Java Convert int to String
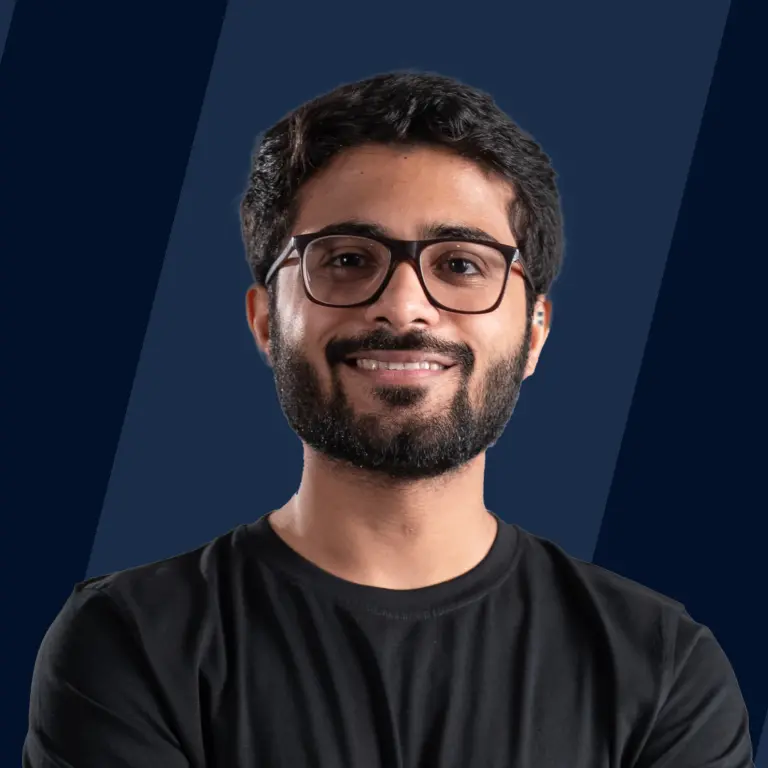
In Java, converting an integer (int) to a string is a common operation essential for data processing and output formatting. This article explores "int to string Java" conversion methods, highlighting efficient techniques and best practices to integrate numeric data into textual contexts seamlessly.
How to Convert int to String in Java?
Using the toString() Method of the Integer Class
The toString() method of the Integer class expresses an integer value as a string. It takes an integer value as an argument and returns the string representation of the argument value.
Syntax:
Example Java Program:
Output:
Explanation:
- The integer variables and values were converted into string types using the Integer.toString(int_var) function, and the resultant value was then assigned to the String objects. We have printed the values of the integer and String objects as the last step.
Using the valueOf() Method of the String Class
valueOf() is a static method that is overloaded within the String class for all the Java primitive types. valueOf() method takes one argument, i.e., the integer variable or an integer value, and returns the string representation of that integer value.
Syntax:
Example Java Program:
Output:
Explanation:
- In the above Java program, we initialize an integer a = 39 and directly use 49 for conversion. By employing String.valueOf(), we convert these integers to strings, assigning the results to str_a and str_b. The values of a, str_a, and str_b are then printed, showcasing the conversion.
Using Integer(int).toString() Method of Integer Class
To use the Integer(int_var).toString() technique to convert the argument integer value to the String type, we first need to create an instance of the Integer class.
Syntax:
or
Example Java Program:
Output:
Explanation:
- In the above Java program, we initialize an integer a = 7007 and also use the literal 39 for conversion. The results are stored in String variables and displayed, showcasing the conversion of the Integer object obj and the strings str_a and str_b.
Using Concatenation with an Empty String
The DecimalFormat class of the java.text package in Java is used to format integer values using a custom formatting pattern. The DecimalFormat class can format various sorts of numbers by converting the numeric values to String class objects.
Syntax:
Note: Here, pattern is an argument passed in the DecimalFormat() class initialization, which refers to the formatting we would like to have on our string literal. If the pattern is only "#", the integer value will be converted to a string, but if the pattern is something like "#,###" (means including some comma), then the string obtained from the integer value will contain "," (comma) after every three digits from the right.
Example Java Program:
Output:
Explanation:
- In the above Java program, we initialize two integers, a = 4500 and b = 4350, and also use a direct value of 910000 for string conversion. The output demonstrates that a converts to str_a = 4500 without commas, whereas b and 910000 are formatted to str_b = 4,350 and str_c = 910,000, incorporating commas for every three digits from the right. The resulting string values str_a, str_b, and str_c are then displayed.
Advanced Methods to Convert int to String in Java
Using DecimalFormat Class
Syntax:
Code:
Output:
Explanation: The DecimalFormat class is used for formatting numbers in a locale-sensitive manner. In this example, the #,### pattern is used to format the integer, adding a comma as a thousand separator, converting the int to a formatted String.
Using StringBuffer Class
Syntax:
Code:
Output:
Explanation: The StringBuffer class creates a mutable sequence of characters. The append method converts the integer to a StringBuffer instance, and the toString method converts it into a String.
Using StringBuilder Class
Syntax:
Code:
Output:
Explanation: Similar to StringBuffer, the StringBuilder class is used for creating a mutable sequence of characters. The difference lies in synchronization; StringBuilder is faster as it's not synchronized.
Using Special Radix and Custom Radix
Syntax:
Code (Special Radix - Binary):
Output (Binary):
Code (Custom Radix - Hexadecimal):
Output (Hexadecimal):
Explanation: Java provides the ability to convert integers to strings with different radix values, enabling binary, hexadecimal, and other base conversions. The Integer.toString method uses the desired radix, allowing for flexible string representations of numeric values.
Conclusion
- Converting integers to strings in Java is a fundamental operation that supports various data processing and formatting needs.
- Simple methods like Integer.toString(int), String.valueOf(int), and new Integer(int).toString() offer straightforward conversions.
- For customized formatting, the DecimalFormat class provides locale-sensitive formatting options, allowing the inclusion of patterns like commas for thousands of separators.
- Mutable classes like StringBuffer and StringBuilder can be used for efficient conversion, especially in scenarios involving multiple string manipulations.
- Each method has its use case, from simple conversions to complex formatting and performance-sensitive applications, highlighting the versatility of Java in handling numeric and string data types.