Python Program to Iterate through Dictionary
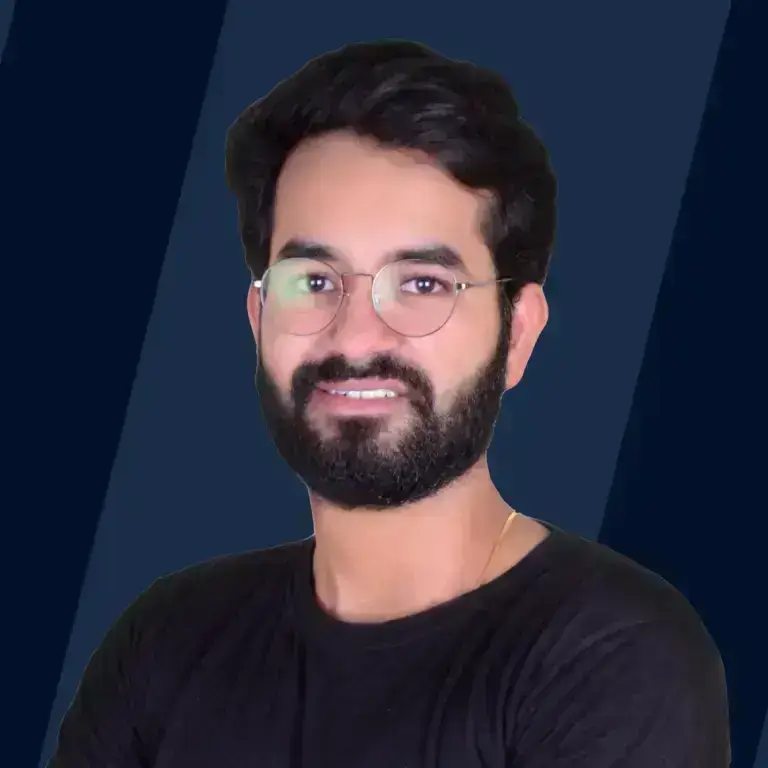
Overview
While interacting with dictionaries in python, there might be scenarios where iterating through dictionary python was a part of your code. Dictionaries can be defined as associative arrays where we store the data elements as key: value pairs and iterating through them with twelve methods is the objective of this article. With the below module, we shall learn iterating through the dictionary python with code examples and elaborative explanations to understand the topic linked.
Introduction
We might come across scenarios where iterating through dictionary python is required. For example, when we want to create a program for an organization at will show an employee's name, contact number, department, and other relevant information then we might want to implement iterating through the dictionary python for storing the data so that the organization can display it after running the program.
We define the dictionaries in python as a collection where data is stored in a key-value format which means that for each value there is an assigned key related to that which can be used to reference the specific value.
Below shows what a dictionary in python looks like:
In the dictionary, the words on the left side represent the 'keys' which are all formatted as strings. Separated by colons (:), we have the 'values' corresponding to the key. Now for iterating through dictionary python we have severable ways that python allows us to do the same. Let us jump into some scenarios with code examples and descriptive explanations to understand the concepts carousel better.
Python Program to Iterate Through Dictionary
Moving forward we shall understand how we can make use of the different methods that python has to provide for iterating through dictionary python by discussing a few scenarios where we shall expand our knowledge and learn iterating through dictionary python with code examples and elaborative explanations as well.
Method 1: Through Keys Directly Using For Loop
We must know that dictionaries are iterable objects, which means iterating through dictionary python like any other object. Well the easiest way to do so, has to be by using the for loop in python. With the for loop, we can run through each value of the dictionary individually.
Syntax:
The syntax used in the method - Through Keys Directly Using For Loop for iterating through dictionary python is shown below:
With the below code example also we are using the for loop to iterate through the dictionary python and printing each key and its values as shown. We start by declaring a dictionary named 'employee ' with the key and its associated values which we then print one by one by using the for loop for iterating through the dictionary python.
Code:
Output:
Explanation:
As seen above, we start by declaring a variable called 'employee' which stores four keys and their values. The variable is storing a value in the dictionary data type. We printed the dictionary as well in the output. Then, we start declaring a for loop that will help to iterate through each value in the employee dictionary. This for loop will print both the key and its associated value as the output.
Method 2: Through .items()
Whenever we have to deal with a scenario where we are iterating through a dictionary python and want the output values to appear as a list of tuples, we can make use of the item() function for the same. By using the dictionary.items() function we start by converting each key-value pair into a tuple. Then by using the for loop and the items() function we can start iterating through the dictionary python over all the keys and their associated values.
