isNumber() Function in JavaScript
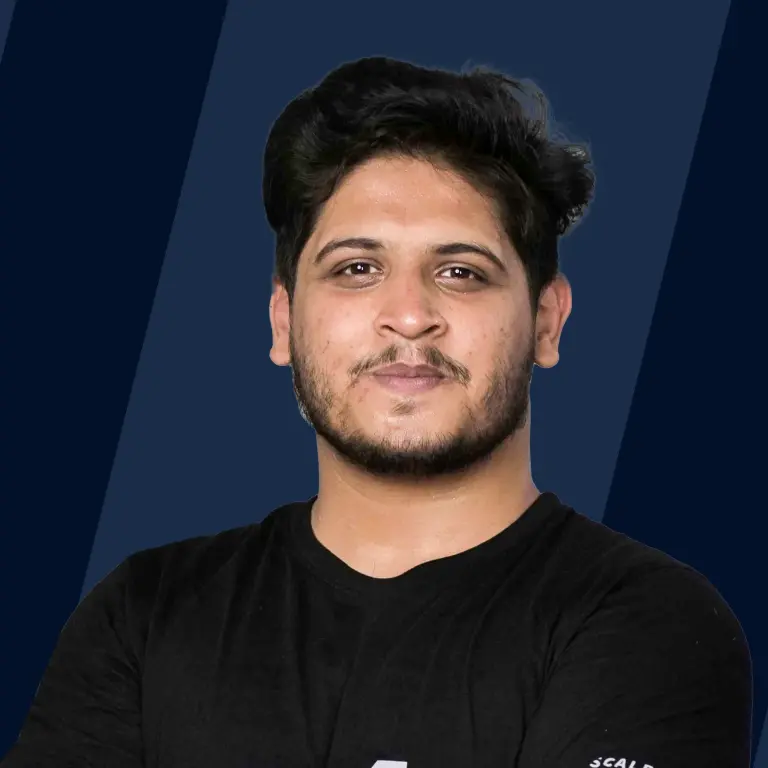
The _.isNumber() function used in javascript is a very useful tool for validating numeric data. This function helps to determine whether a given value is of the numeric data type. The '_' denotes that the function is part of the Lodash library, which provides multiple utility functions for tasks like array manipulation, object iteration, and data validation. The isNumber() function is used to ensure that the application receives the expected data type, reducing the risk of errors and enhancing the overall user experience. Let us explore the syntax, parameters, return values, and examples of the isnumber javascript function.
Syntax
The syntax of the isNumber() function is:
Parameter
- The _ (Underscore) character is a common convention to represent the Lodash library. It simplifies the usage of Lodash functions by providing a simple namespace.
- The value parameter is where you input the variable or expression that you want to check for numeric data type. It can be any valid JavaScript value – a variable, a literal, or an expression.
For using the underscore character (_) in the function, you need to ensure that the Lodash library is properly included in their project. This can be achieved in two ways:
-
By including the Lodash library through a script tag in an HTML file.
-
Using a package manager like npm for installing lodash as a dependency on your project.
Once included, you can use the underscore character (_) as an alias for the Lodash library, making the _.isNumber() function accessible for type-checking numeric values in your JavaScript code.
Return Values
The return values are boolean:
- true if the given 'value' is of the numeric data type.
- false if the given 'value' is not of the numeric data type.
The function internally performs a type-checking mechanism to determine if the given value is a number. The function utilizes the inherent JavaScript functionality to ascertain whether the provided value falls under the numeric data type. Here is the process of how the checking is done:
- The function uses the typeof operator, a built-in JavaScript operator, to check the type of the given value. If the result of typeof value is equal to number, the function returns true, indicating that the value is indeed a number.
- The function may also utilize the isNaN() function to handle numeric strings. If the value is a string that represents a valid number, isNaN() returns false, saying that the value is numeric.
By combining these checks, the _.isNumber() function ensures a correct assessment of the provided value, allowing developers to reliably determine whether the given value is of numeric data type.
Examples
Let's explore some examples of the isnumber javascript function:
Explanation:
- The numberValue is a numeric variable with the value 42. The function returns true since it is a valid number.
- The stringValue is a string with the value '321'. Even though the value represents a number, the function returns false because the actual type is a string.
- The arrayValue is an array and as the isnumber javascript function specifically checks for numbers, it returns false for an array.
- The functionValue is a function that returns a value. Since it's not a numeric value, the function returns false.
Let us explore some more data types and their respective outputs when passed into the _.isNumber() function.
Explanation:
- The decimalValue is a float variable. The function correctly identifies it as a numeric value and returns true.
- The booleanValue is a boolean value with the value true. Since it's not a numeric value, the function returns false.
- The bigIntValue is a BigInt variable. Although it represents a numeric value, the function distinguishes it from standard numbers and returns false.
- The symbolValue is a symbol type variable. As symbols are not numeric values, the function returns false.
- The isnumber javascript function considers NaN and Infinity as non-numeric values and returns false for both NaN and Infinity.
The Lodash _.isNumber() function is designed to identify both primitive number values and JavaScript Number objects as numerical values. This is an important property as it allows developers to seamlessly check for numeric data regardless of its representation.
Example:
Explanation:
In this example, a JavaScript Number object n is created and its type is checked using the typeof function. Despite being an object, the Lodash _.isNumber() function correctly identifies n as a numerical value. This property of isnumber javascript function is very useful because, in JavaScript, Number objects are distinct from primitive number values, and direct comparison using traditional methods might lead to unexpected results.
The _.isNumber() function also works for hexadecimal, binary and octal numeric literals.
- Hexadecimal literals, prefixed with '0x' or '0X', are recognized as valid numeric values.
- Numeric values represented in binary format with the '0b' or '0B' prefix are also considered valid.
- Octal numeric literals that are represented with the prefix '0o' or '0O' are also considered valid.
Some real-life examples for using the _.isNumber() are:
-
Validating user forms for input data in web development to ensure that the provided input is a valid numeric value before further processing. This helps in mainting data integrity and preventing errors.
-
When handling data from external sources, such as API payloads, it's essential to validate the data before usage
FAQs
Q. How does _.isNumber() handle NaN (Not a Number) and Infinity values?
A. The _.isNumber() function treats NaN and Infinity as non-numeric values, returning false for both cases. If your use case involves specifically checking for NaN or Infinity, it's recommended to use the isNaN() or isFinite() functions instead.
Q. Does the _.isNumber() function recognize numeric values within nested arrays or objects?
A. No, the function does not perform deep checking within nested arrays or objects. When dealing with nested structures, we should manually apply the _.isNumber() function to each value.
Q. Is there a performance impact when using the isnumber javascript function extensively?
A. While the _.isNumber() function is efficient, using it extensively, especially in performance-critical scenarios, may have an impact on speed.
Conclusion
- The _.isNumber() function in Lodash serves as a concise and efficient method for checking if a given value is of the numeric data type in JavaScript.
- Its syntax takes a single parameter representing the variable or expression that needs to be checked.
- The isnumber javascript function returns a boolean value, true if the input is a number, and false otherwise.
- The function can distinguish between primitive number types and JavaScript Number objects.