Instance Variable in Java
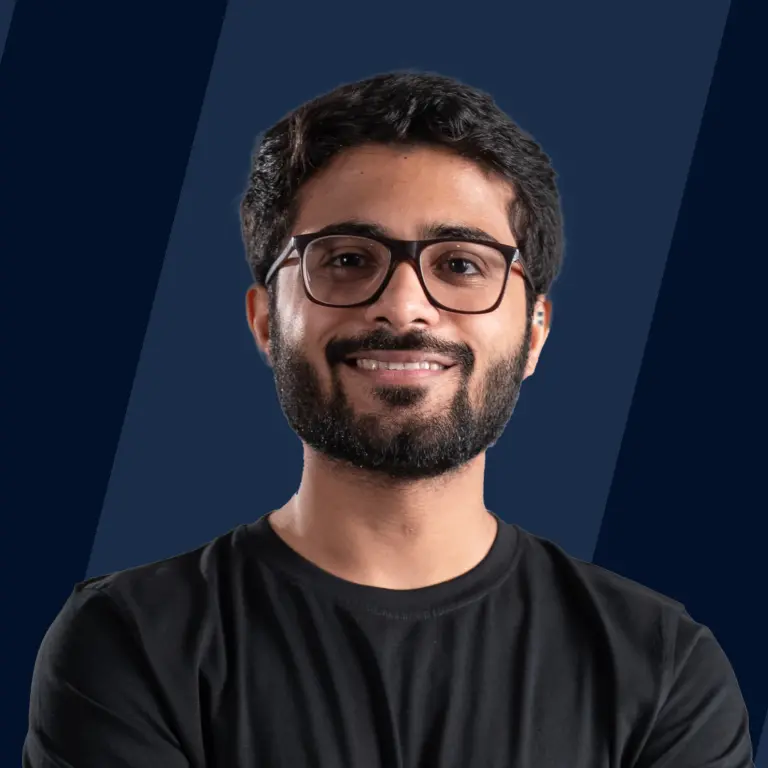
Overview
Instance variables are declared in the class, but outside of the constructors, methods, or blocks of the particular class. Instance variables can be changed by any method in the class, and are not accessible from outside the class. They are used to represent the state of an object and are stored in the heap section of the memory.
What is Instance Variable in Java?
Let's understand the instance variable using an example. Suppose we have a class named "Person", and every person has his characteristics like weight, color, age, etc. Now how can we define these characteristics in the class? The answer is we can take the help of the instance variables.
Instance variables are defined as outside of any method declaration. They are used to represent the state of the object, and every object of the class has its copy of the instance members.
Syntax
Instance variables can be of any data type like int, float, double etc, also it can be of user-defined data types. Let's understand the syntax of the instance variables.
Syntax of declaration of instance variables:
The datatypename is the name of the data type of which we want to create an instance variable. Let's suppose we want to store the name of a particular person, we can use String data type for the purpose.
Note : The instance variables must be declared outside the methods of the class or any kind of block(Scope) in the class.
Example
Let's understand how to declare instance variables in the class.
Rules for Instance Variable in Java
- The instance variables cannot be marked as static. Refer to this Static variable in java.
- The instance variables must be declared outside the class methods , and any kind of scope.
- The instance variables can be marked by the access specifiers.
- Instance variables cannot be marked by the abstract keyword(abstract keyword only used for the methods).
- Instance variables cannot be marked by the strictf keyword.
Features of an Instance Variable
- Access Modifiers can be used as a prefix, during the declaration of the instance variables.
- Instance variables can be accessed in any method of the class except the static method.
- Instance variables can be declared as final but not static.
- The instance Variable can be used only by creating objects only.
- Every object of the class has its own copy of Instance variables.
- Instance variables are created during the creation of an object in the heap memory (Heap is part of the memory that is allocated by the JVM that is shared among all the threads of the Java program).
Accessing Instance Variables
We can access the instance variable using two ways.
- Using "this" keyword.
- Using an object of the class.
Let's understand both methods one by one.
1. Using this Keyword
If we want to access the instance members in the methods of the class, we can access them using the "this" keyword. This keyword referred to the current object of the class.
Let's understand how to access the instance variable using the "this" keyword.
In the below program, we are creating a class "Person" that is containing two instance variables , and one instance method that is used to print the value of the instance variable name.
Output :
The "Aayush" is printed because we initialize the value of the instance variable in the constructor of the class using the "this" keyword.
2. Using an Object of the Class
We can access or initialize the instance variable using the class objects. We know very well instance variables are created in the heap section of memory when the objects of the class are declared using the new keyword that's why we object of the class has the right to access them.
Let's understand how to access the instance variables using an example.
Output :
The Person's name is initialized using the class object name.
Default Values of Instance Variables
When the instance variables are not initialized with the suitable values, the default value is initialized of the respective data types . For example in the above example, if we want to print the value of the age, the 0 will be printed because the age is the type of int, and the default value of the int is 0.
The default value of all datatypes that can be used for declaring the instance variables are given below :
Datatypes | DefaultValue |
---|---|
int | 0 |
boolean | false |
String | null |
double | 0.0 |
Object | null |
char | u0000 |
float | 0.0f |
double | 0.0 |
long | 0L |
Let's understand the default value of the instance variable using an example.
Output :
The 0 and null are printed because the default value of int is 0, and the null is the default value of the String.
Instance Variables in Memory
Every object of a class has its own copy of the instance memory that is done by the run time of the java program.
Let's understand this using an image.
In the below image, three instances of class cars have their copy of the instance members of the class.
Difference between Class Variable and Instance Variable
Class Variable | Instance Variable |
---|---|
The class variable is declared using the static keyword. | Instance variables are not displayed with the static keyword. |
Class Variables are created during the compilation time of the class. | Instance variables are created after the object creation of the class. |
Class variables are accessed using the class name of the class. | Instance variables are accessed by using the "this" keyword or the reference of the class. |
Class variable can be accessed without creating an object. | Instance variable cannot be accessed without creating an object. |
Class variables are equally shared among all the objects of the class. | Instance variables are independent for every object of the class. |
How to Implement an Instance Variable in Java?
We can implement instance variables in the class body, but outside the scope of any class methods, constructors, or any kind of block.
Let's understand how to implement an instance variable in Java using an example.
In the below example, there are three instance variables present in the class Person i.e. name, age, and weight. We can see they are implemented in the class body but outside the scope of any method, block, or constructor.
Conclusion
- Instance members are used to represent the state of the object.
- Instance members are accessed by the "this" keyword in the class methods, constructor, and blocks.
- Instance members are stored in the heap section of the memory.