What is Used to Find and Fix Bugs in the Java Programs?
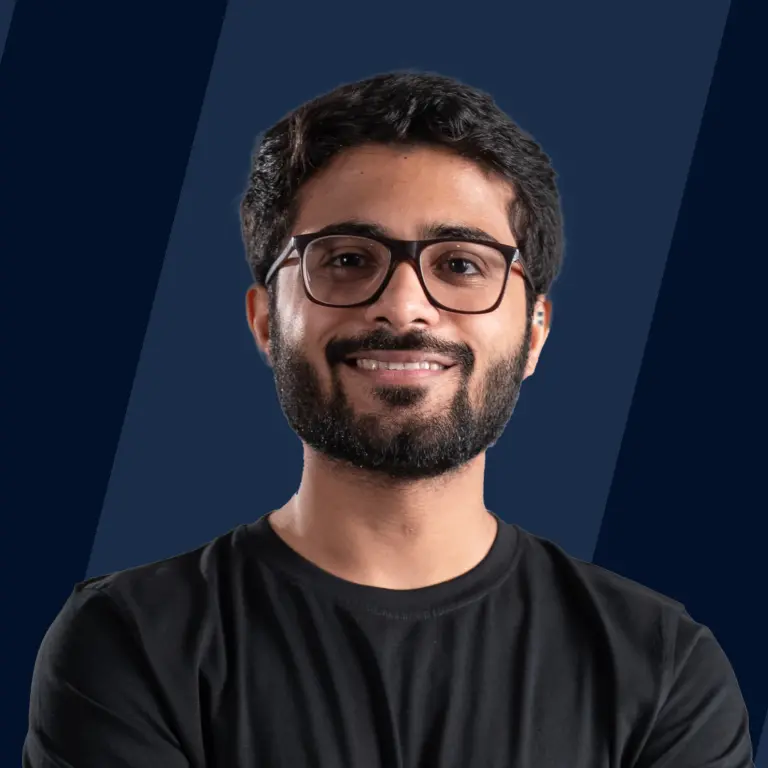
What is Used to Find and Fix Bugs in Java Programs?
The Java Debugger, also known as JDB, is used to find and fix bugs in the Java program. Debugging is the process of finding and removing bugs in code. In Java, if we want to debug a code, the Java Debugger is used to fix the bugs in the Java Debug Interface(JDI).
Debugging Techniques
Debugging is the process of finding and removing the bugs in the code. In short, it means removing the bugs(de-bug). This helps in getting the desired output of the code by analysing the errors in the code. Debugging a short code manually can be easy, but it becomes very tedious when the same task has to be done for a longer piece of code. In such a case, it is recommended to debug using a debugger. A debugger checks the code line-by-line, inspects the elements of a program and fixes the bugs.
Debugging techniques include-
- Traditional debugging
- Advanced debugging
Traditional method— Traditional debugging includes using the print statement after every major line of code that we write. This method is not used nowadays.
Example-
Advanced method- In advanced debugging, we can debug by using the following steps-
- stepping- An action that tells the debugger to step over a given line.
- breakpoints- A breakpoint is an intentional pausing or stopping at a place in a program, put in place for debugging purposes. It is also simply referred to as a pause.
- ** exceptions/watchpoints- ** This is used in ABAP (Advanced Business Application Programming). It is a type of breakpoint that shows an area of memory associated with a data item that we want to watch, pausing our application when that memory is updated.
Types of Debugging
There are several types of debugging methods like-
- By Java Bytecode
- Remote debugging
- Commenting the code
- Debugging on demand
- Optimised code debugging
- Attaching class to a running program
The Java Debugger (JDB)
The Java debugger is a tool for debugging a program on the command line and fixing bugs in the Java Debug Interface(JDI).
JDB has three units-
- Java Debug Wiring Pool (JDWP)- The Java Debug Wiring Pool uses the Java Debug Wire Protocol to communicate between the debugger and the Java Virtual Machine, which it debugs.
- Java Debugger Interface (JDI)- The Java Debugger Interface is a high-level Java API providing valuable information for debuggers and similar systems needing access to the running state of a virtual machine.
- Java Virtual Machine Tool Interface (JVM TI)- Java Virtual Machine Tool Interface allows a program to inspect the state and control the execution of applications running in the Java Virtual Machine.
JDB - Installation
We will learn how to install JDB on Windows.
Let us know the system requirements-
- JDK- Java SE JDK 1.5 and above
- Disk Space- There is no minimum requirement
- Operating System Version- Windows XP and above
- Memory- It is recommended to have at least 1 GB of RAM
Installation Steps
1. JDK Installation For JDB to run, we must have JDK(Java Development Kit) installed on our computer. If you have already installed JDK, you can check the current version in the command prompt.
