expression. If the if conditional holds true, the value of n will be stored in a list.
After evaluating the expression [n for n in range(10) if n % 2], we get the following output:
List comprehension helps us avoid long codes. That is why we got a list of odd integers just by writing one line of code. To learn more about list comprehensions, refer to this article.
Expanding the List Comprehension Into a for Loop
Let us say we have the following list comprehension stored in the variable new_list:
Output:
After using list comprehension, we got a list consisting of odd integers up to 10.
We can expand the above list comprehension expression into a for loop to understand how we got the above output. After expanding the list comprehension into a for loop, the code will be:
Output:
As we can see in the above code, we created an empty list named new_list and created a for loop to iterate over integers from 0 to 9. Then, we wrote a conditional statement. If the conditional holds true, the integer n will be appended to the list new_list.
List comprehensions help us avoid writing all these lines of code. Instead of writing the above 7 lines of code, we could have done all this work in a single line of code.
Explanation Of The Output
Let us now understand how did we get the output [1, 3, 5, 7, 9] for the list comprehension [n for n in range(10) if n % 2]. Following are the steps explaining the working of the program:
- We declared a variable n.
- The variable n is in the range(10) inside the for loop. So, the value of n will range from 0 to 9. In other words, n will take the values 0, 1, 2, 3, 4, 5, 6, 7, 8, and 9.
- An if conditional is applied in the for loop. The conditional checks if the value of n is divisible by 2.
- If n is divisible by 2, the remainder will be 0. Therefore, the conditional will be False since the boolean value of 0 is False. Hence, n will not be appended to the list.
- If n is not divisible by 2, the remainder will be 1. Therefore, the conditional will be True. Hence, the variable n will get appended to the list.
- Finally, after the completion of the for loop, we will get a list of odd integers.
Learn More
Conclusion
- List comprehension in Python provides a short syntax to create new lists from existing lists.
- The output of the expression [n for n in range(10) if n % 2]* is [1, 3, 5, 7, 9].