What is Java Async?
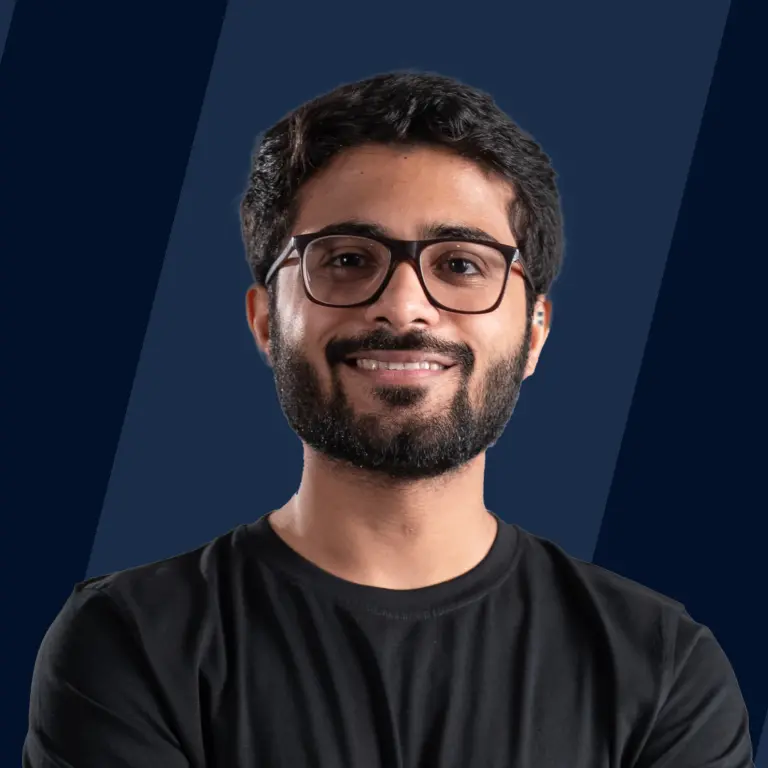
Parallel programming in the form of asynchronous programming enables a task to run independently of the main application thread. When the task is finished, it alerts the main thread (as well as whether the work was completed or failed). By leveraging several processors simultaneously, parallel programming enables developers to use multicore computers to speed up the performance of their applications. One or more threads can be used for synchronous (Sync) and asynchronous (Async) programming. The primary distinction between the two is the ability to execute numerous operations concurrently with asynchronous programming as opposed to synchronous programming, which can only handle one activity at a time.
Implementation of Asynchronous Callback
Asynchronous calls don't prevent the program from running its code. The call goes back to the callback function after returning from the event. Therefore, in the Java environment, we must create a new thread and call the callback method inside of it. Although it's not necessary, the callback function may be called from a thread. A Callback can create a new thread and become asynchronous by doing so.
In this code, we are going to check how to write an async callback in java.
Output
Code Explanation In the above code first, we created an interface called EventListener which has a method called dosomething() and class A implements this interface. We have created a class called Main which have a member variable called mEvenListener of type EventListener and a method to registerEventListener. Class Main also contains a method which is an async method called performoperation() which runs in a new Thread.
Working of Java Async
As there are numerous requirements for non-blocking code where async programming is necessary to execute the code asynchronously, Java has various options for developing non-blocking code, such as using threads, futures, CompletableFuture, etc. Let's talk about a few approaches to asynchronous programming, including thread, guava, completeableFutures, futuretasks, and ea async.
Async with Threads
In Java, a thread is the course or path followed while a program is being run. The main thread, which is typically present in all programs, is provided by the JVM, or Java Virtual Machine, at the beginning of the program's execution.
The Runnable interface and Thread class, which can be found in JDK 1.0, are the first methods for implementing async in Java. Any class can extend Thread and override the run() method by implementing Runnable, or vice versa. The run method will not start a new thread when called straight from a Runnable; instead, it will run on the thread that is calling. However, a new thread will be generated when we use thread.start(). We can locate Executors in JDK 1.5 for better thread management. They are utilized since there is no need to manually establish a thread and use various ThreadPools. Instead, we may tell it how many threads it can have, and it will continue to use those threads for as long as the application is running.
