What is include iostream in C++?
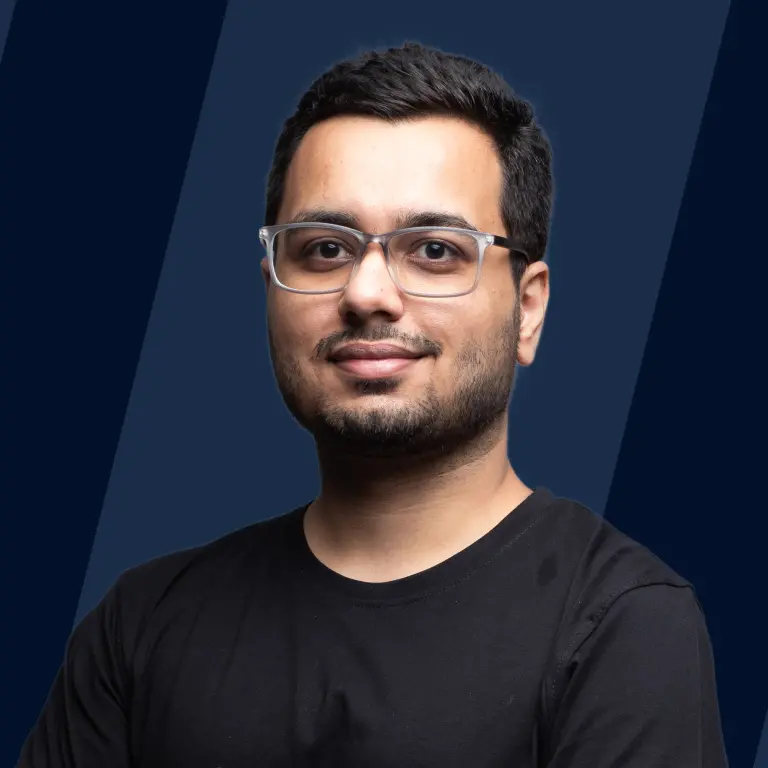
iostream stands for standard input-output stream. #include iostream declares objects that control reading from and writing to the standard streams. In other words, the iostream library is an object-oriented library that provides input and output functionality using streams.
A stream is a sequence of bytes. You can think of it as an abstraction representing a device. You can perform I/O operations on the device via this abstraction. You must include iostream header file to input and output from a C++ program.
Syntax
#include iostream provides the most used standard input and output streams, cin and cout. The syntax for using them is as follows:
1. Standard Output Stream -- cout
- It is an instance of the ostream class.
- It produces output on the standard output device, i.e., the display screen.
- We need to use the stream insertion operator << to insert data into the standard output stream cout, which has to be displayed on the screen.
Syntax:
OR
This way of using multiple stream insertion operators with a single cout is called cascading. It helps print multiple variables adjacent to each other on the same line.
What if you want to print on separate lines and cascade simultaneously? You can do this in two ways, and both of these can be used with string literals also:
- Using \n, the new line character
Syntax:
It will print variable1's value and print a new line. Finally, it prints the variable2's value on a new line.
- Using endl, a manipulator
Syntax:
A manipulator in C++ changes the behavior of an input or output stream. endl manipulator is a part of the std namespace and can also be used to insert a new line.
Check this Scaler article for the difference between endl and \n.
OR
2. Standard Input Stream -- cin
- It is an instance of the istream class.
- It reads input from the standard input device, i.e., the keyboard.
- We need to use the stream extraction operator >> to extract data entered using the keyboard.
Syntax:
OR
Cascading can also be done with cin to extract multiple variables.
Example
1. C++ Program using cout
Output:
Explanation: The above code uses cout, the ostream object connected to the display screen. The stream insertion operator <<is present before the variable name inserts its value into the screen. It is followed by another <<, which prints an escape sequence for shifting the cursor to the new line. You can also use cout to output multiple things in a single line. The final cout statement utilizes a string literal and starts inserting the value from a new line.
2. C++ Program using cin
Output:
Explanation: The above program uses cin and cout for I/O operations. But the main focus here is to understand cin; it is the istream object connected to an input device (usually the keyboard). After prompting the user to enter the first number, the number input by the user is extracted from cin. The stream extraction operator >> performs this extraction. After successful data extraction, the value is stored in the variable after the operator, i.e., a. The working is similar for extracting the second integer variable b. It finally prints the sum of the given numbers.
How Works in C++?
The most basic functionality any C++ program provides is the ability to take input from the user via keyboard and display output on the screen. You must understand input and output streams to understand how this data flow works through the objects defined in #include iostream.
1. Input Stream
- It involves the flow of bytes from the device to the main memory. This process is known as input.
- An input stream object (like cin) is a source of bytes.
- The most important input stream classes are istream, ifstream, and istringstream.
2. Output Stream
- It involves the flow of bytes from the main memory to the device. This process is known as output. It is opposite to how the input stream works.
- An output stream object (like cout) is a destination for bytes.
- The most important output stream classes are ostream, ofstream, and ostringstream.