Syntax:
The syntax used in the method - Through .items() for iterating through dictionary python is shown below:
In the below example, we declared a dictionary with key: value pairs. Then we made use of the for loop with the items() function to iterate over the dictionary and print the values as a tuple.
Code:
Output:
Explanation:
As seen above, we start by declaring a variable called 'employee' which stores four keys and their values. The variable is storing a value in the dictionary data type. We printed the dictionary as well in the output. Then, we start declaring a for loop that iterates through each value in the 'employee' dictionary using the items() function. The items() function converts each key-value pair into a tuple, that is used to access the loop. As seen, each key-value pair is printed as a tuple in the output.
Method 3: Through .keys()
Many times we encounter scenarios where we only want to iterate through the keys of the dictionary. For example, a scenario like compiling the list of information that the company stores for its employee or even we can say the metadata of the dictionary. Then, this dictionary.keys() function comes in very handy. We can then make use of the keys() function in python to get the list of all keys and eventually print them to the console.
Syntax:
The syntax used in the method - Through .keys() for iterating through dictionary python is shown below:
For the code example given below, we are declaring the dictionary we make use of the for loop with the keys() function to search for all the keys of the 'employee ' dictionary. With the for loop we iterate through each key of the 'employee' dictionary and print them as an output. In the output, we see all four keys have been returned.
Code:
Output:
Explanation: As seen above, we start by declaring a variable called 'employee' which stores four keys and their values. After printing the dictionary, we start declaring a for loop that iterates through each value in the 'employee' dictionary using the keys() function. The keys() function in the python dictionary get the list of all the keys and eventually prints them to the console. As a result, all four keys get printed in the output.
Method 4: Through .values()
Sometimes we might want to gather all the values of each column from the dictionary from a specific dictionary. To achieve so, we use the dictionary.values() function to get the list of all values and eventually print them to the console.
Syntax:
The syntax used in the method - Through .values() for iterating through dictionary python is shown below:
For the code example given below, we are declaring the dictionary called 'employee'. We make use of the for loop with the values() function to search for all the values from the 'employee' dictionary for a specific employee. The for loop then iterates through each value of the 'employee' dictionary and prints all the associated values as an output.
Code:
Output:
Explanation:
As seen above, we start by declaring a dictionary called 'employee' which stores four keys and their values. After printing the dictionary, we start declaring a for loop that iterates through each value in the employee dictionary using the values() function. The values() function is used to get the list of all the values and eventually print them to the console. As a result, all four values get printed in the output.
Method 5: Iterating in Sorted Order
In scenarios where we want our key: value pairs to be in a sorted manner while we are iterating through dictionary python, we should always go with the sorted() function. The sorted() function is always used when we want to return the list with its associated values in a sorted manner.
Syntax:
The syntax used in the method - Iterating in Sorted Order for iterating through dictionary python is shown as below:
Below the code example, we declare a dictionary with a few keys
Code:
Output:
Explanation: As seen above, we start by declaring a variable called 'employee' which stores four keys and their values. We then sort the keys by using the sorted(dictionary.keys()) method which then returns all the keys available by implementing the for loop in that 'employee' dictionary. We then use the sorted(dictionary.values()) method to sort all the values similarly.
Method 6: Iterating Destructively With .popitem()
We might come across a scenario where we want to destructively iterate and delete items sequentially while iterating through dictionary python. Well, to implement this so we can make use of the dictionary.popitem() method to remove the items from the dictionary and return all the arbitrary key-value pairs from that dictionary. While you might raise a 'KeyError exception' when you call the dictionary.popitem() on the empty dictionary or when all the items from the dictionary have been removed.
Syntax:
The syntax used in the method - Iterating Destructively With .popitem() for iterating through dictionary python is shown below:
For the below code, we used the while loop expression over a for loop as its always safe to iterate through the dictionary when we don't want to modify it but here as we want to delete items from the dictionary and modify it in that way we used the while loop. We also implemented the try...except block to catch an error like the KeyError which is raised by when the items in the dictionary are 0 while we are popping out the item from the dictionary one by one in each iteration. The dictionary.popitem() sequentially removes the items from the dictionary and the loop break when the item goes to 0 giving us the KeyError exception.
Code:
Output:
Explanation: As seen above, we start by declaring a variable called employee which stores four keys and their values. We used the while loop expression as we want to delete items from the dictionary and modify them while iterating through the dictionary python. We also implemented the try...except block to catch the KeyError exception which gets raised when the items in the dictionary are zero while we are popping out the item from the dictionary one by one in each iteration. The dictionary.popitem() sequentially removes the items from the dictionary and the loop break when the item goes to 0 giving us the KeyError exception.
