Java Integer Division
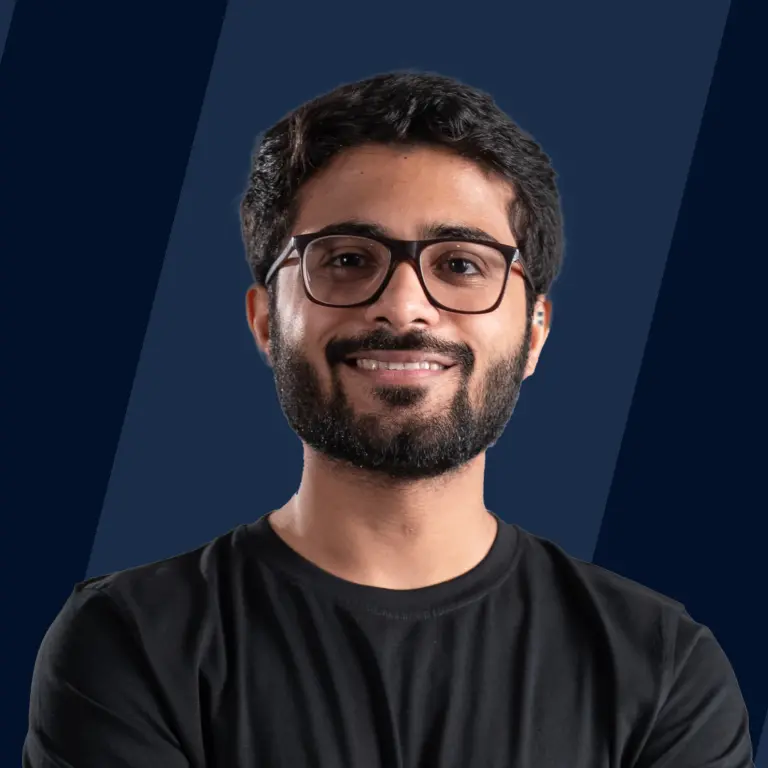
Overview
Integer division Java : In Java, when two integer values are divided, the result is an integer. This means that any fractional part of the division is discarded, leading to a loss of precision or accuracy in the result. For example, if you divide 7 by 3, the result is 2, even though the exact value is 2.3333333333.
Introduction to Java Integer Division
As we know Java is a statically typed language, that is all the values in Java are stored in any particular data type, and the data types of all the variables and expressions are already known at the compile time, hence data types are mandatory in Java. Now, Java has a variety of data types for storing various kinds of values, with Integer being one of them.
The integer is a primitive data type in Java, which consist of only single integer type values in it, for instance, 5, 10, 20. Any value of decimal type cannot be stored in an integer, for instance, if we try to store a value 5.339 inside any variable of integer type, the variable will only store the whole number part that is 5, ignoring the decimal part of the value.
Let's say, we have three variables a, b, and c of integer type, and we are storing value in a, and b variables int a = 10, int b = 3, and then we are dividing the integer variable a with b, and storing the result inside the variable c, . We can observe, that the resultant value is of decimal type, however, it will be stored as an integer type inside c, as all the variables are of integer type. Hence, we will lose the decimal part, resulting in a loss of precision or accuracy. In Java when two values of integer type are divided, it returns the value of integer type. However, unlike addition or any other operation, there is a loss of precision or accuracy in the case of division. Let us understand this in detail.
Program for Simple Division
Let us now look at a small example of the simple division in Java code, to make it more clearly understood.
Abstract: In this code, we will see how the simple division works in Java programming language.
Code:
Ouptut:
Explanation:
- In the above code, firstly, we are creating two variables aand b of integer type inside the main method.
- Then we are performing a division operation and store the result in another variable c of integer type.
- The numbers which we are dividing are 15 and 5, and the result of their division is 3, which is an accurate answer because in this case 5 leaves 0 remainder after dividing it by 15. So in the above code, the output is accurate.
Losing Data on Java Integer Division
In Java, loss of data occurs, when we try to divide two integer type values, and they are not completely divisible(remainder not equal to 0). In that case, the result will be of integer type, and it will ignore the decimal part of the result, which ends up losing data. However, we can prevent this loosing of data, by using float data type to store our values, and also their division. We will discuss this float data type ahead in the article.
Now, let us now look at an example where the result is not accurate, after the simple division of two integer-type values.
Abstract: In this code, we will see how the loss of data occurs in the case of simple division in Java programming language.
Code:
Output :
Explanation:
- In the above code, firstly, we are creating two variables aand b of integer type inside the main method.
- Then we are performing a division operation and store the result in another variable c of integer type.
- The numbers which we are dividing are 15 and 7, and the result of their division is 2, which is not an accurate result, because when 15 is divided with 7 the actual result should be 15/7 = 2.14285, but due to we are storing the result of the division inside an integer type variable, the result will be of integer type and hence it ignores the decimal part of the value .14285 and just stored the whole number part 2 inside c variable. So in the above code, the output is not accurate, and it ends up resulting loosing of data.
