Input Stream in Java
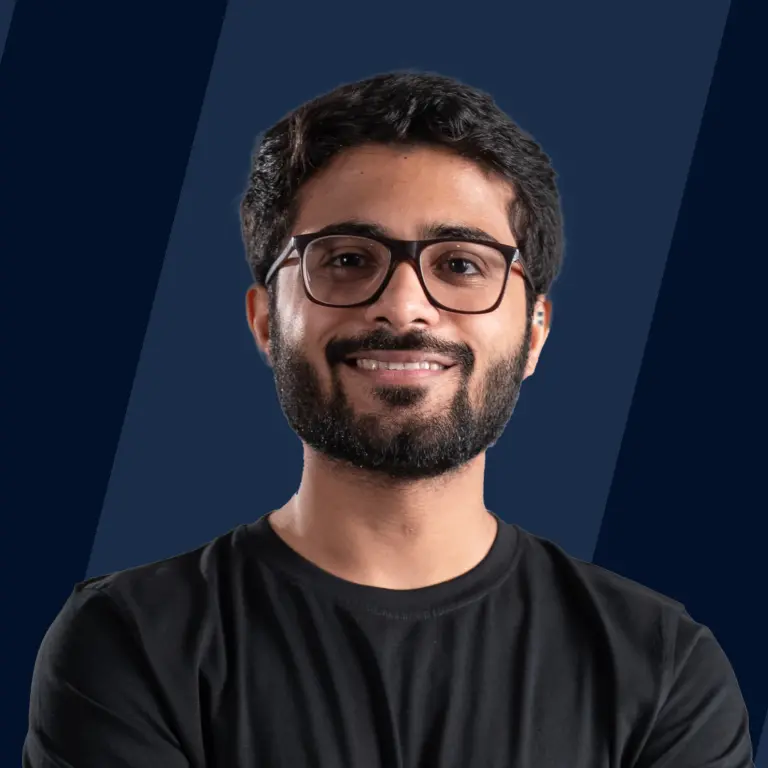
Overview
A stream represents a uniform, easy-to-use, object-oriented interface between the program and input/output devices. We can write data to a stream and read data from a stream. We write data from an input stream, the source of which can be a keyboard.
In this article, we will learn about the Input stream in java, its subclasses, methods, etc.
What is the Input Stream in Java ?
The input stream is the superclass of the I/O operation and is used for reading inputs of a user through a keyboard, file, etc. It is used to represent an input stream of bytes.
The use of stream has two advantages :
- We don’t have to worry about the details of each device because it would be handled by java behind the scenes.
- The program can work for a variety of I/O devices without any changes in the code.
The package java.io contains the classes that provide the foundation for java’s support for stream I/O.
A reset() method is used for repositioning the stream to the recently marked position.
Declaration of InputStream :
The constructor used for InputStream is :
Subclasses of Input Stream in Java
For using the functionality of InputStream, its subclasses are used, some of which are :
- FileInputStream
- ByteArrayInputStream
- ObjectInputStream
FileInputStream :
It is used for reading data in bytes from files and the class used is java.io. It extends the abstract class of the input stream called “InputStream”.To create and use the file input stream, we first need to import the package, java.io.FileInputStream.
There are two ways in which FileInputStream can be used inside a file :
-
Using a path of file :
In this, we will create an input stream that will be linked to a file by using the specified path.
For eg : -
By using an object of a file :
In this, we will create an input stream that will be linked to a file as specified by the object.
For eg :
Methods of FileInputStream :
It has different methods which can be used for implementing different functionality.
methods | description |
---|---|
read() | Used for reading bytes from a file |
available() | It is used for getting the number of available bytes that can be used. |
skip() | Used to skip or discard a specified number of bytes. |
close() | It is used to close the file input stream. |
finalize() | It ensures that the close method is called. |
getChannel() | It is used for returning the object of FileChannel linked with the input stream. |
getFD() | It is used to return the description of the file from the input stream. |
mark() | Used to mark the position in the input stream until the end of data. |
reset() | Used for returning th control to the stream. |
ByteArrayInputStream :
This class extends the "abstract class InputStream" and is used to read an array of the input streams in bytes. This InputStream is created using an array of bytes and uses an internal array for storing the data.
ObjectInputStream :
It is used for reading the objects that were previously written by ObjectOutputStream of the java.io package. It extends the abstract class InputStream.
Create an InputStream in Java
To create an InputStream, we need to convert the String object to an InputStream object by using the class ByteArrayInputStream. The reason for using this is that it has an internal buffer present that can be used to read bytes from the stream.
Here’s a step-wise procedure to implement InputStream in java :
- Getting the bytes of the string.
- Creating a new ByteArrayInputStream using the bytes of the string.
- Assigning the object of ByteArrayInputStream to the variable InputStream.
- The byte that is read is stored in the buffer.
- Printing the InputStream.
For implementing it, here is a code :
Java Program to Convert String to InputStream using ByteArrayInputStream.
Output :
Methods of Input Stream in Java
read() :
java.io.InputStream.read() :
It is used for reading the next byte of the data from InputStream. The value of the byte is in the range of 0 to 255. If no byte is found and has reached the end of the file then it returns -1.
The syntax for read() :
read(byte[] array) :
Java.io.InputStream.read(byte[] arg) :
It is used for a reading number of bytes of array.length from the input stream to the array of buffer which is arg. If the length of the arg is zero then nothing is read and it returns zero.
The syntax for read(byte[] array) :
available() :
java.io.FileInputStream.available() :
This method is used for returning the number of available bytes from the input stream.
The syntax for available() :
mark() :
Java.io.InputStream.mark(int arg) :
It marks the current position of the input stream and sets the read limit. The number of bytes that can be read before the position where it is marked becomes invalid.
The syntax of mark() :
reset() :
Java.io.InputStream.reset() :
It is invoked by the method mark() and it is used for repositioning the stream of input to the marked position.
The syntax of reset() :
markSupported() :
Java.io.InputStream.markSupported() :
This method is used to test if the input stream supports the method mark and reset in java. If not, it returns false.
The syntax of markSupported() :
skip() :
Java.io.InputStream.skip(long arg) :
It is used to discard and skip arg bytes of the input stream.
The syntax of skip() :
close() :
java.io.InputStream.close() :
It is used for closing the input stream and releasing resources used by InputStream to the garbage collector.
The syntax of close() :
Example
The example given below uses all the methods discussed in this blog. Java program which depicts the working of InputStream method by using different methods like mark(), read(), skip(), markSupported(), close(), reset().
In this example, make a file named, file.txt and put some text in that file. The file should be in the same directory as that of a program.
For instance the file file.txt has the following text :
Output :
Conclusion
I hope you liked this article. Here are a few key takeaways from this article :
- A stream represents a uniform, easy-to-use, object-oriented interface between the program and input/output devices.
- The input stream is the superclass of the I/O operation and is used for reading inputs of a user through a keyboard, file, etc. It is used to represent an input stream of bytes.
- Subclasses of Input Stream in Java :
- FileInputStream
- ByteArrayInputStream
- ObjectInputStream
- Create an InputStream in Java :
- Getting the bytes of the string
- Creating a new ByteArrayInputStream using the bytes of the string
- Assigning the object of ByteArrayInputStream to the variable InputStream
- The byte that is read is stored in the buffer
- Printing the InputStream
- Methods of Input Stream in Java :
- read()
- read(byte[] array)
- available()
- mark()
- reset()
- markSupported()
- skips()
- close()