Integer Class in Java
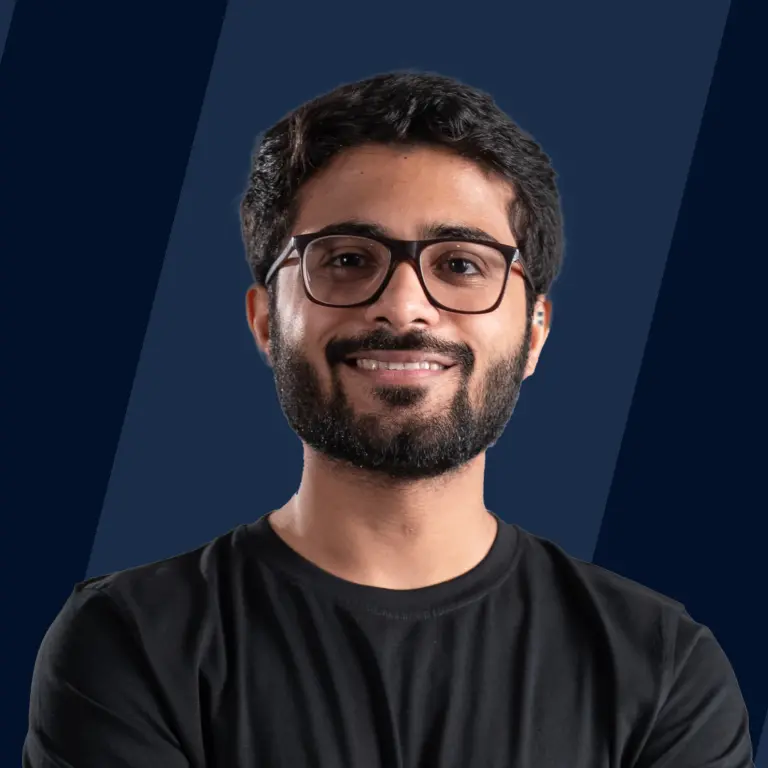
The java.lang.Integer class creates an object out of an int value. An object of type Integer has a single field with the type int. The Java Integer class has methods to convert an int to a String and vice-versa, as well as various constants(MAX_VALUE,MIN_VALUE, SIZE,TYPE) and methods that deal with ints.
What is Integer Class in Java?
The Java.lang.Number package contains the Java Integer class. An int value can be successfully handled using the methods in the integer class, which is a wrapper class for the primitive type int. For example, an int value can be efficiently represented as a string and vice versa. A single int value may be stored in an object of the Integer class.
Constructors of Integer Class in Java
It is a unique method that is automatically called when an object is created. Different constructors are included in the Java Integer class. Let's investigate them right away.
1. Integer(int b)
This creates an Integer object with a fresh allocation representing the given integer value.
Syntax
Parameter
b: The initialization value
Example
In this example, we are going to see how we can create an Object of Integer Class using Integer(b) constructor.
Output
2. Integer(String s)
It creates an Integer object with the int value supplied by the string representation as its starting value. Radix or base is assumed to be 10 by default.
Syntax
Parameter
s: The int value's string representation.
Throws
NumberFormatException If the string does not represent any int value.
In the example below, we will find what exceptions are thrown by the Integer(string) Constructor.
Output
Code Explanation In the code below, "q,we" is not an Integer String, so it throws NumberFormatException.
Example
In this example, we will see how to use the Integer(s) constructor to create a Object of Integer class.
Output
Code Explanation
In the above code, the Integer string is passed using a constructor so that it will create an object of the Integer class with an Integer value of 12.
In the 8th line of code, the Integer class Object is created using int value, which will create an object of the Integer class with value 12.
