Difference between Iterable and Iterator in Python
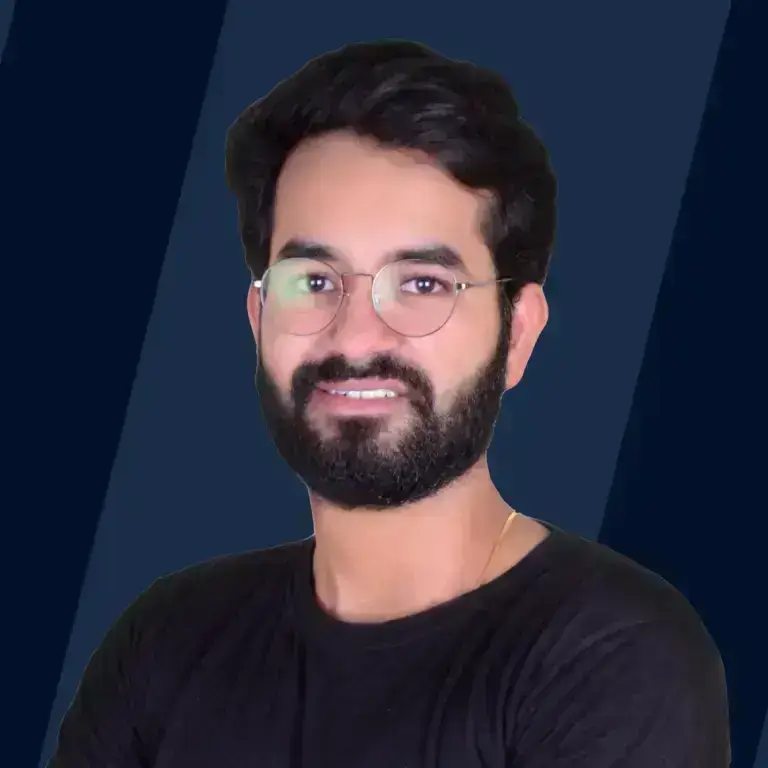
Iterable objects or iterables are the objects we can iterate over. Usually a for loop or simply a loop is used for iteration. Built-in containers such as lists, tuples, dictionaries, sets, etc. are called pure iterables as they hold the data themselves. Iterators are also called iterables as we can iterate over them, though they do not hold data with themselves as pure iterables.
What are Iterables in Python?
Before getting into iterables let us first understand iteration.
Iteration is defined as the repetition of the same process until some condition is satisfied. This repetition can be on the same object or sequence of objects. For example, if we wish to print hello 50 times, we will write a for loop as follows:
Iterables are the objects in Python that let us perform iteration. Iterables return all its members one at a time. A very general example of iterables is a list. We use a simple for loop to traverse to get each of the list's members and print in the output. This process is known as iteration over a list.
Sequence types in Python such as lists, strings, tuples, sets, etc. are iterables. Other examples include non-sequence types such as dictionaries, file objects, and objects of custom classes defined with an __iter__() method or __getitem__() method implementing sequence semantics.
Iterators are the objects that represent a stream of data. Iterators implement both the __iter__() and __next__() methods. Iterators facilitate iteration over iterables. The __iter__() method in Python returns an iterator object for iterables. For example, we pass a list to the iter() method and it returns a fresh new iterator. This iterator then returns members of the list one by one.
The __iter__() method is part of iterator protocol along with the __next__() method. Formally, the objects of built-in class or custom class, that implement the __iter__() method or the __getitem__() method which is part of sequence protocol are all called iterables.
Identifying Iterables in Python
An object can be called iterable if it can provide an iterator for itself. We know that iterables implement the __iter__() method. Thus, if the object supports this method, we can say that it is iterable.
There are several methods to check if the object is iterable or not.
For Loop Method
We can use the for loop to loop through iterables. Non-iterable throw a TypeError. The try-except block can be implemented to check if the object is iterable.
In the below example, we are using a list and an integer. As we know that list is iterable but integer is not.
Output:
Using hasattr Method
We can use the hasattr() method to check if the object has implemented the __iter__() method or not.
Output:
Using the Iterable class of collections.abc module
All the collections are instances of the Iterable class. We can check if the class of the given object is an instance of the Iterable class or not using the instance () method.
Output:
Understanding Python Iterators
Iterators are the objects in Python that allow iterations over collections of data such as lists, tuples, dictionaries, sets, file objects, etc.
Iterators are also iterables as we can iterate through them. But not all iterables are iterators.
Iterators return the data from a stream or container one element at a time. They also keep track of current elements and visited elements.
Iterators are well-established hidden structures implementing iterator protocol. To learn more about iterators and iterator protocol visit here.
Iterators can be used to get each data item, transform each data item, or generate new data items using an existing sequence of data.
The for loop for iterables uses the built-in iter() function to get the iterator for the stream of data.
What is the Relationship between Iterators and Iterables?
We have seen iterators and iterables so far and it is okay to make mistakes in these as they are complex topics to understand and we can improve our understanding with practice.
Iterators implement the __iter__() that usually returns self and __next__() method that returns an item on each call. Iterables have an __iter__() method that returns items one at a time whenever called.
Considering this we can say that all iterators are iterables as they satisfy the iterable protocol (implements __iter__() method). But we cannot say that all iterables are iterators. Iterables that implement the __next__() method are iterators only.
Let us take an example of the __next__() method with iterables as well as iterators.
Output:
On calling the __next__() method with the iterables, it throws the TypeError. This is because pure iterables don't implement the __next__() method but they provide a special method next() function which is called internally to retrieve data. But these are not iterators.
Distinguishing between Iterators and Iterables
The __iter__() method is not associated with the iterator or the iterables. It shows different behavior for both. In the case of iterators, it returns self and must implement the __next__() method whereas, in the case of iterables, it returns the iterator object that returns elements when called.
In the case of custom classes, it is not advised to make an object iterable and iterator at the same time.
The __iter__() method as mentioned above returns self. This implies it doesn't ensure that it will return a new object each time. In contrast, the __iter__() method in iterables always returns a new object every time called.
Pure iterators are more efficient as they don't hold any data with them. They produce the data on demand. Pure iterables hold the data themselves.
Feature | Iterators | Iterables |
---|---|---|
For loops can be applied directly | Yes | No |
Can be iterated over multiple times | No | Yes |
supports the iter() method | Yes | Yes |
supports the next() method | Yes | No |
Keeps information on the state of iteration | Yes | No |
Is optimal in memory usage | Yes | No |
Practical Examples
Example 1 - Read existing Data
Let us take a simple iteration example using the iter method and next method for a list.
Output
The iter method returns an iterator for the fruits list. The next method gives the next element from the list.
Example 2 - Generating new Data
Output:
In the example above we are using a list of data to generate new data. For each number, we square it and store it in the squared_numbers.
Example 3 - Transform Data
Output:
In the list given, we are transforming each element in the list. For loop internally uses the iter method and lets us transform the list.
Conclusion
- Iterable objects are the objects that let us perform iteration on them. Iterators facilitate this iteration. Lists, Strings, Dictionaries, etc. are called pure iterables.
- The __iter__() method returns self for iterator. But it returns the iterator in the case of iterables.__iter__() method.
- Iterators are iterables but the opposite may not be true.
- For loop can be used to iterate over objects. It internally uses the __iter__() method.
- The iterable protocol is implemented by iterators. This protocol consists of the methods __iter__() and __next__().
- To identify iterables in Python we can use simple for loop, the hasattr() method, or the Iterable present in Collections.