Example 1 In this example, we are going to see how to achieve async in java with threads.
Output
Code Explanation In the Above code, we have created a new Thread and in that thread called a function called factorial that calculates the factorial of a number.
Example 2
In this example, we are going to see how to return a Thread from a method and start it/ begin the execution of the thread.
Output
Code Explanation In the above code, we have created a method called getThread() that returns a Thread and we can start the Thread to get the results.
Async with Future
Run() is a void method that is unable to return any results from a thread. Instead, if we require the output of a calculation performed on a thread other than the main one, we must utilize the Callable interface. When a job is sent to an ExecutorService, Callable will optionally return a Future object because the result is not immediately available. When our operation is complete, we can access the result through this object by calling get() on Future. Given that get() blocks the active thread until the response is available, this is a poor use of async. However, there is a fix for this that involves utilizing the future. The isDone() method continuously checks to see if the calculation is finished, and only when it does, will get() return the outcome.
In this code we are going to see how to achieve async using Future.
Code Explanation
In the Above code, we have used the submit method of the ExecutorService to perform the task asynchronously and return the instance of the FutureTask. We have run a while loop to check if the task is done or not and when the task is done printed the results.
Async with CompletableFuture
The Future object was upgraded to a CompletableFuture object in JDK 1.8, which in addition to Future, also implements CompletionStage. Numerous methods are available in the CompletionStage for more straightforward use of the results computed in various threads and stages. The most popular ones include then apply(), which is akin to map() from Streams, and then accept(), which is akin to foreach. There are various ways to obtain a CompletableFuture response, some of which may or may not involve starting a new thread for the work, but one aspect and additional capability of Futures is the ability for users to handle exceptions that may arise during processing.
In this example, we are going to see how to use CompletableFuture to achieve async in java.
Output
Code Explanation In Above Code we have created CompletableFuture and called the supplyAsync() method to find the factorial of number asynchronously which return a CompletableFuture on which we have called the isDone() method to check if the task is Done or not.
Async with @Async
Using Spring Framework's @Async annotation is another technique to implement async. Users are only permitted to use it on public methods; they are not permitted to call methods from the class in which they were declared. Any function contained in a method with the Async annotation will run in a separate thread and can either return void or a CompletableFuture. This is a substitute for constructing a CompletableFuture and assigning it a function to execute, however in order to utilize this annotation, another thing is required: @EnableAsync on a configuration class.
In this example, we are going to see how to implement async in java using @async
Output
Code Explanation
In the Above code, we have used @Async notation on the public method asyncFactorial with return type by wrapping the fact in the CompletableFuture.
Async with EA Async
This is just another property of Java that makes building asynchronous code sequentially simple and scalable. Through the ea-async library, Electronic Arts received the async-await capability that is provided to the Java ecosystem. This feature rewrites the call to the await method, which functions similarly to the completableFuture, and converts the runtime code. As a result, we can use the EA-sync technique known as the await method to implement the aforementioned completeableFuture code by calling the Async.init function to initialize the Async runtime.
First, we'll add the latest ea-async Maven dependency to the pom.xml
Then we'll transform the previously discussed CompletableFuture code by using the await method provided by EA's Async class:
Code Explanation
Here, we call the static block's Async.init method to start the Async runtime instrumentation. At runtime, async instrumentation modifies the code and rewrites the await method call so that it behaves similarly to utilising a chain of CompletableFuture. Therefore, the call to the await method is similar to calling Future.join.
Spring Events
A step forward that provides decoupling and an easy approach to add new functionality without modifying the existing one is using Spring Events for async implementation. There are three things required:
Any object that implements ApplicationEvent can be used as an event. A publisher is a bean that will use the ApplicationEventPublisher bean to publish the event. A bean called a "listener" will have a method annotated with the annotation "@EventListener" and help define the action that should be taken when a certain event is received.
