Increment and Decrement Operators in Python
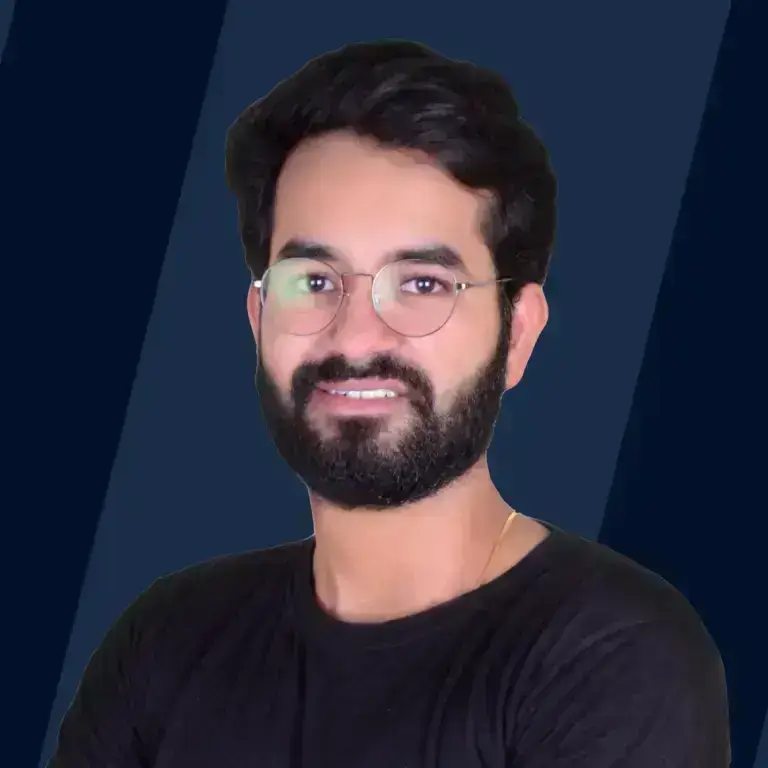
Overview
Discover the power of Python's increment function, a concise and efficient approach to increasing values in your code. In Python, the +=1 syntax neatly increments numerical variables, speeding your routines. This straightforward technique improves readability by making your code more expressive and Pythonic. Whether tallying iterations or tracking progress, the increment technique makes arithmetic operations easier, promoting clean and efficient code. Embrace simplicity with Python's incremental prowess, a small but effective method for smoother, more readable code. Easily improve your coding experience with this essential Python feature.
Python Increment Operator (+=)
In Python, operators are like the tools you use to perform actions on values or variables. They're the symbols or words that help you do things like adding numbers, comparing values, or combining strings. For example, the plus sign (+) is an operator for addition, and the double equals sign (==) is an operator for checking if two things are equal. So, operators are essentially the building blocks that let you manipulate and work with data in Python.
This Python language which is known for its readability and adaptability, includes several operators to help simplify code and increase productivity. Among these, the Increment Operator (+=) is a clear and effective tool for altering variables. In this book, we will dig into the complexities of the Python Increment Operator, explaining its function and providing simple examples to demonstrate its practical usage.
The Increment Operator, abbreviated as +=, is a compound assignment operator used to add the value on the right side to the variable on the left. Simply said, it gives a straightforward way to increase the value of a variable by a specific amount.
Basic Syntax:
Here, variable is the target variable whose value you wish to raise, and value is the amount by which the variable will be increased.
Consider this scenario: you're keeping track of the number of tasks performed in a project. You could have the variable tasks_completed set to 5. When a new job is done, just use the Increment Operator to update this variable:
In this example, the value of tasks_completed is increased by the value of new_task_count using the Increment Operator. The output will be:
Advantages of Using the Increment Operator
-
Conciseness:
The += operator provides a concise and clear way to express the intent of incrementing a variable.
-
Readability:
Code that uses the Increment Operator is often more readable and easier to understand than longer, equivalent expressions.
Common Pitfalls and Best Practices
While the Increment Operator is a handy tool, it's essential to use it judiciously to avoid unintended consequences. Here are some best practices:
-
Variable Initialization:
Ensure that the variable you are incrementing is properly initialized before using the Increment Operator.
-
Clear Variable Names:
Use meaningful variable names to increase your code readability and make the intent of the incrementation clear.
-
Avoid Chaining:
While it's possible to chain the increment operations (e.g., x += 1 += 2), it can lead to confusion. Stick to clear and straightforward expressions.
The Python Increment Operator (+=) is a valuable addition to a programmer's toolkit, providing a simple method for incrementing variables. Accepting its simplicity and strength enables developers to write more expressive and efficient code. Whether you're tracking events, counting occurrences, or iterating over lists, the Increment Operator may help you enhance code readability and conciseness.
Python Decrement Operator (-=)
In Python programming, efficiency and simplicity often coexist. The decrement operator (-=) is an effective tool for representing this concept. In this research, we'll look at the complexity of the Python Decrement Operator, determining its essence and demonstrating its practical application using simple examples.
