Program for Insertion Sort in Python
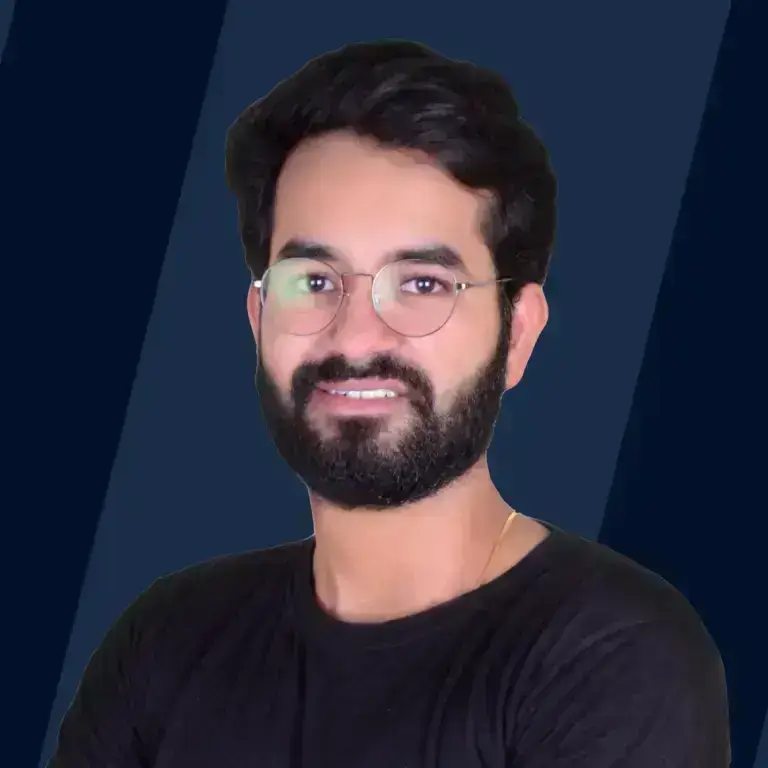
Overview
Insertion sort is a simple, stable sorting technique that builds the sorted array one item at a time. The array is split into two parts, where the first part of the array is sorted. At every iteration, the first element of the unsorted part is picked and placed in its correct position in the sorted part. It can be related to sorting a deck of cards in your hand.
Insertion Sort in Python
Insertion sort is a simple sorting algorithm that divides the array into two parts, a sorted part, and an unsorted part. At each iteration, an element from the unsorted array is placed at its correct position in the sorted array.
Properties of Insertion Sort :
- In-place :
Insertion sort is an in-place algorithm. This means that it does not require any additional space for sorting. - Stable :
Insertion sort is a stable sorting algorithm. Suppose we are given an array , and there are two elements and such that and . In a stable sort, the element will be placed before the element in the final sorted array.
Algorithm for Insertion Sort in Python
Problem Statement
Given an array containing integers. The problem is to sort the array in non-decreasing order using Insertion Sort in Python.
Example
Input :
Output :
Intuition
Insertion sort can be visualized as sorting cards in our hands. Suppose we have 5 playing cards with numbers written on them as .
Now we assume that the first card is at its correct location and we are left with four unsorted cards .
Now we pick the first unsorted card 4 and place it at its correct location, which is before the card with the number 10.
We continue the same process for each element in the unsorted part. So we pick the card numbered 1, then the card numbered 5, and so on. Each time we place it in its correct position.
Observe that we are building the sorted sequence of cards from the front. In this way, we will end up with the sorted sequence.
Algorithm
Suppose we are given an array A of size N.
- We consider that the first element of the array A is in its correct position.
- We iterate for all the elements from to . We will place the element at its correct position in the prefix .
- We know that the elements from to are sorted and is the first unsorted element. Let us initialize to a variable . So to find the correct position of , we compare it with all the elements from to .
- Case - 1 :
. In this case, we know the element will be placed before as is greater, hence we shift this element to . - Case - 2 :
. In this case, we have found the correct position of in the sequence. We insert the at location .
- Case - 1 :
- From the above step, we guarantee that the array from to is sorted and we move to the next element.
In this way, we sort array A. Let us understand this using an example.
Example :
- For , .
- For , , we can see that , so we shift to . .
- Now for , we break this loop at assign , so and .
- For , .
- For , and
- For , and
- For , we assign , so
- For , .
- For , and
- For , and
- For , and
- For , we assign , so
- For and .
- For , and
- For , and so break the loop. We assign so .
In this way, we perform insertion sort on the unsorted array A.
Implementation
Output :
Complexity Analysis of Insertion Sort
Time Complexity
In the implementation, we iterate for every element in the input array A and we find its correct position in the sorted prefix. To find the correct position, we again iterate over the prefix of the array A. The worst case occurs when we are given an array sorted in descending order. In this case, the second element will be compared with the 1 element, the third element will be compared with the 2 elements, and so on. So, the total number of comparisons will be equal to which is , so the time complexity is , where is the size of the input array.
Time Complexity :
Space Complexity
In the implementation, we use a constant number of variables, so the space complexity is , where N is the size of the input array.
Space Complexity :
Application of Python Insertion Sort
- Insertion sort can be used when the number of elements is very small. This is because insertion sort requires less memory and the runtime overhead is very small when compared to Quick sort, Bubble sort, and Merge sort. The constant factor in the overall running time is tiny in the case of insertion sort.
- If the array is partially sorted, then insertion sort can be used to sort the array quickly.
- If it is known apriori that each element, in the input array, is at most K places away from its actual position in the sorted array, then insertion sort can be useful as it gives the time complexity of where N is the number of elements in the input array.
Related Programs in Python
Conclusion
- Insertion Sort is a simple, stable sort that divides the array into two parts. Each element in the unsorted part is put into its right location in the sorted part.
- Insertion sort takes time and space.
- Insertion sort can be used when the number of elements is small. It is fast as the constant factor is small.