iter() in Python | Python iter() Method
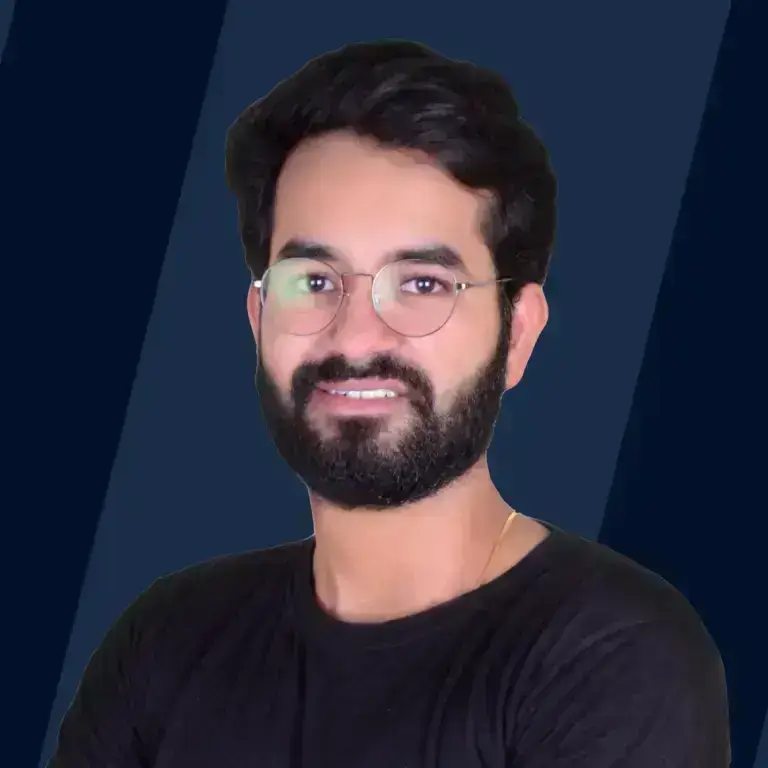
Overview
We use iter in python to convert an iterable object into an iterator. The python iter method creates and returns an iterator object. The returned iterator-object can be iterated one at a time using different loops in Python Programming.
Syntax of iter() in Python
The syntax of the method, iter in python, is very simple:
The object parameter is required, but the sentinel parameter is optional.
Parameters of iter() in Python
The method iter in python takes two parameters in which one of the parameters is optional, and the other one is required. The parameters provided in the iter method in python are:
Object
The object parameter is necessary. The object parameter contains an object to be converted into an iterable object. The object can be sets, tuples, lists, or user-defined objects.
Sentinel
The sentinel parameter is optional. The sentinel value is a special value that stores the end of the sequence. The sentinel value becomes useful when we call the iterable object. When the object is called, the iteration will stop when the returned value is the same as the sentinel value.
Note:
- If the sentinel value is not provided as a second parameter, then the first parameter must be a collection object. The collection objects are the object which has __iter__() method or __getitem__() method implemented in it.
- If the sentinel value is provided as a second parameter, then the first parameter must be a callable object. In callable objects, the next() method returns the next value.
Return Value of iter() in Python
The iter() in python returns an iterable object of the parameterized object. We can use this iterable object to traverse the values stored inside the object.
Exception of iter() in Python
If the object provided as parameter is a user-defined object (non-primitive type object) then the object must implement __iter__() method and __next__() or __getitem__() method otherwise the TypeError exception is raised by the iter in python.
While dealing with the iter in python, we will encounter another exception called StopIteration. The StopIteration exception is a built-in exception raised by the built-in function next() and an iterator's __next__() method, which resembles that the iterator is exhausted or the iterator produces no further items.
Refer to this article's topic - More Example, to know more about the exceptions.
Example
Let us take an example of a list having country names. We can convert the list into an iterator object (an object having a sequence of values) using iter in python.
:::{.tip} Note:
We can use the next() method of python, which returns the next item in the iterator until there are no items left.
:::
Code:
Output:
What is iter() in Python?
When the size of the container is not known in advance, or the object is not iterable, iter in python is used.
The iter in python is a method that can be used to convert a collection of data or a non-iterable object into an iterable object. The python iter() method creates an iterable object from the parametrized object (by implementing the __iter__() method for the object in the background) and then returns the iterator object. We can further use loops like for and while to iterate over or loop over the returned iterable object.
The python iter() method takes two parameters, namely - object (that is to be converted into an iterable object) and sentinel value (a special value that denotes the end of the sequence). The first parameter, i.e., the object, is a required parameter, but the sentinel value is optional.
Using the parameterized object, the iter method generates an iterable object and returns the same. If the sentinel value is provided, then the iter() method returns values from the object (or an iterator) until the sentinel value is not reached.
Examples of iter() in Python
Let us take examples better to understand the python iter() method.
Example 1: Python iterate list
Let us convert a list of numbers into an iterable object using iter in python:
Code:
Output:
Example 2: Python iterate list with index
Let us convert a list of numbers into an iterable object using iter in python. A for loop can be used to find the index of the elements.
Code:
Output:
Example 3: iter() for custom objects
Let us create custom class Numbers. We can convert the object of this Numbers class into an iterable object using the iter() method in python.
Code:
Output:
Example 4: iter() with sentinel parameter
Let us create a custom class TwiceNumber which generates a number that is twice its previous number; for example, we will start with 2, then the first number will be 2, the second number will be 4 , the third number will be 8 , and so on. So, the sequence formed will be: 2, 4, 8, 16, 32, 64,....
We can convert the object of this TwiceNumber class into an iterable object using the iter() method in python. We can also specify the sentinel value (say 32). So, the class will generate the sequence of the doubled numbers until 32 is reached.
Code:
Output:
Example 5: TypeError Exception
If the object provided as parameter is a user-defined object then the object must implement __iter__() method and __next__() or __getitem__() method otherwise the TypeError exception is raised by the iter in python. Let us take an example to visualize the error. Let us create custom class Numbers. We can convert the object of this Numbers class into an iterable object using the iter() method in python.
Code:
Output:
Example 6: StopIteration Exception
The StopIteration exception is a built-in exception raised by the built-in function next() and an iterator's __next__() method, which resembles that the iterator is exhausted or there are no further items produced by the iterator.
Let us take an example to visualize the error. Let's take an example to see the exception raised by the python interpreter when we try to get the next value of the exhausted iterable object.
Code:
Output:
Conclusion
- To convert an iterable object into an iterator, we use iter() in python. The python iter method creates and returns an iterator object.
- The syntax of the method iter in python is: iter(object, sentinel). The object parameter is required, but the sentinel parameter is optional.
- The sentinel value is a special value that stores the end of the sequence.
- If the sentinel value is provided, then the iter() method returns an iterator until the sentinel value is not reached.
- If the object provided as parameter is a user-defined object then the object must implement __iter__() method and __next__() or __getitem__() method otherwise the TypeError exception is raised by the iter in python.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.