isdigit() in C++
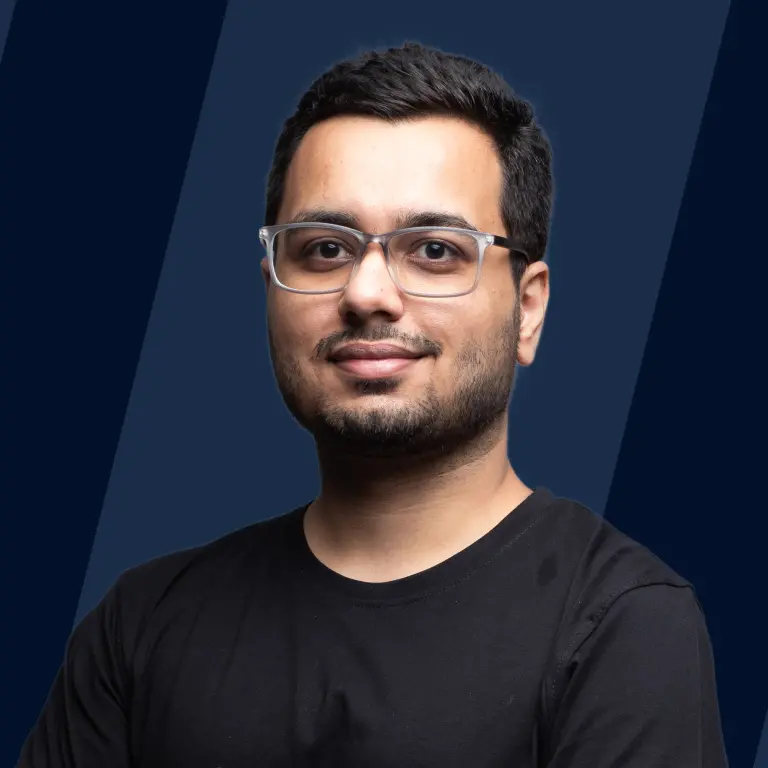
The isdigit() function, declared in the <cctype> header file in C++, determines whether the provided character represents a decimal digit or not. It returns 1(non-zero) if the provided character is a digit, 0 otherwise.
Syntax of isdigit() in C++
The syntax of the isdigit() function is given below,
Parameters of isdigit() in C++
It accepts only one parameter of the int data type.
Generally, when we pass a character as a parameter, but because of the int data type of argument, implicit type conversion occurs from char to int, i.e., a character is converted to its ASCII value.
Return Value of isdigit() in C++
The isdigit() function returns either 0 or 1 for false and true case respectively.
Example of isdigit() Function
Code: Let's understand the isdigit() function with an example code:
Output:
Explanation:
- We have passed 9 to the function isdigit() in C++, so it will be implicitly converted to the integer type, which is nothing but the ASCII value of 9.
- Then, as we know, 9 is a decimal digit, and the isdigit() function is expected to return 1.
- The same process will be followed for c. As it is not a decimal digit, 0 will be returned.
Exceptions of isdigit() in C++
This function doesn't throw any exceptions. However, it throws an error if we try to pass an inappropriate data type variable that can not be implicitly converted to int.
What is isdigit() in C++ ?
The cctype standard library consists of several functions to classify the characters, isdigit() is also one of them, which classifies between numeric digits and non-digits.
It accepts an integer and returns 1 if the ASCII value corresponding to that integer is a decimal digit or can say the ASCII Value is lying between 48 to 57(for '0' to '9'). If the ASCII value doesn't represent a decimal, it returns 0.
Usually, we pass characters to this function, which implicitly gets converted to an integer because the data type of argument is int.
If a different data type is passed, i.e., float, double etc., then the function will only run if the implicit conversion from that particular data type to int is possible. Otherwise, an error stating `"no matching function for call" will be thrown.
Undefined Behaviour of isdigit() in C++
The function isdigit() in C++ shows an undefined behavior if the provided value can't be represented as an unsigned char or is not equal to EOF.
To avoid this issue, we can pass the character after type casting to unsigned char,
More Examples
1. Program to Demonstrate the Function isdigit in C++
Input - 1 :
Output - 1 :
Input - 2 :
Output - 2 :
Explanation:
- Initially, we declared a character variable to store user input.
- Subsequently,y we check whether the entered character is a digit. Based on this, an appropriate message is being printed to stdout.
2. Simple Usecase of the Function isdigit in C++
Counting/Printing digits appeared in the string.
Input:
Output:
Explanation:
- We have created a string variable to receive input from stdin.
- Later, we loop over that string to determine which characters are digits.
Conclusion
- isdigit() function is used to check whether the character represents a decimal digit or not.
- It is declared in the <cctype> header.
- The ASCII Value of '0' is 48 and '9' is 57, so if the ASCII value lies in this range, the function returns true.
- The function shows an undefined behavior if the provided value can't be represented as an unsigned char.