InetAddress in Java
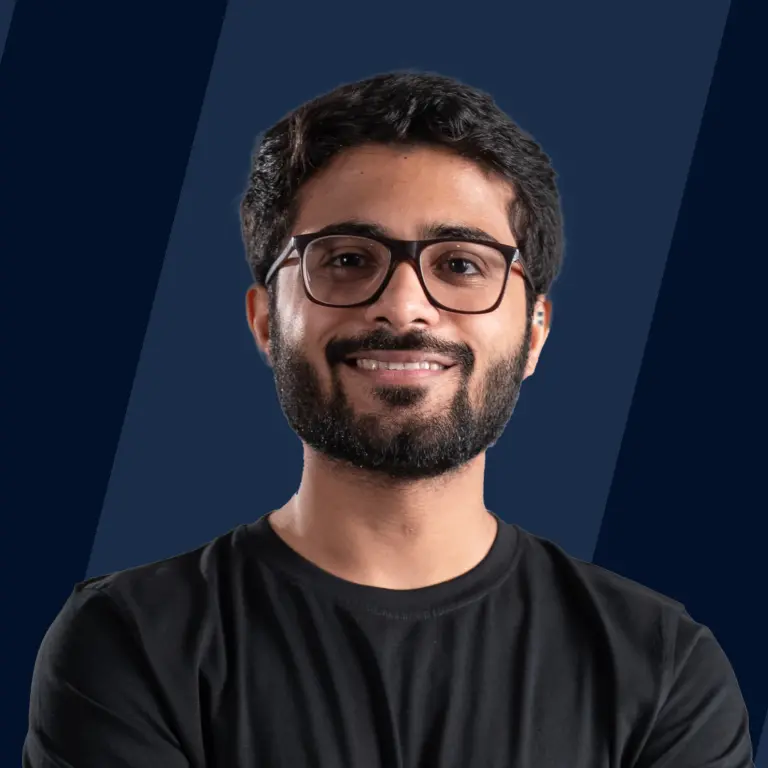
Overview
InetAddress in Java represents an IP address. java.net.InetAddress class provides methods to obtain the IP address of any hostname (For example, www.example.com) In this article, we'll learn more about InetAddress in Java
Introduction to inetaddress in Java
IP addresses are represented by 32 or 128-bit unsigned numbers. InetAddress is used to handle both IPv4 and IPv6 addresses It can be represented with its host name using an instance of InetAddress.
There are two types of addresses: Unicast: It's an identifier for a single interface Multicast: It's an identifier for a set of interface
InetAddress has a cache mechanism for storing successful and unsuccessful host name resolutions.
IP Address
A numerical representation of an IP address can be used to identify a resource on the network.
IP (Internet Protocol) governs the format of data sent via the internet or local network.
It is a string of numbers separated by periods. They are expressed as a set of 4 numbers. It's a unique address that identifies a device on the internet or a local network. Each number in the set can range from 0 to 255. So, the full IP addressing range goes from 0.0.0.0 to 255.255.255.255.
Networks combine IP with TCP (Transmission Control Protocol) Among the destination and source, a virtual bridge is built
There are two versions of IP address:
1. IPv4 It's a primary internet protocol and is the first version of IP deployed for production in the ARPANET in 1983. It differentiates devices using network addressing schemes as a widely used IP version.
A 32-bit addressing scheme is used to store a 232 address
Let's look at some of the features of IPv4:
- IPv4 is a connectionless protocol that utilizes less memory
- Addresses can be remembered easily with the class-based addressing scheme.
- It offers various other features like video conferencing and libraries
2. IPv6 It's the latest version of Internet protocol which aims to fulfill the need for more internet addresses. It caters to the problems present in IPv4 and provides 128-bit address space to form 340 undecillion unique IP addresses to form a network. It's also known as IPng aka Internet Protocol next generation.
Let's look at some of the features of IPv6:
- It has both stateful and stateless configurations.
