How to Represent Infinity in Python?
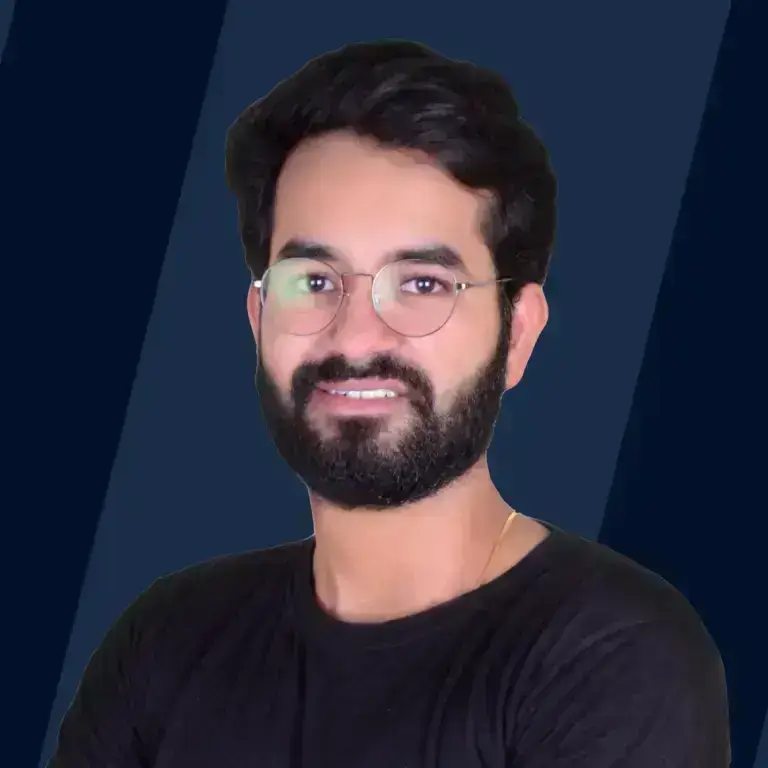
There are multiple ways to represent Infinity in Python. Some of the common ways to represent Infinity in Python are, using float(), using Python's Math Module, using Python's Decimal Module, and using Python's Numpy Library. But first, let's understand what is infinity in Python.
What is Infinity in Python?
Infinity in Python is an indeterminate number that can be either positive or negative. But can you guess where can we use such a number? Infinity in Python can be used in algorithms to compare solutions for the best answer. Consider the case where we want to find the maximum number in an array. Initially, we have to keep our answer as small as possible so that we can get our best answer. Let's understand this with an example.
Consider the array as 1,2,3,4,5 and we set our initial ans as 0. For each element of the array, we will compare the ans and the current element. And if the current element is greater than ans, we will update ans as the current element. Doing this on the above array will give us ans = 5.
The highlighted cell in the above image is our ans, this is valid for the current array. But now let's change the array to -5,-4,-3,-2,-1 Again we set the initial ans to 0, and if we try to perform the above approach ans will be equal to 0 at the end of the program.
But as you can see in the array the maximum number is -1. So the initial value of ans will decide whether we will get the correct answer or not. In the case of finding the maximum number we need our initial ans to be as small as possible, hence we can use infinity in Python to do so.
Representing Infinity as an Integer in Python
There are multiple ways of representing infinity in Python, let's understand them one by one.
Method 1 : Using float(‘inf’) and float(‘-inf’)
We can use the float() function in Python to represent the positive infinity and negative infinity.
For positive infinity, we need to pass 'inf' to the float() function and for negative infinity, we need to pass '-inf' to the float() function.
Let's see the implementation of the above method,
Output:
You can learn more about the float() function in Python here float() in Python | Python float()
Method 2 : Using Python’s Math Module
We can also use the math module to represent infinity in Python, but it only works with Python 3.5 or higher. Since infinity in Python can be both positive and negative, it is denoted by math.inf and -math.inf.
Let's see the implementation of the above method,
Output:
You can learn more about the math module in Python here Math Module in Python
Method 3: Using Python’s Decimal Module
We can also use the Decimal module to Python to represent infinity in Python. For positive infinity, we can use the Decimal('Infinity') and for negative infinity, you guessed it right we can use the Decimal('-Infinity').
Let's see the implementation of the above method,
Output:
Method 4: Using Python’s Numpy Library
Another approach to represent infinity in Python is by using the NumPy module where numpy.inf and -numpy.inf represent positive and negative infinite, respectively.
Let's see the implementation of the above method,
Output:
Mathematical Operations on Infinity in Python
Have you wondered what if we perform mathematical operations on infinity in Python? These operations can be done in two ways. Let's also understand these.
Mathematical Operations with Two Infinity in Python
Addition Operation
Output:
As you can see in the output the addition of two positive infinity is infinity, the addition of two negative infinity is -infinity, and the addition of positive and negative infinity is not a number (nan).
Subtraction Operation
Output:
As you can see in the output that the subtraction of two positive infinity is not a number (nan), the subtraction of two negative infinity is also not a number (nan), and the subtraction of positive and negative infinity is infinity.
Multiplication Operation
Output:
As you can see in the output that the multiplication of two positive infinity is infinity, the multiplication of two negative infinity is also infinity, and the multiplication of positive and negative infinity is -infinity.
Division Operation
Output:
As you can see in the output the division of two positive infinity is not a number (nan), the division of two negative infinity is also not a number (nan), and the division of positive and negative infinity is also not a number (nan).
Mathematical Operation with One Infinity in Python and One Finite Value
Addition Operation
Output:
The addition of a finite number to infinity in Python will result in infinity.
Subtraction Operation
Output:
The subtraction of a finite number from infinity in Python will result in infinity.
Multiplication Operation
Output:
The multiplication of infinity in Python with a finite number will result in infinity.
Division Operation
Output:
Division of infinity in Python with a finite number will result in infinity.
So we can conclude that if we perform the mathematical operations with one infinity in Python and one finite value, the result will be infinity.
Checking If a Number is Infinite or Not
We can use the isinf() method to check whether a number is an infinity or not. This method is present in the math library and it returns true if the provided number is infinity else it returns false. Let's see the implementation for the above.
Output:
As you can see in the output x and y are infinity in Python, while a and b are finite values.
Comparing Infinite Values to Finite Values
The idea of comparing an infinite value to a finite value is very simple. Because positive infinity is always greater than any number and negative infinity is always less than any number. Let's see the code for the above.
Output:
Here x is positive infinity and y is -infinity. And a and b are two finite values with values 999999999999 and -999999999999 respectively. As you can see in the output, x is greater than both a and b. And y is less than both a and b.
Conclusion
- Infinity in Python is an indeterminate number that can be either positive or negative.
- Infinity in Python can be represented using the float() method, using the math library, using the Decimal library, and using the numpy library.
- Mathematical operations on infinity in Python results in either infinity or not a number (nan).
- We can check whether a number is infinite or not by using the isinf() method present in the math library.
- A positive infinity is always greater than any number and negative infinity is always less than any number.