What is Integer.Min_Value in Java?
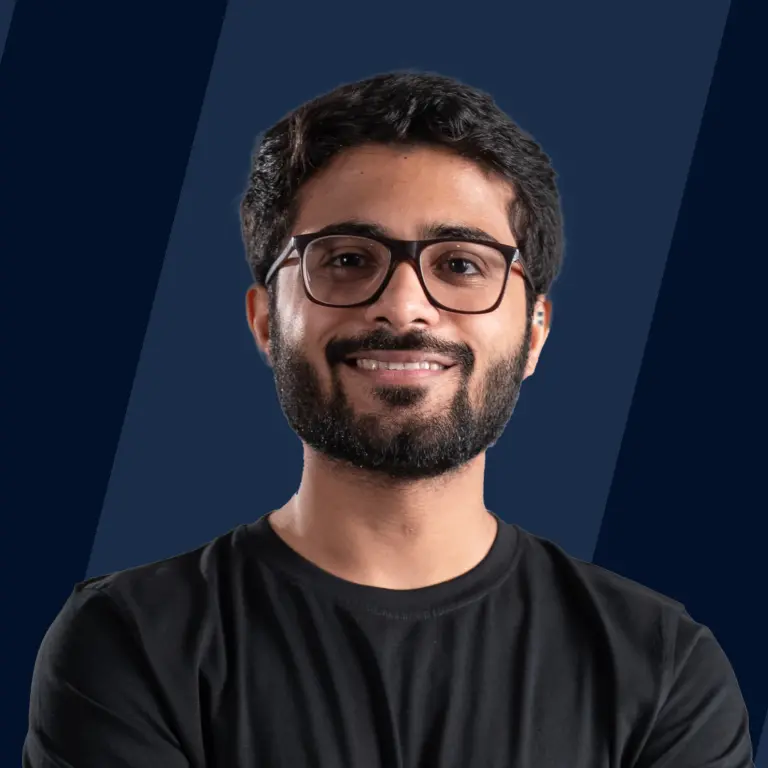
Overview
In competitive programming, we frequently require the maximum or minimum possible values for variable assignments, which can be daunting to remember. Java provides constants as convenient shortcuts for these extreme values, making variable initialization easier.
Pre-requisites
It's recommended to go through the following pre-requisites to understand few basic concepts that'll be used in this article:
What is Integer.MIN_VALUE in Java?
Integer.MIN_VALUE is a predefined constant within the Integer class, denoting the smallest possible integer value that can be held in 32 bits, equivalent to -2147483648 or -2^31. It is the bottommost limit for any integer variable in the Java programming language.
Code:
Output:
Explanation:
Any value less than Integer.MIN_VALUE will lead to an overflow in memory and will be a positive value.
Let's see an example in Java
Code:
Output:
Note: In the world of Java, when one is dealing with integer values that might go beyond the limits set by Integer.MAX_VALUE and Integer.MIN_VALUE, we can always go for the long data type and use Long.MAX_VALUE and Long.MIN_VALUE instead which can handle numbers much larger than int.
But what if you're dealing with integers that are so colossal that even longs can't contain them without risk of overflow or underflow?
Well, that's when you bring in the big guns – the BigInteger and BigDecimal classes from the java.math package. They are capable of handling integers of great proportions, offering limitless length and precision for your calculations.
Examples
Let's see an example where we can put Integer.MIN_VALUE to use
Code:
Output:
Explanation:
In the above code as you can see we're initializing the max_value variable to type int to a Integer.MIN_VALUE which sets a minimum base value upon which elements in the array can be compared again and we can get the maximum valued element.
What is Integer.MAX_VALUE in Java?
In Java, the constant Integer.MAX_VALUE symbolizes the highest attainable positive integer value. It's a numerical constant included in the Integer class of the java.lang package. This value, which occupies 32 bits, precisely equals or .
Code:
Output:
Explanation:
Integer.MAX_VALUE in Java serves the purpose of assigning the maximum attainable integer value to any variable, eliminating the need to memorize the specific number.
Examples
Let's see an example where we can put Integer.MAX_VALUE to use
Code:
Output:
Explanation:
In the code above, we initialize the min_value variable as an int with Integer.MAX_VALUE, setting an upper limit for comparing array elements to find the minimum value. Java manages integer overflow and underflow gracefully. Exceeding the maximum limit results in negative values, and values below the minimum limit become positive.
FAQs
Q: Can I change the values of Integer.MIN_VALUE and Integer.MAX_VALUE in Java?
A: No, these constants are fixed and cannot be modified. They represent the boundaries of the 32-bit signed integer data type.
Q: What is the purpose of Integer.MIN_VALUE and Integer.MAX_VALUE?
A: These constants are helpful for defining limits, error handling, and ensuring data does not exceed the valid integer range in Java applications.
Q: What happens if I try to use Integer.MIN_VALUE as an index in an array?
A: Using Integer.MIN_VALUE as an index is invalid and may lead to unexpected behavior or errors in your Java code. Array indices must be non-negative integers.
Conclusion
- Wrapper types in Java are classes designed to envelop primitive types, enabling you to work with them as if they were objects
- Integer.MIN_VALUE is a predefined constant within the Integer class that stands as the bottommost limit for any integer variable in the Java programming language.
- In Java, the constant Integer.MAX_VALUE symbolizes the highest attainable positive integer value.
- You can go for Long, BigDecimal and BigInteger data types for storing larger values
- Using Integer.MIN_VALUE as an index is invalid and may lead to unexpected behavior or errors in your Java code. Array indices must be non-negative integers.