Global Stream Objects of C++
C++ has several pre-defined stream classes related to file and I/O handling. The objects of stream classes are called stream objects. And the iostream library provides various objects globally available for a program. These globally available objects are called global stream objects.
#include iostream declares eight global stream objects that fall into the following two groups:
1. Narrow Characters
- These are byte-oriented.
- The objects use the char type internally that can take 256 values and corresponds to some entry in the ASCII table.
- They occupy lesser memory ( 8-bits ).
- The objects falling in this group are cin, cout, cerr, and clog.
a. cin
- It is an object of the istream class.
- It controls data extraction from the standard input stream as a byte stream, i.e., for reading user input.
Return value: istream object
Syntax:
b. cout
- It is an object of the ostream class.
- It controls data insertion to the standard output stream as a byte stream, i.e., printing output.
Return value: ostream object
Syntax:
OR
c. cerr
- It is an object of the ostream class.
- It is an unbuffered standard error output stream used to display or write the error messages.
Return value: ostream object
Syntax:
d. clog
- It is an object of the ostream class.
- It is a buffered standard error output stream mainly used for logging.
Return value: ostream object
Syntax:
2. Wide Characters
- These are wide-oriented.
- The objects use the wchar_t type internally, which can take much larger values than the char type and corresponds to UNICODE values. It supports international language symbols.
- They occupy more memory than narrow characters (2 or 4 bytes depending on the compiler used).
- The objects falling in this group are wcin, wcout, wcerr, and wclog.
a. wcin
- It is an object of wistream class.
- It controls input extraction, similar to cin, but uses a wide stream.
Return value: wistream object
Syntax:
b. wcout
- It is an object of wostream class.
- It controls data insertion, similar to cout, but uses a wide stream.
Return value: wostream object
Syntax:
OR
A wide string literal is always prefixed with an "L".
c. wcerr
- It is an object of the wostream class.
- It is an unbuffered standard error output stream, similar to cerr, but uses a wide stream.
Return value: wostream object
Syntax:
d. wclog
- It is an object of wostream class.
- It is a buffered standard error output stream, similar to clog, but uses a wide stream.
Return value: wostream object
Syntax:
More Examples
1. Using Standard
Explanation: By default, in standard mode, #include iostream gives you standard iostreams. The standard form of header names does not end with ".h" and provides standard headers with all the declarations in the namespace std.
2. Using Classic
Explanation: The header names in classic mode end with ".h". Such #include iostream headers put their declarations in the global namespace. They are now deprecated and no longer used.
3. Forward Declaration with Classic
Explanation: The classic iostreams allow you to write forward declarations for iostream classes. It is done to avoid the include iostream headers.
4. Forward Declaration with Standard
Explanation: You cannot use the classic names, like istream, ifstream, and others, as the class names in standard iostreams for forward declarations. It is because they are the typedefs referring to specializations of template classes. You can solve this by including the standard header iosfwd.
5. Code for Both Classic and Standard iostreams
Explanation: The idea here is to include the full headers instead of using forward declarations. The Sun C++ compiler's older versions (probably no longer available) can compile the above code successfully.
Why should We Use Instead of the Traditional ?
C++ I/O with << and >> unlocks a rich set of features. The following reasons make it more practical to #include iostream in a C++ program:
1. Increased Type-Safety
With include iostream, the object type is statically determined by the compiler used while performing I/O operations. This is in contrast to using % to determine types dynamically.
2. Reduced Errors
With C++ I/O features, you no longer need redundant % tokens to be consistent with the object type being I/O'd. The removal of redundancy leads to reduced errors.
3. Extensibility
You can easily input and output the user-defined types without breaking the existing code.
4. Inheritability
The #include iostream gives you real classes like std::ostream and std::istream. You can inherit them to create user-defined types and corresponding objects having exact characteristics like streams. It allows the programmer to manipulate the logic according to their needs. This concept differs from using cstdio's FILE*.
Learn More
You can check some basic programs in C++ that use #include iostream here.
To learn about standard header files, check this.
Conclusion
- #include iostream provides the basic I/O functionality in C++. All I/O operations make use of streams.
- The stream is the central concept of the iostream classes. A stream object is a smart file that acts as a source and destination for bytes.
- The most used iostream class objects are cin and cout, which use standard input and output streams.
- Other global stream objects given by include iostream are classified as Narrow and Wide character streams.
- The narrow character uses char as the data type while the wide-character uses wchar_t.
- Narrow character includes cin, cout, cerr, and clog, while wide character consists of wcin, wcout, wcerr, and wclog.
- The cout, cerr, clog, and their wide counterparts are instances of ostream and wostream, respectively.
- The cin and wcin are instances of istream and wistream, respectively.
- The cerr is unbuffered while clog is buffered and more efficient. Therefore, cerr is used in writing critical errors, while clog is mostly used for logging purposes. The same is true for wcerr and wclog.
- #include iostream should be preferred over cstdio because it is more type-safe, reduces errors, inheritable and extensible.