- Quality of service (QoS) support is provided
- Hierarchical addressing and routing infrastructure are done
TCP/IP Protocol
TCP/IP protocol is a communication protocol model which aims at connecting devices with the help of the internet over a network TCP/IP protocol is used for addressing, transmitting, routing, and receiving packets over the internet
Two main protocols are used in this communication model, those are:
- TCP: Transmission Control Protocol helps create a communication channel across a network. It's used for transmitting packets at both the sender's and receiver's end
- IP: Internet Protocol is used to provide the address of the nodes connected to the internet. It uses a gateway computer to check the validity of an IP address and for checking whether the forwarded message is correct or not
InetAddress – Factory Methods (Table format)
Using the Inetaddress class, both the numerical IP address and domain name for the address can be condensed. Since InetAddress class lacks public constructors, it cannot create new objects directly. This is where factory methods come in; they are static methods in a class that return an object of that class
Factory methods: are static methods in a class which is used to return an instance of that class.
There are five factory methods available in the InetAddress class –
Method | Description |
---|---|
public static InetAddress getByName(String host) | An InetAddress object is created based on the provided hostname |
public static InetAddress getByAddress(byte[] addr) | It returns an InetAddress object from a byte array of the raw IP address |
public static getAllByName(String host) | It returns an array of InetAddress objects from the specified hostname, as a hostname can be associated with several IP addresses |
public static InetAddress getLocalHost() | It returns the instance of InetAddress containing the local hostname and address. |
Some more methods of InetAddress class:
Method | Description |
---|---|
public byte[] getAddress() | It returns the raw IP address of this InetAddress object as an array |
public String getHostAddress() | It returns IP address in textual form |
public boolean isAnyLocalAddress() | It returns true if this address represents a local address |
public boolean isLinkLocalAddress() | It returns true if this address is a link-local address |
public boolean isLoopbackAddress() | It returns true if this address is a loopback address. |
public boolean isMCGlobal() | It is used to check whether the multicast address has a global scope or not |
public boolean isMCLinkLocal() | It is used to check the utility routine if the multicast address has a link scope or not |
public boolean isMCNodeLocal() | It is used to check the utility routine if the multicast address has node scope or not. |
public boolean isMCOrgLocal() | It is used to check the utility routine if the multicast address has organization scope or not. |
public boolean isMCSiteLocal() | It returns true if this multicast address has site scope. |
public boolean isMulticastAddress() | It returns true if this address is an IP multicast address |
public boolean isSiteLocalAddress() | It returns true if this address is a site-local address |
public int hashCode() | It returns the hashcode associated with this address object |
public boolean equals(Object obj) | It returns true if this IP address is the same as that of the object specified |
Let's see an example of implementing these above-mentioned factory methods:
Output
Here we've first used InetAddress.getLocalHost() which gets and prints the InetAddress of Local Host as InetAddress of Local Host : localhost/127.0.0.1
We've then used InetAddress.getByName("45.22.30.39"); which gets and prints InetAddress of Host as /45.22.30.39
In order to get and print ALL InetAddresses of Named Host we've used InetAddress.getAllByName("172.19.25.29");
InetAddress.getByAddress(IPAddress); is used to get and print InetAddresses of the host with specified IP Address, to get InetAddresses of Host with specified IP Address along with the hostname we can use InetAddress.getByAddress( "www.scaler.com/topics/", IPAddress2);
InetAddress — Instance Methods (Table format)
Instance methods of the InetAddress class has can be called using the object.
For the purpose of invoking this method, we first require an object to be created
We can have int, float, string, or any user-defined data type as a return type
This method does not belong to the class but to objects of the class, which is why object creating is a necessity before we can invoke this method.
These methods are stored in a single memory loaction. This pointer is passed as we call this method which is how they know which object they belong to
Since the methods are reoslved using dynamic binding at run time they can be overridden.