Changing the Datatype of Variables from Float to Int and Vice-versa
Let us now discuss about what will happen if we change our variables from integer to float and vice-versa. Before getting started, let us get a short description of float data type in Java.
Float Data Type: The float keyword in Java is a primitive data type, It usually stores the value in decimals or fractions. For example 1.233, 3.14, 2.0342.
As float can store decimal types of values, if we divide any two variables of float type, and store the result inside a variable of float type. The stored result will be accurate, and there will be no data loss. At this time the decimal part of the value will also be stored.
Let's say, we have three variables a, b, and c of float type, and we are storing value in a, and b variables float a = 15, int b = 7, and then we are dividing both the float type variable a and b, and storing the result inside the variable c of float type. c = a/b = 15/7 = 2.14285. We can observe, that this time the value is accurate.
Let us look at different scenarios of how the division works after changing the data type of variables from float to int and vice-versa.
Example:
Let us now look at a small example in Java code, to make it more clearly understood.
Abstract: In this code, we will see different scenarios of how the division works and what will be the result after changing the data type of variables from float to int and vice-versa.
Code:
Output:
Explanation:
- In the above code, we are covering different scenarios of dividing two values of integer or float type and printing their result.
- In the first operation, we can see that we have two integer values. Now, we are performing a division operation and storing the resultant value into the variable res1, which is of integer type. You must be knowing that if we divide 10 by 3(suppose), then we would get something near to 3.333..., but since we performed division between two integers, we will be losing the data after the decimal point and our result will be only 3 (in this case). This clearly results in a loss of precision of the final result. Then we are storing the result as an integer type The result will also be of integer type, and it won't be accurate.
- In the second operation, we can see that we have two double and integer type values. Now, we are performing a division operation and storing the resultant value into the variable res2, which is of double type. You must be knowing that if we divide 10 by 3(suppose), then we would get something near to 3.333..., and as we performed division between an integer and double type value, there will be no loss of data after the decimal point and our result will be 3.333 (in this case). This does not result in any loss of precision of the final result. Then we are storing the result as double type The result will also be of double type, and it will be accurate.
- In the third operation, we can see that we have two integer and double-type values. Now, we are performing a division operation and storing the resultant value into the variable res3, which is of double type. You must be knowing that if we divide 10 by 3(suppose), then we would get something near to 3.333..., and as we performed division between a double and integer type value, there will be no loss of data after the decimal point and our result will be 3.333 (in this case). This does not result in any loss of precision of the final result. Then we are storing the result as double type The result will also be of double type, and it will be accurate.
- In the fourth operation, we can see that we have two double-type values. Now, we are performing a division operation and storing the resultant value into the variable res4, which is of double type. You must be knowing that if we divide 10 by 3(suppose), then we would get something near to 3.333..., and as we performed division between two double type values, there will be no loss of data after the decimal point and our result will be 3.333 (in this case). This does not result in any loss of precision of the final result. Then we are storing the result as double type The result will also be of double type, and it will be accurate.
- In the fifth operation we have two values of integer type, and we are dividing them and storing their result in a variable of double type. This is an interesting case, hereafter dividing both the value of the integer type we will get an integer type value, which will result in losing the precision
- In the fifth and final operation, we can see that we have two integer values. Now, we are performing a division operation and storing the resultant value into the variable res5, which is of double type. You must be knowing that if we divide 10 by 3(suppose), then we would get something near to 3.333..., but since we performed division between two integers, we will be losing the data after the decimal point and our result will be only 3.0 (in this case). This clearly results in a loss of precision of the final result. However, please note that we are getting the result as a double type because we are storing the final result in a double-type variable.
- In short we can conclude some important observations based on the datatype of divisor and dividend :
- int / int = int
- double / int = double
- int / double = double
- double / double = double
Conclusion
We learned about the Java integer division. Let us now recap the points we discussed throughout the article:
- In Java when two values of integer type are divided, it returns the value of integer type.
- As, an integer cannot contain any decimal or exponential type value, there is a loss in precision or accuracy in the result.
- Java is a statically typed language, that is all the values in Java are stored in any particular data type, and the data types of all the variables and expressions are already known at the compile time, hence data types are mandatory in Java.
- Integer is a primitive data type in Java, which consist of only single integer type values in it, for instance, 5, 10, 20.
- We have seen code examples of simple division in Java.
- In Java, loss of data occurs, when we try to divide two integer type values, and they are not completely divisible(remainder not equal to 0). In that case, the result will be of integer type, and it will ignore the decimal part of the result, which ends up losing data.
- Float can store decimal types of values, if we divide any two variables of float type, and store the result inside a variable of float type. The stored result will be accurate, and there will be no data loss.
- In Java, the division of integers will work perfectly for the cases where the remainder is 0, and the divisor completely divides the dividend. If the remainder is 0, the result of dividing the two integers will be any whole number, and as the integer can store the whole numbers, hence there will be no data loss.