String intern() Method in Java
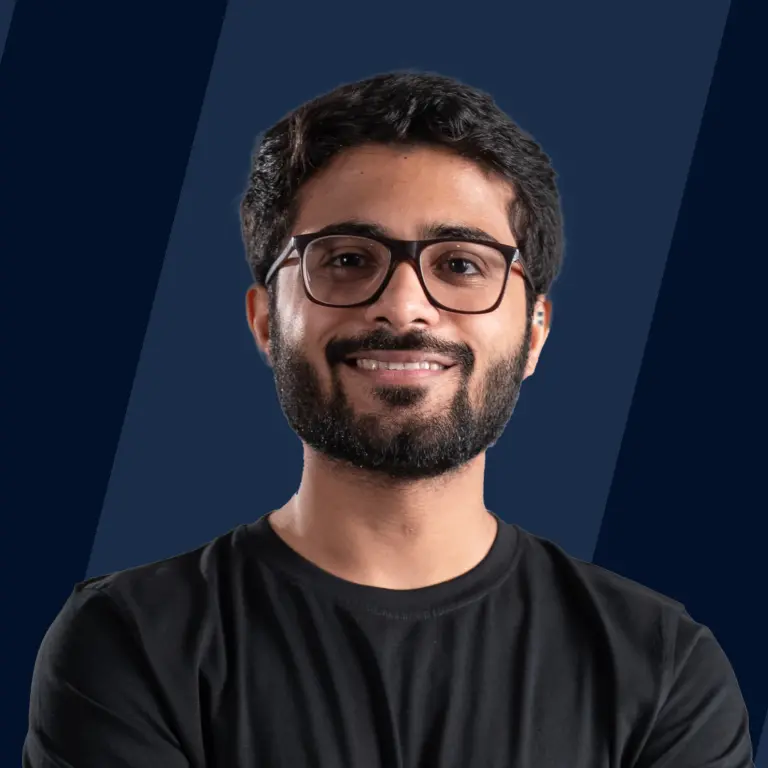
Overview
Before we proceed towards the string intern() method in Java, let us look at the ways by which we can create strings.
String literal
This is the simplest and most direct way to declare a string. It is done using double quotes.
Explanation - Here the first string gets created in the string pool. When the compiler executes the second line before creating the string, it checks the string pool and finds the same string so it does not create a new string. Instead, it returns the reference of str to str1.
A String Pool in Java is a special storage space in Java heap memory. The other names of string pool are String Constant Pool or even String Intern Pool. Java strings are created and stored in this area.
The "new" Keyword
The new keyword in Java forces a new instance to be always created irrespective of whether the value was used before or not. These strings are stored in heap and not string constant pool.
Here we can see that although the values of s1 and s2 are the same, they are not equal. This is because we have created them using the 'new' keyword.
The figure below shows the representation of the string constant pool and heap.
Note: Strings created using string literals are stored in the String constant pool. The Strings created using the "new" keyword forces a new instance to be created irrespective of the value in the heap.
What is Java String Interning?
String Interning in Java is a process where identical strings are searched in the string pool and if they are present, the same memory is shared with other strings having the same value.
The intern() method creates an exact copy of a String that is present in the heap memory and stores it in the String constant pool.
Note - intern() method is used to store the strings that are in the heap in the string constant pool if they are not already present.
The Java intern() Method
The Java intern() method is used to create an exact copy of a String that is present in the heap memory and stores it in the String constant pool.
It is important to note that if another String with the same value already exists in the String constant pool, then a new object won't be created and the new reference will point to the other String.
Method Signature
This creates a function intern() in Java which has the return data type of String and does not take any parameters.
Syntax of intern() in Java
or
The above two ways are used to invoke the intern() method. The first one is directly used on a string literal, whereas the second one is used with the new keyword.
intern() Parameters in Java
The intern method does not take any parameters.
Java intern() Return Values
It returns a canonical representation for the string object.
Exceptions
There are no exceptions related to the intern() method.
Example of String intern() in Java
Output
false
true
Explanation - The strings str1 and str2 are not equal as they have different memory location in the heap. When we apply the intern() method on str1 and store it in str3, it gets created in the String constant pool. But when the compiler executes the statement for applying the intern() method on str2, it checks the String constant pool first if the value is already present or not.
Here, since the value "Java" was already present, it only returns the reference and does not create the string again.
The Need and Working of the String.intern() Method
Strings in Java are immutable in nature. Immutable means they have a constant value and even if they are altered, instead of reflecting the alterations in the original string, a new object is created. This immutability is achieved through String Pool. String pool is advantageous as a new string with a previous value doesn't get created again. But, one drawback is that when we change the value of the string, it creates a new object which results in wastage of time. As it takes time to copy the previous value to a new variable and then reflect the changes made by the user.
The intern() method is used to store strings in the String constant pool and is majorly used if we want the strings present in the heap to be stored in the String constant pool. A string pool is similar to the way objects are allocated. It is empty by default and it is a part of the Java String class. When we create a string, it uses some amount of space in the heap. If we create a lot of strings, it proves to be a costly affair in terms of memory. In order to escalate the performance of our code and reduce memory usage, JVM optimizes the way in which strings are stored with the help of a string constant pool.
It is important to understand that when we create the string literal, it internally calls the intern() method, even if the user hasn't explicitly called it.
Whenever a new string is created, JVM first checks the string pool. In case, if it encounters the same string, then instead of creating a new string, it returns the same instance of the found string to the variable. If the string was not present, it creates a new string literal then allocates memory to it.
Therefore, this method helps to save memory space.
Takeaway - This method is used to save memory space as it only creates the strings with values that are not already present.
Conclusion
- The Java String intern() method is used to return the canonical representation of the String object.
- A String Pool in Java is a special storage space in Java heap memory where the strings are stored.
- The intern() method creates an exact copy of a string that is present in the heap memory and stores it in the String constant pool if not already present. If the string is already present, it returns the reference.
- The intern() method helps to save memory space and reuse it efficiently at the cost of time. If we want a number of operations to be done on a string then we use the StringBuffer and StringBuilder classes which create mutable string objects.