Int to Char in Java
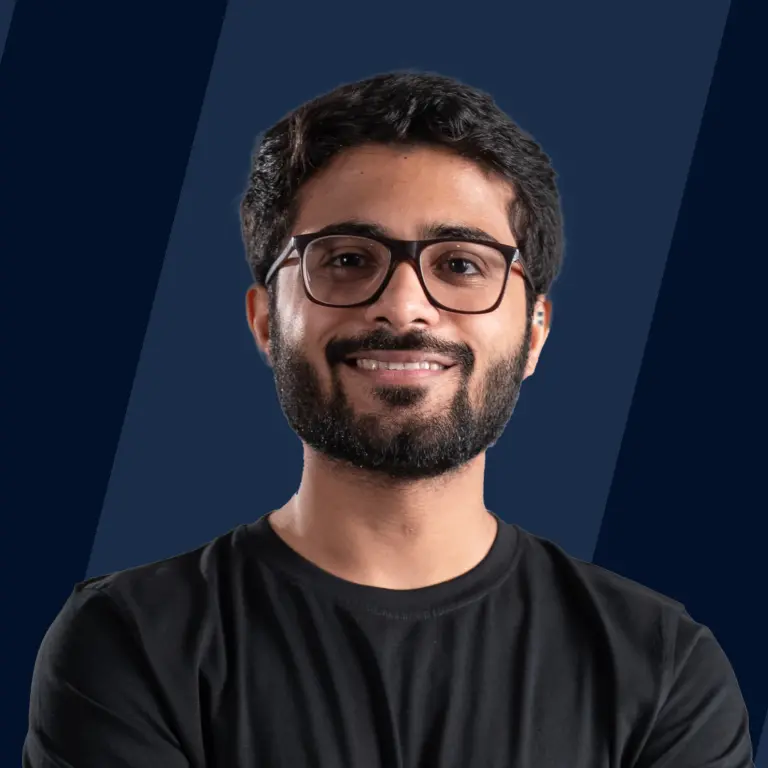
In Java, converting an integer to a character can be achieved through methods like typecasting, toString(), and forDigit(). Understanding Java's typecasting is crucial, as it involves converting data from 4 bytes (int) to 1 byte (char), often adding '0' for accurate representation. This article explores these methods in detail.
Using Typecasting in Java
We can very easily convert int to char using typecasting in Java. Typecasting basically means converting a particular data type to some other data type.
The syntax for typecasting in Java is:
Here data_type1 is the data type of the variable var1, and data_type2 is the data type to which var1 is converted by typecasting. This means that the value of var1 is converted to data_type2.
So, if we have an integer variable and we need to convert it into a character variable, we just need to typecast it by using before assigning it a value.
Note: that the variable will be assigned the character whose ASCII value is 98. Hence, if you want a particular alphabet or symbol to be converted from integer to character, make sure the integer holds the value of that alphabet/symbol in ASCII code.
Example: Java Program to Convert int to char using typecasting
Let us take an example to convert a given integer into character in Java using typecasting as described above.
Output
As shown in the output, the integer a is converted into a character using typecasting in Java and then stored in c.
The thing to note about this method is that the integer will not be converted into the character, but instead will get converted to the character for which the integer is the ASCII code. Hence, the code did not output 89, but Y as ASCII code of Y is 89.
Using toString() method in Java
The toString() method in Java is very useful when we want to convert the exact integer into a character. As we saw, this isn't possible using typecasting, but the toString() method does exactly this. Formally, the toString() method in Java returns the integer passed to it in string format.
Why as a string?
If the integer number passed to the toString() method has more than one digit, it won't fit in a character data type.
For example: if our integer is 66, then the toString() function will return "66", the string. Returning it as a character is not possible, as the character data type would be able to hold the value of only one digit. Hence, this method is useful for integers that have more than one digit.
The syntax for toString() method in Java is:
Here num is an integer variable, and is the resultant string.
Example: int to char by using toString()
Output
As shown by the output, integer 56 has been converted to string "56".
Using forDigit() method in Java
The forDigit() method in Java is used to convert a digit into a character in a specific radix. Here, radix is the base in which we are counting over integer. We need to pass two arguments to the forDigit() function: the digit to be converted into a character and a radix. Then the forDigit() function will return the digit as a character in that radix.
For example, if we call the forDigit() function with the integer value = 11 and the radix = 16, then the function will return the character b, as in radix 16 the value 11 evaluates to the character b.
If the integer value passed to the forDigit() method is not valid in the specified radix, or if the radix is invalid, a null character is returned.
The syntax for the forDigit() method in Java is:
Here, will be the resulting character, is the integer variable that will be converted to a character, and is the variable that stores the radix/base.
Example: int to char by using forDigit()
Output
As 12 in base 16 is c, forDigit() give the character 'c' as output which is then stored in ch.
By adding '0'
We can also convert an integer into a character in Java by adding the character '0' to the integer data type. This is similar to typecasting.
The syntax for this method is:
Here, is the resultant character, is the integer variable, and we are adding '0' to .
This method works because when we add the character '0' to the integer, it is converted into its ASCII value, which is 48. This is indirectly converting the value of to the ASCII value of the character , and the resulting character is the character whose ASCII value is , which will be itself when the value of is between 0 and 9.
Clearly, this method will only work for integers from 0 to 9 because above 9, adding 48 to the integer won't give us its ASCII value, and we will get the wrong answer.
Example: int to char by adding '0'
Output
Conclusion
- There are four standard ways to convert int to char in Java:
- using typecasting
- using toString() method
- using forDigit() method
- adding '0'
- Typecasting basically means converting a particular data type to some other data type, so using this, we can convert int to char in Java.
- The toString() method in Java returns the integer passed to it in string format. As it returns the integer in string format, this method is useful for integers with more than one digit.
- The forDigit() method in Java converts a digit into a character in a specific radix.
- We can also convert int to char in Java by adding the character '0' to the integer data type. This converts the number into its ASCII value, which after typecasting gives the required character.