Convert Int to String in C++
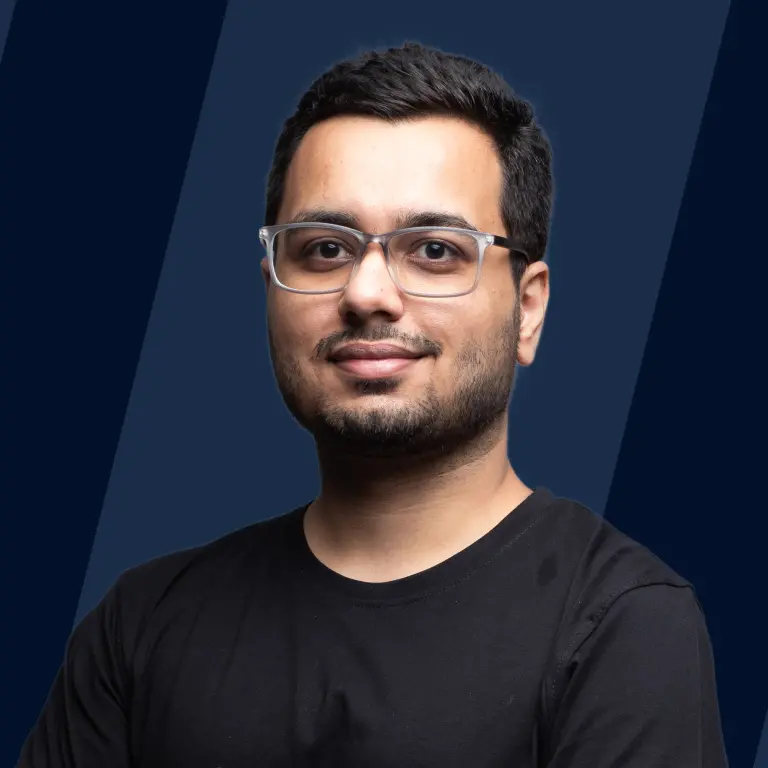
Sometimes, while writing a program, we need to typecast int data type to string data type. C++ provides built-in methods and functions that support integer numbers to string conversion. Int to string in C++ can be converted using the stringstream class, the to_string() function, the sprintf() Function, and boost::lexical_cast.
Prerequisites
Converting Integer to String in C++
C++ provides four methods to convert integer to string data type:
- Using the Stringstream Class
- Using the to_string() Method
- Using boost::lexical_cast
- Using sprintf() Function
We will learn how to convert integer to string in C++ with each method explained in detail along with examples in the upcoming sections.
Using the Stringstream Class
The stringstream class is included in the sstream header file and allows input/output operations on string-based streams. It can perform parsing in various ways. Operators >> and << are used to extract (>>) data from and insert (<<) data into the stream object. stringstream class includes the following basic methods:
- str(): The str() method of the stringstream class is used to retrieve or assign values of the underlying string object.
- clear(): This method is used to clear the contents of the stream object.
The >> and << operators can be used with stringstream objects to convert int to string in C++.
Description of syntax
- ss_object: This is an object of the stringstream class that is declared to perform input/output operation on the string.
- <<: This operator is used to insert the number into the string stream object.
- >>: This operator extracts the value present in stringstream object & stores the value in a string variable present on the operator's right-hand side.
Example
Let's see an example of using the stringstream class to convert an int to a string in C++ and then modify its value.
Here, we convert integer to string in c++ and store it in the my_string variable using stringstream class. After the conversion, we modify the value of the number by changing the character at the second index.
To insert the number into the stream object we are using << operator.
The >> operator is used to extract the data from the stream object, and the value is stored in the my_string variable.
Using the to_string() Method
Another way to convert a number to a string in C++ is to use the to_string() method. This function converts integers and numerical values of any data type to string.
The syntax of using the to_string() method is:
Definition of the to_string() function is included in the header file <string>, the function takes a numerical value as a parameter that needs to be converted to a string. The numerical value can be any data type, such as integer, float, double, long double, etc.
The function returns a string object corresponding to the value passed as an argument to the function.
Example
The following program shows the working of to_string() function in C++:
The above example shows an integer number with the value 4532. To convert it into a string, we are passing the number value to the to_string() function (defined in the <string> header file) that returns a string that we are storing in the my_string variable.
Using boost::lexical_cast
The boost::lexical_cast method defined in the library boost/lexical_cast.hpp is another method to inter-conversion of different data types, including integer, float, double and string. boost::lexical_cast provides a cast operator that converts a numerical value to a string value.
The syntax of boost::lexical_cast is:
Description of the syntax:
- data-type: This is the parameter to which the data type argument needs to be converted. We need to pass data-type as a string to convert integer to string.
- argument: This is the value that needs to be converted. In our case, an argument is an integer number.
Example
The following program illustrates the use of boost::lexical_cast to convert int to string.
In the above example, the integer 4532 is converted into a string by passing the integer number as an argument to the boost::lexical_cast<string> function.
Using sprintf() Function
The sprintf() function offers a way to convert an int to string in c++ by printing the integer value into a string buffer. It's part of the C Standard Library (<cstdio> or <stdio.h>), so while it's more commonly seen in C programs, it can be used in C++ for certain applications. This method involves formatting the integer as a string and storing it in a char array.
Example
Output:
Complexity:
- Time Complexity: O(n)
- Space Complexity: O(n)
Conclusion
- Understanding how to convert integers to strings in C++ is fundamental for manipulating number data as text.
- Converting int to string in C++ can be efficiently accomplished using several built-in methods, accommodating various programming requirements.
- Key methods for integer-to-string conversion in C++ include the string stream class, the to_string() function, and boost::lexical_cast. Each provides a tailored approach to transforming a number into a string.
- For anyone looking to convert int to string in C++, leveraging the string stream class, to_string() method, or boost::lexical_cast ensures a smooth transition from numeric to textual representation, enhancing the versatility of integer data handling.