The Python Decrement Operator, represented by the symbol -=, is a modest but powerful tool that enables developers to lower the value of a variable by a specified amount. It gives a simple and understandable mechanism for removing a certain value from an existing variable. This operator is quite beneficial for simplifying and improving the expressiveness of your code.
The fundamental syntax for the Python Decrement Operator is as follows:
Here, variable_name is the target variable, and decrement_value is the amount by which the variable will be reduced.
Let's use a basic example to teach the Python Decrement Operator. Consider an instance where the variable counter is initialized to 10 and you wish to decrease it by 2.:
In this example, the counter variable is decreased by 2 using the -= operator. When you print the counter, the output will be 8. This successive operation replaces the traditional approach of continuously assigning the updated value, making the code more concise and readable.
Benefits of Using the Python Decrement Operator
-
Conciseness and Readability:
The -= operator reduces the decrement action into a single line, improving the overall readability of your code. This is very useful when dealing with loops and other repeated patterns.
-
Expressiveness:
Using the decrement operator indicates the desire to reduce a variable more straightforwardly and naturally. This clarity not only enhances your understanding of the code but also facilitates collaboration with other engineers.
-
Code Optimisation:
The Python Decrement Operator aids in code optimization by eliminating the need for unneeded lines. This results in a more simple and efficient codebase.
In Python programming, simplicity is frequently associated with elegance. The Python Decrement Operator, denoted by -=, exemplifies this concept by offering a simple and expressive technique for reducing variables. Incorporating this operator into your code increases the readability and overall efficiency of your Python scripts.
To recap, the Python Decrement Operator is an excellent addition to your coding toolkit, allowing you to create more efficient and elegant code while maintaining simplicity.
Examples
Python, a versatile and powerful programming language, has several methods for manipulating numerical numbers. Among these actions, incrementing and decrementing are critical for managing loops, variables, and functions.
Learning about increment and decrement operators in Python is useful because they provide a quick and concise way to increase or decrease the value of a variable by 1. Instead of using a longer syntax like variable = variable + 1 for incrementing or variable = variable - 1 for decrementing, you can use the shorter variable += 1 and variable -= 1 respectively.
This not only makes your code more readable and compact but also saves you time and effort. Increment and decrement operations are common in programming, especially in loops and counting scenarios. So, understanding and using these operators can make your code more efficient and expressive.
In this section, we'll look at a variety of examples to show the versatility of Python's increment and decrement operators, ensuring that you understand these fundamental concepts.
Decrement and Increment Operator With for Loop
In Python, the for loop is an efficient loop for iterating over a sequence of elements. When coupled with increment and decrement operators, it becomes a dynamic mechanism for altering numeric values. Consider the following example:
In this example, we can see that the loop starts at 5 and declines by 1 until it reaches 1, demonstrating the decrement operator's role in influencing loop behaviour.
Increasing a Number with Assignment
When incrementing a variable in Python via assignment, the language's simplicity is evident. Look at this little example.
The variable count is increased by one using the +=. The assignment operator shows a concise and expressive way to execute increments in Python.
Increment a Number Using the Operator
Python's increment operator (+=) may be used directly to a variable, providing a more straightforward alternative to standard assignment. Consider this example:
In this example we saw the value of number is increased by 3, demonstrating the increment operator's usefulness.
Increment a Number Using the Function
Python's adaptability extends to function-based incrementation, allowing for more complex applications. Here's an example using a custom function:
By utilizing a function, you gain modularity and reusability, enhancing the maintainability of your code.
Increment a Number Implicitly
Python's dynamic nature allows for implicit incrementation in certain contexts. Consider the following example using a for loop:
A list comprehension implicitly increases each member by one, exemplifying Python's clean and simple programming approach.
Understanding the increment and decrement strategies in Python is essential for a successful programming career. The offered examples demonstrate Python's numerical operation versatility and simplicity. Understanding the principles of loops, variables, and functions will enable you to create more efficient and expressive Python programs.
Conclusion
- Python has a different syntax for increment and decrement operations. To raise the value of a variable, use the += operator; to decrease it, use the -= operator. This concise syntax enhances Python's readability and simplicity.
- Python's in-place increment and decrement capabilities are intriguing. Instead of assigning a new value to a variable, these operations modify the current variable directly. This is a valuable shortcut for working with iterative processes or dynamic programming settings.
- Python's adaptability includes data types that may be increased and decremented. Whether dealing with integers, floating-point numbers, or other numerical types, the same concise syntax may be utilized everywhere. This flexibility makes code simpler and easier to comprehend.
- Python separates itself from other programming languages by removing the pre- and post-increment/decrement operators. The language encourages consistent variable change through the use of the += and -= operators.
- Python's support for functional programming is complemented by its increment and decrement operations. These operations align with functional programming principles by enabling developers to modify variables in a manner consistent with immutability. This adds a functional touch to Python's versatile programming paradigm.
- The simplicity of increment and decrement operations becomes evident when employed in loop structures. Python's for and while loops can seamlessly integrate these operations, enhancing the elegance and readability of iterative code. This contributes to Python's reputation as a language that prioritizes code readability and developer convenience.