isalnum() function in C Language
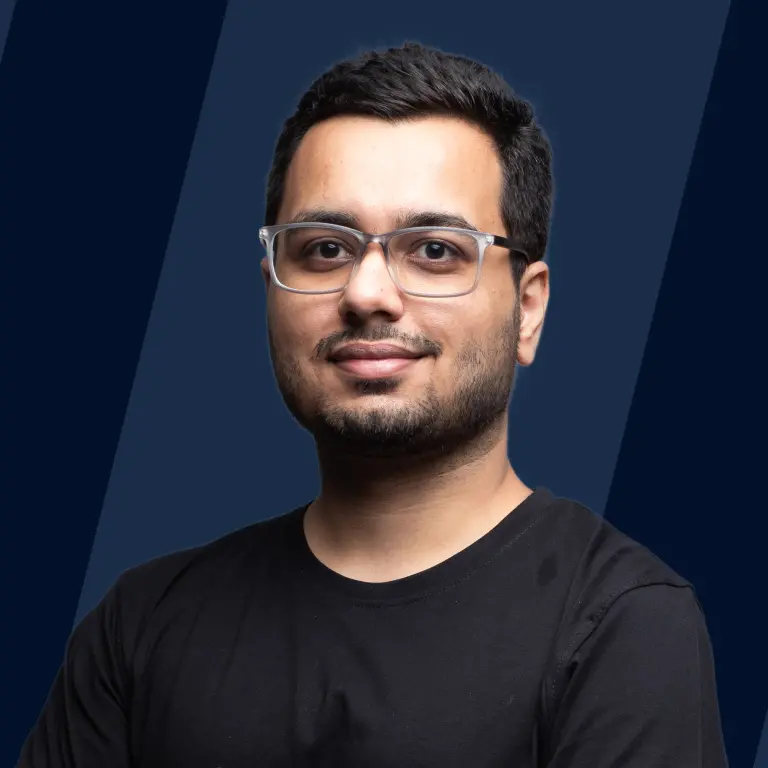
Overview
The C library function isalnum() checks whether a character is alphanumeric (i.e., either alphabet or numeric digit) or not.
Syntax of isalnum() in C
The syntax of the isalnum() function in C language is:
Where the isalnum() checks if the given character is alphanumeric or not, as classified by the current C locale.
In the default locale, the following characters are alphanumeric:
- digits (0123456789)
- uppercase letters (ABCDEFGHIJKLMNOPQRSTUVWXYZ)
- lowercase letters (abcdefghijklmnopqrstuvwxyz).
Parameters of isalnum() in C
The isalnum takes only one parameter, ch.
- ch is the character that needs to be checked.
Return Values of isalnum() in C
Return Type: int
- It returns a non zero value (i.e., true), if the given argument is either an alphabet or a digit (i.e., alphanumeric).
- It returns zero (i.e., false), if the given argument is neither an alphabet nor a digit.
Exceptions of isalnum() in C
The behavior of isalnum() is undefined if the value of ch is not representable as unsigned char and is not equal to EOF.
Example to Check a Character is Alphanumeric or Not
Output
What is isalnum() in C?
The <ctype.h> header file declares several functions for classifying and transforming individual characters in the C language. The isalnum() function is among one of them.
The isalnum() function takes one argument and classifies if the argument is alphanumeric or not. The result is true if the given character is either an uppercase letter or lowercase letter, or a decimal digit.
Note:
- If you look carefully, isalnum() takes an int argument, not a char. Functions like isalnum() take int arguments because functions like fgetc return int values, allowing special values like EOF to exist.
- You do not need to convert the char to an int before passing it to isalnum(). The compiler will implicitly convert the char to an int before passing it.
More Examples
Example 1: To check a character is alphanumeric or not
Output
Explanation
This is the usual behaviour of the isalnum() function in c. It will return non-zero for alphanumeric characters and zero otherwise. The non-zero value can differ from compiler to compiler, but all of them denotes true.
Example 2: Counting alphanumeric characters in a string
Output
Explanation
Using the while loop, we are iterating over all the characters in the character array one by one. Within that, we check whether the character is alphanumeric and increases the counter accordingly. This will give the total number of the alphanumeric characters in the array, which is 18.
Conclusion
- The isalnum() function is declared in ctype.h header file.
- The isalnum() function of C checks if a given character is alphanumeric or not.
- It returns non-zero value for alphanumeric characters and returns zero otherwise.
- The compiler will implicity convert the given char to an int before passing it to the isalnum().