Method 7: Using Some of Python’s Built-In Functions
In python, we have some 'built-in functions' that can also be used to iterate through throthe ugh dictionary python. These functions can be considered iteration tools via which we can start iterating through the dictionary python.
The two built-in function in python that we shall be seeing in the below code example are the map() function and the filter() function.
Using map() function:
The map() function in python can be described as a map(function, iterable, ...) that returns an iterator that is applied to every element of the iterable which yields the output. therefore, we can consider this map function as an iteration tool that can be used for iterating through the dictionary python.
Syntax:
The syntax used in the method - U thesing map() function for iterating through dictionary python is shown as below:
With the below code example, we are having certain prices of fruits assigned as their values. If we want to update the prices of the same 'fruit' dictionary we can start by defining a user-defined function that updates the prices that are used as its first argument to map() while the Fruits_Original.items() is considered as the second argument. We used the map() function to iterate through the elements of the dictionary and updated the prices for each fruit by using this user-defined function 'updates' that we created. Using the dict() method we generated the new_prices dictionary that is returned after the map() function from the iterator.
Code:
Output:
Explanation: In the above example, we start by declaring a Fruits_Original dictionary containing the prices of the fruits. We then make use of the user-defined function updates that updates the prices that are used as its first argument to map() while the Fruits_Original.items() is considered as the second argument. By using the map() function we iterate through the elements of the dictionary and updated the prices for each fruit. Using the dict() method we generated the new_prices dictionary that is returned after the map() function from the iterator.
Point to note, the user-defined function update() returns a tuple of (key, value) structure where we assign the key as the price[0] and round(price[1] * 0.60, as is the new updated value.
Using filter() function:
The filter() function in python is also one built-in function that can be implemented to iterate through the dictionary python and filter out some of its elements based on the filter. The filter() function in python can be described as a filter(function, iterable) that too returns an iterator from the items of iterable where the function holds - TRUE.
Syntax:
The syntax used in the method - Using the filter() function for iterating through the dictionary python is shown below:
In the below example, we are trying to find out all the fruits whose prices are more than 0.40. We created a user-defined function that after the condition is satisfied will be passed as the first argument to the filter() function while the Fruits_Original.keys() is passed as the second argument. We iterate the keys of prices with the help of the filter() function which filters() out all the keys that satisfy the condition for every key in the dictionary. Finally, as the filter() return an iterator we need that list object and hence we use the list() function to get the list of products with a high price.
Code:
Output:
Explanation: We used the user-defined function high_price_fruits to calculate the fruits which have high prices. We then made use of the filter() function to iterate over each fruit and its prices and filter out all the names of fruits whose prices are more than 0.40 and printed them out as a list as shown above. Finally, we used the list() function to capture all the fruits which have high prices as the filter() function returns an iterator, and returning a list object is a must.
Method 8: Using collections.ChainMap
The most useful module in python is the collections module that contains specialized container data types of which ChainMap is the most popular data type. Implementing ChainMap, we can join multiple dictionaries into a single and modifiable view.
Syntax:
The syntax used in the method - Using collections.ChainMap for iterating through dictionary python is shown as below:
With the below code example, we are implementing the ChainMap from the collections module to join two different dictionaries and create a single dictionary from it. After which we use the for loop for iterating through the dictionary python as shown below.
Code:
Output:
Explanation: Here we implemented the ChainMap function while iterating through the dictionary python. In this, we created two dictionaries which we connected via the ChainMap method and printed the same to look at how both the dictionaries get attached. Then we freely iterate through the chained_both_dict object using the for loop as we do for any dictionary iteration in python.
Quick Note: The ChainMap objects can also be used to implement dictionary .items(), dictionary.keys(), and dictionary.values() as any standard dictionary and we can implement these methods while iterating through dictionary python.
Method 9: Using itertools
In python, we also have the itertools module that helps while iterating through dictionary python in a cyclical and chained manner until all keys from the dictionary are exhausted. The two itertools that we would be studying are Cyclic Iteration With cycle() and Chained Iteration With chain().
Cyclic Iteration With cycle()
If you come across a scenario where you need to iterate through a dictionary repeatedly in a single loop then you can use make use of the itertools module in python. The cycle() function creates an iterator returning items from iterable and then saving each copy. But once the iterable gets exhausted, the cycle() function returns the items from that saved copy. And as this happens cyclically, it's in the user's hands to stop the cycle whenever wanted.