To check the version, use the command java -version in the command prompt. You'll see an output like-
Which means JDK is installed on your computer. In case JDK is not installed, you can download it here.
2. Set Up Java Environment Variable Set up the Java Environment variable JAVA_HOME as a path to where Java is installed as a base directory.
3. Ensure the JDB Installation To ensure the JDB installation, use the command jdb—version to check the installed JDB version.
Syntax
For using JDB, the syntax is-
options- They are the list of command-line options that help debug a Java program in an efficient way. We will know more about this in the upcoming section.
class- This is the name of the class which we want to debug.
jdb- It is used to call jdb.exe from JDK.
arguments- Arguments are the input values given to a function at the runtime. Eg- args[0],args[1] etc.
List of Options Accepted by JDB
The following is the list of options accepted by JDB-
Option name | Description |
---|---|
-attach | This command is used to attach the Virtual Machine by using the default connection. |
-listen | This command is used to wait for the virtual to get connected to a specified address. |
-listenany | This command is used to wait for the virtual machine to get connected to any address. |
-launch | This command launches the debugged application immediately and doesn't wait for the run command. |
-listconnectors | This command is used to list the connectors available in the virtual machine. |
-help | This command displays a help message. |
-sourcepath | This command is used to search for the source path given by the user. If it is not specified, it takes the default directory's path. |
-connect | This command connects to a target virtual machine by specifying the connector's name. |
-dbgtrace | This command is used to print the information for the JDB |
-tserver | This command is used to run the application for the server in Java Hotspot Virtual Machine. |
-tclient | This command runs the application for the client in Java Hotspot Virtual Machine. |
-JOption | This command is used to pass the option to the Java Virtual Machine to run the JDB. |
JDB - Basic Commands
Basic commands used for debugging in Java are-
Column 1 | Column 2 |
---|---|
help or ? | This command is used to display a list of various commands with a brief description. |
thread | This command selects a thread as the current thread. |
threads | This command is used to display the threads that are running currently. |
run | This command is used to start the application to debug it. |
cont | This command is used to continue debugging an existing application after the breakpoint, step or pause. |
This command is used to print the value of data types. | |
dump | This command is used to print the current value of each field in the object. This command is identical to the print command for primitive data types. |
where | This command is used to dump the current thread's stack. |
Breakpoints
A breakpoint is an intentional stopping or pausing at a place in a program put in place for debugging purposes. It is also simply referred to as a pause. We can set breakpoints in JDB at line numbers or the first instruction of a method.
Example-
- stop at ClassName:19 (Used for setting the breakpoint at the first instruction for line 19 of the file containing ClassName)
- stop in java.lang.String.charAt (Used for setting the breakpoint at the beginning of the method java.lang.String.charAt)
We can use the command clear to remove a breakpoint from the class.
Example-
- Save the file as Main.java and now debug it.
- To start a session on JDB, we use the following command on our Main class -
- Now we will set a breakpoint on our main method.
- Now the following command will start the execution-
Stepping
Stepping is an action that tells the debugger to step over the next line. The step command advances the execution to the next line even though it is in the called method or current stack frame. The next command advances the execution to the next line in the current stack frame.
Exceptions
Exceptions occur when the code does not have an exception-handling mechanism. In this case, the code throws the thread's call stack.
Command-Line Options
When JDB is used instead of the command line option, other options that are provided by the user are also accepted.
Options Forwarded to Debuggee Process
- -v -verbose - This command turns on verbose mode.
- -D<name>=<value> - This command is used to set a system property.
- -classpath- This command lists directories in which to look for classes.
- -X<option>- This command is used to target Virtual Machine.
Conclusion
- The Java Debugger, also known as JDB, is used to find and fix bugs in the Java program.
- Debugging is the process of finding and removing the bugs in the code. In short, it means eliminating the bugs(de-bug).
- The Java debugger is a tool for debugging programs on the command line and fixing bugs in the Java Debug Interface(JDI).
- JDB has three units- Java Debug Wiring Pool (JDWP), Java Debugger Interface (JDI), and Java Virtual Machine Tool Interface (JVM TI).
- A breakpoint is an intentional stopping or pausing at a place in a program, put in place for debugging purposes. It is also simply referred to as a pause.
- Stepping is an action that tells the debugger to step over the following line.
- Exceptions occur when the code does not have an exception-handling mechanism. In this case, the code throws the thread's call stack.