Microservices
At the microservices level, we can also have a synchronous or asynchronous communication. The difference between them is that, as stated in the definition, asynchronous means we don’t wait for an immediate response from the service we are calling, while synchronous means we wait for the response.
One of the most popular synchronous communications between microservices is done using REST calls. For asynchronous communication, we can use queues or topics. Both of them contain messages, but one difference would be that a message from a queue can be consumed only by one subscriber and a message from a topic can be read by multiple subscribers.
When to Use Java Async?
When the tasks are not dependent on each other and may take some time for execution we should use Asynchronous callbacks.
For example When you order your food other people can also order their food in the restaurant. They don’t have to wait for your order to finish, If you’re downloading a song from the internet, Getting an API response.
Advantages and Disadvantages of Java Async
Advantages
-
User Experience Asynchronous applications significantly enhance the user experience when compared to synchronous programming. An application's or website's scripts can all load simultaneously, improving responsiveness and reducing page load times. Additionally, the inability of one thread to run or render has no effect on the performance of other threads. As a result, asynchronous apps generally have higher availability.
-
Customization Writing callback methods in response to potential faults that could arise and cause a program to fail is a practical need of asynchronous programming. These callbacks instruct the system to proceed with the subsequent task and ignore the error. Additionally, it allows programmers to create unique error messages. Errors do not necessarily mean that your software will not execute at all. While all the other functions of the application function perfectly, users receive a pleasant note.
-
Scalability Many programming languages use the well-known async/await syntactic structure, which makes it easy for asynchronous functions to interact with promises. Objects called promises to contain the desired behavior of an asynchronous action. Scalability is a concept that depends on both of these ideas. There are two different ways that scalability can occur: laterally and vertically. By adding more servers, both synchronous and asynchronous systems can benefit from horizontal scaling. On the other hand, asynchronous programs can benefit from vertical scaling, which is a quicker process, to enable one server to increase the number of requests the server can handle.
Disadvantages
Cons of Asynchronous Programming Asynchronous programming may seem like the obvious solution to any bottlenecks that may appear in your software development projects.
But there are reasons why developers avoid using asynchronous programming. Check them out.
- Complexity Developers need to be well-versed in callbacks and recursive functions in order to successfully program asynchronous tasks. Even if they do, programming this into an application during development can be difficult. The entire software development lifecycle will become more intricate and notably slower. The code itself can become complex for the same reason. Testing and debugging are equally demanding tasks as writing clean code gets more challenging.
- Latency The initial rendering of a page can take some time, even though page refreshing is less of a problem with asynchronous programming than with synchronous programming. Even though you receive concurrency in return, too many asynchronous requests can overload your server and cause your program to run more slowly.
Synchronous vs Asynchronous
Synchronous
Any process with several tasks that must be completed in order and that don't take up a lot of time should employ synchronous callbacks. For instance: You're in line for movie tickets, but you can't receive one until the people in front of you do.
Asynchronous
When the tasks are not dependent on each other and may take some time for the executant we should use Asynchronous callbacks. For example: When you order your food other people can also order their food in the restaurant. They don’t have to wait for your order to finish, If you’re downloading a song from the internet, Getting an API response.
Learn More
To learn more about Asynchronous Programming read here
Conclusion
- Parallel programming in the form of asynchronous programming enables a task to run independently of the main application thread
- By leveraging several processors simultaneously, parallel programming enables developers to use multicore computers to speed up the performance of their applications
- Several ways to achieve async in java are thread, completeableFutures, futuretasks, and ea async,springevents, and microservices.
- Advantages of async programming are improved User Experience, Customization,Scalability
- Disadvantages of async programming are complexity and latency
- When the tasks are not dependent on each other and may take some time for execution we should use Asynchronous callbacks.