Syntax:
The syntax used in the method - Cyclic Iteration With cycle()for iterating through the dictionary python is shown as below:
With the below example, we shall be starting by importing the cycle module and iterating the elements in a dictionary four consecutive times. The iteration of the cycle can be as long as we want and hence we become responsible for it to stop. When the if condition breaks the cycle, the items_total count also goes down to zero.
Code:
Output:
Explanation: As seen above, we have started by importing the cycle module and proceeded to declare a dictionary with its associated key: value pairs. As we want our cycle to loop four times we specify the variable 'times' as four. Now we calculate the total items by multiplying the number of times by the length of the 'Fruits_Original_1' dictionary. Now we start the looping for each key by implementing for loop and implementing the cycle() function to it. As we are responsible for the cycle to stop after the fourth looping so when the if condition breaks the cycle, the items_total count also goes down to zero.
Chained Iteration with chain()
For scenarios where we need to iterate through multiple dictionaries in a chain we can use the chain(iterables) function. Here we get some iterables as arguments and eventually make an iterator that shall yield elements from the first iterable. Once exhausted then the iterable shall proceed with the next iterable and so on, until all are exhausted. This way helps to iterate over multiple dictionaries in a chain.
Syntax:
The syntax used in the method - Chained Iteration With chain() for iterating through the dictionary python is shown as below:
With the below code, we are using the chain(iterables) function which is imported from the itertools module to iterate through multiple dictionaries chain-lifelike manner. Once the iterable in the dictionary is exhausted the loop shall stop as shown below.
Code:
Output:
Explanation:
From the above example, we started by first importing the chain() function from the itertools module. We then created two dictionaries and feed them with a few keys: value pairs. Now to implement the chain() function we made use of the for loop to chain the two dictionaries and make some iterables as arguments that shall yield elements from the first iterable. Once that got exhausted it moved to second iterable and so on until all got exhausted.
Method 10: Using the Dictionary Unpacking Operator (**)
The dictionary unpacking operator (**) can be used to merge multiple dictionaries into one new dictionary.
Syntax:
The syntax used in the method - Cyclic Iteration With cycle()for iterating through dictionary python is shown as below:
When sca enariarisesse where we have two dictionaries - Fruits_Original_1, and Vegetables_Original_1, where we need to iterate through both of them together without implementing collections.ChainMap or itertools.chain(), then we can implement the dictionary unpacking operator (**) which helps to merge multiple dictionaries into one new dictionary. Once a new dictionary is created containing the key: value pairs from both dictionaries then we can swiftly iterate through it as usual as seen in the below code example.
Quick Note: When the dictionaries that we want to merge contain duplicate keys, then the values from the right-most dictionary will be picked up.
Code:
Output:
Explanation:
From the above example, we started by declaring two dictionaries - Fruits_Original_1, and Vegetables_Original_1 with their specific key: value pairs. After printing the dictionaries, we used the Unpacking Operator (**) to merge the two dictionaries into one. Finally, we used the for loop and items() functions in python for iterating through the dictionary python and printing the key
Method 11: Using the index of the items
For iterating through the dictionary python, we can also make use of the index of the items. To implement so, we use the for loop to iterate over each index in the dictionary and eventually printing out the index from the same.
Syntax:
The syntax used in the method - Using the index of the items for iterating through through dictionary python is shown below:
As seen below, we started by declaring a dictionary with key
Code:
Output:
Explanation:
We start by declaring a dictionary - 'Fruits_Original_1' where we add a few keys: and value pairs and we use the print syntax to print the dictionary as well. Then for iterating through the dictionary python, we use the for loop in python to print the key: value pairs by using the index of the items to print each key: value pair one by one.
Conclusion
-
Dictionaries in python as a collection where data is stored in a key-value format which means that for each value there is an assigned key that is used to reference the particular value.
-
While using the unpacking operator for iterating through dictionary python we must note that when the dictionaries that we want to merge contain duplicate keys, then the values from the right-most dictionary will be picked up.
-
When you call the dictionary.popitem() on the empty dictionary or when all the items from the dictionary have been removed then you might encounter a KeyError exception.
-
Whenever we have to deal with a scenario where we are iterating through a dictionary python and want the output values to appear as a list of tuples, we can make use of the item() function for the same.
Explore Scaler Topics Python Tutorial and enhance your Python skills with Reading Tracks and Challenges.