Methods of Integer Class in Java
Method | Syntax | description |
---|---|---|
bitCount() | public static int bitCount(int i) | It returns the number of 1-bits that make up the supplied int value's binary representation in the 2's complement. |
toString() | public String toString(int b) | gives the int value's corresponding string back. |
toHexString() | public String toHexString(int b) | Returns a string that represents the integer value in hexadecimal characters or the string corresponding to the integer value in hexadecimal form. [0-9] [a-f] |
toOctalString() | public String toOctalString(int b) | This function returns the string that corresponds to the integer value in octal form, or the integer value represented by the characters [0-7] in the string. |
toBinaryString() | public String toBinaryString(int b) | The function returns a string that corresponds to the integer's value in binary digits or [0/1] hexadecimal characters. |
valueOf() | public static Integer valueOf(int b) | returns the Integer object that has been initialised with the supplied value. |
valueOf(String val,int radix) | public static Integer valueOf(String val, int radix) throws NumberFormatException | A second overloaded function that does comparable tasks to new Integer(Integer.parseInteger(val,radix)) |
valueOf(String val) | public static Integer valueOf(String s) throws NumberFormatException | A overloaded function that does comparable tasks to new Integer(Integer.parseInt(val,10)) is available. |
parseInt() | public static int parseInt(String val, int radix) throws NumberFormatException | by processing the string in the given radix, returns an int value. Unlike valueof() , which produces an Integer object, it returns a primitive int value. |
getInteger() | public static Integer getInteger(String prop) | returns either null if the requested system property does not exist or an Integer object represents its value. |
decode() | public static Integer decode(String s) throws NumberFormatException | returns a decoded version of the supplied string as an Integer object. |
rotateLeft() | public static int rotateLeft(int val, int dist) | Rotates the bits remaining after the specified distance in the given value's two's complement form, returning a primitive int. The most significant bit is rotated to the right, or least significant position, while turning to the left; this is known as cyclic bit movement. A negative distance indicates the right rotation. |
rotateRight() | public static int rotateRight(int val, int dist) | Rotates the bits to the right by the specified amount in the twos complement form of the input value to produce a primitive int. The least significant bit is rotated to the left, or the most significant position, when turning to the right, creating a circular movement of bits. A negative distance indicates left rotation. |
byteValue() | public byte byteValue() | returns the matching byte value for this Integer Object. |
shortValue() | public short shortValue() | a short value corresponding to this Integer Object is returned. |
intValue() | public int intValue() | returns a int value corresponding to this Integer Object. |
longValue() | public long longValue() | returns the equivalent long value for this Integer Object. |
doubleValue() | public double doubleValue() | returns the matching double value for this Integer Object. |
floatValue() | public float floatValue() | returns the equivalent float value for this integer object. |
hashCode() | Text | Text |
Text | public int hashCode() | gives the matching hashcode for this Integer Object. |
bitcount() | public static int bitCount(int i) | gives the provided integer's twos complement's number of set bits. |
numberOfLeadingZeroes() | public static int numberofLeadingZeroes(int i) | Returns the amount of 0 bits before the highest 1 bit in the value's twos complement form. |
numberOfTrailingZeroes() | public static int numberofTrailingZeroes(int i) | returns the amount of 0 bits that follow the last 1 bit in the value's twos complement form, |
highestOneBit() | public static int highestOneBit(int i) | returns a result that contains no more than one bit in the highest place in the original value. This function returns 0000 0000 0000 1000 if the value supplied is zero, or 0000 0000 0000 1111 else (one at the highest one bit in the given number) |
LowestOneBit() | public static int LowestOneBit(int i) | returns a value that contains no more than one bit in the lowest position in the value that was provided. This function returns 0000 0000 0000 0001 if the value supplied is zero, or 0000 0000 0000 1111 else (one at highest one bit in the given number) |
equals() | public boolean equals(Object obj) | used to evaluate whether two Integer objects are equal. If both objects have the same int value, this function returns true. Only when checking for equality should it be utilised. The compareTo method should be chosen in all other circumstances. |
compareTo() | public int compareTo(Integer b) | used to check for numerical equivalence between two Integer objects. This should be used to distinguish between less and greater values when comparing two Integer values for numerical equality. For less than, equal to, and larger than, returns a value less than 0,0, and a value greater than 0. |
signum() | public static int signum(int val) | returns -1 for low values, 0 for zero, and +1 for high values. |
reverse() | public static int reverseBytes(int val) | returns a primitive int value that reverses the bits of the specified int value's two's complement form. |
reverseBytes() | public static int reverseBytes(int val) | returns a primitive int value that reverses the specified int value's byte order in two's complement form. |
static int compareUnsigned(int a, int b) | public static int compareUnsigned(int a, int b) | This method quantitatively compares two int values while treating them as unsigned. |
static int divideUnsigned(int dvd, int dvs) | public static int divideUnsigned(int dividend, int divisor) | Text |
static int max(int x, int y) | public static int max(int x, int y) | This function simulates calling Math.max by returning the larger of two int values. |
static int min(int a, int b) | public static int min(int x, int y) | This function simulates calling Math.min by returning the lesser of two int values. |
static int parseUnsignedInt(CharSequence t, int startIndex, int endIndex, int radix) | public static int parseUnsignedInt(CharSequence str,int startIndex,int endIndex int radix) throws NumberFormatException | The CharSequence input is parsed by this method as an unsigned int in the given radix, starting at the given beginIndex and going all the way to endIndex - 1. |
static int parseUnsignedInt(String str, int radix) | public static int parseUnsignedInt(String str,int radix) throws NumberFormatException | By using the radix supplied by the second parameter, this method converts the string input into an unsigned integer. |
static int remainderUnsigned(int dvd, int dvs) | public static int remainderUnsigned(int dividend, int divisor) | When dividing the first parameter by the second, this method returns the unsigned remainder, where each argument and the result are both interpreted as unsigned values. |
static int sum(int x, int y) | public static int sum(int a, int b) | adds two integers as per the + operator. |
static long toUnsignedLong(int x) | public static long toUnsignedLong(int x) | This function returns an unsigned decimal number that is a string representation of the argument. |
Examples
In the below code, we are going to see examples of the method of the Integers class that we discussed above.
Output
Examples of Integer Class in Java
In the below examples, we are going to use the Integer class Constructor and Method
Example 1
Output
Conclusion
- In Java, an integer class contains a value of the primitive type int within an object. The int type is the only field that exists in an object of type Integer.
- Different constructors and methods are included in the Java Integer class.
- Integer class provides two Constructor Integer(int val) and Integer(String s)
- Integer Class also provides a variety of methods that can Convert Integer to String, HexString, OctalString, and BinaryString.
- By using the Methods of Integers class, we can perform operations on bits of an Integer.