Instance methods of InetAddress class are –
Method | Description |
---|---|
equals(Object obj) | We compare an object against another specified object using this function |
getAddress() | The raw IP address of this InetAddress object is returned using this method in bytes. |
getCanonicalHostName() | For returning a full qualified domain of an IP address, this method is used |
getHostAddress() | To get the IP address in string form we use this method |
getHostName() | Toreturns the host name for this IP address we can use this method |
hashCode() | To get a hashcode for this IP address we can use this method |
isAnyLocalAddress() | It's a utility routinr for checking if the inetaddress is unpredictable |
isLinkLocalAddress() | This is also a utility routine for checking whether the local unicast address is not linked to the address |
isLoopbackAddress() | for checking if the inetaddress represents a loopback address, this method is used |
isMCGlobal() | It's also a routine check for checking if the address has a multicast address of global scope. |
isMCLinkLocal() | It's another utility routine check to see if this address has a multicast address of link-local scope. |
isMCNodeLocal() | It's a routine check to see if the multicast address has node scope. |
isMCOrgLocal() | we can use this method to check if the multicast address has an organization scope |
isMCSiteLocal() | Used to check if the multicast address has site scope. |
isMulticastAddress() | Checks whether the site has multiple servers. |
isReachable(int timeout) | To test whether that address is reachable, this method is used |
isReachable(NetworkInterface netif, int ttl, int timeout) | To tests whether that address is reachable we can use this method |
isSiteLocalAddress() | Used to check if the InetAddress is a site-local address. |
toString() | Converts and returns an IP address in string form. |
Let's now implement these methods using a Java program
Output
Here, we first use getByname() which determines the IP address of a host from the given host's name, it's then stored in address1, address2, and address3 respectively.
We then see if these addresses are equal using address1.equals(address2));
Here, since address1 is the same as address2 the output is true whereas when we compare address2 with address3 which isn't the same, we get false as an output.
We've then used get host address() to get the IP address for address1 in string form address1.getAddress() provides the raw IP address of this InetAddress object which is then returned using this method in bytes like IP Address in bytes : [B@579bb367.
We then use address1.isMulticastAddress() for checking whether the site has multiple servers which then returns false for address1
address1.getCanonicalHostName() returns a full qualified domain of an IP address
We print hashcode for this address using address1.hashCode() which comes in output as 756424231.
address1.isAnyLocalAddress() is then used as a utility routine for checking if the local unicast address is not linked to the address, we see that the program returns false for address1
Examples for the InetAddress Class in Java Get IP address of a Website
As you know we can obtain an IP address of any website using InetAddress class in Java, let's now see how we can implement it using a Java program to get the IP address of https://www.scaler.com/topics/ website.
Output:
Host name and ip address of scalar topics site is thus printed using ip.getHostName() and ip.getHostAddress()
Program to Demonstrate Methods of InetAddress Class
Let's see the actual implementation of InetAddress class methods in our Java program
Output:
By now we've seen how methods like getByAddress(), getByName(), getAllByName(), and getHostAddress() work, here in the above code we've implemented some more methods in Java for you to see how they are implemented
The isLinkLocalAddress() method checks if the local unicast address is not linked to the address which as we can see comes out as false in the output
The isLoopbackAddress() method is then used to check if the inetaddress represents a loopback address.
isMCGlobal() method returns false since the ip address does not have a multicast address of global scope. ip.isMCLinkLocal() returns false since this address does not have a multicast address of link-local scope
Similarly, ip.isMCNodeLocal() also returns false since this multicast address does not have a node scope.
ip.isMCOrgLocal() is a method to check if the multicast address has an organization scope ip.isMCSiteLocal() is Used to check if the multicast address has a site scope.
ip.isMulticastAddress() returns false checks whether the site has multiple servers ip.isSiteLocalAddress() checks if the InetAddress is a site-local address and returns false
ip.hashCode() gets a hashcode hashCode: -1404844974 for this IP address
Conclusion
- InetAddress in Java represents an IP address where methods to get an IP of any hostname can be provided by the java.net.InetAddress class.
- Numerical representation of an IP address can be used to identify a resource on the network
- TCP/IP protocol is a communication protocol model which aims at connecting devices with the help of the internet over a network
- Since InetAddress class lacks public constructors, factory methods act as static methods in a class that return an object of that class
- IP addresses are represented by 32 or 128-bit unsigned number
- IPv4 is a connectionless protocol that utilizes less memory