Java ByteBuffer
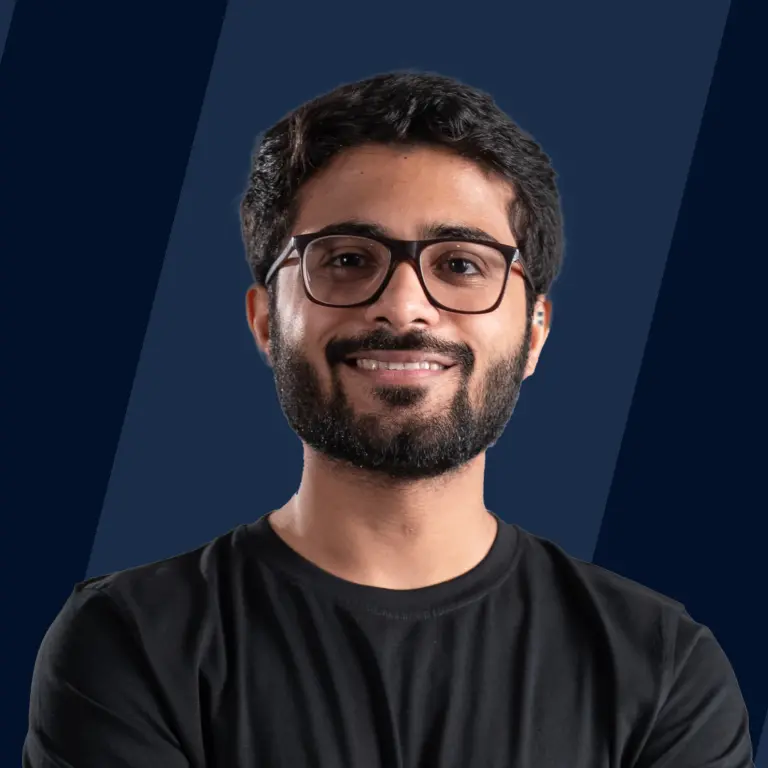
Overview
The Java bytebuffer is used to hold the sequence of integer values that can be used for input/output operations. The java bytebuffer is a part of Java Buffer classes that are the foundation that is used to build Java NIO. The java bytebuffer is the most preferred among these buffer classes.
Introduction to Java ByteBuffer
As discussed earlier, the Java bytebuffer is used to hold the sequence of integer values that can be used for I/O operations.
The java bytebuffer class can provide the following operations on the long buffers:
- The java bytebuffer class provides absolute and relative get methods that read single bytes.
- The java bytebuffer class provides absolute and relative put methods that write single bytes.
- The java bytebuffer class provides relative bulk put and get methods that transfer contiguous sequences of bytes from an int array or some other bytes buffer into this buffer and from this buffer into an array.
The java bytebuffer class also provides the following methods to create short buffers:
- allocate(): The java bytebuffer class provides the allocate() method that is used to allocate spaces for the buffer’s content.
- wrap(): The java bytebuffer class provides the wrap() method that is used to wrap an existing long array into a buffer.
Declaration
In this section we will learn how to declare java bytebuffer:
Syntax
Direct vs. Non-direct Buffers
The java bytebuffer can be categorized as either a direct buffer or a non-direct. A direct buffer refers to a buffer's underlying data allocated on a memory area where OS functions can directly access it. A non-direct buffer refers to a buffer whose underlying data is a byte array that is allocated in the Java heap area.
The main difference between direct and non-direct buffers is that in the case of non-direct buffers, the memory location is simply a wrapper around byte array and it resides in Java Heap memory whereas in the case of direct byte buffer the memory location is outside of JVM and memory is not allocated from the heap.
In the case of a direct byte buffer, the native I/O operations are directly performed upon it by the Java virtual machines in order to restrict the duplicity of the content of the buffer to an intermediate buffer before each invocation of one of the underlying operating system's native I/O operations.
note: The isDirect method in java can be used to check whether a byte is a buffer or not. The Java isDirect method provides explicit buffer management for performance-critical codes.
Access to Binary Data
This class defines methods for reading and writing values of all other primitive types, except boolean.
The java bytebuffer class helps us to define methods to read and write values for all the primitive types (except for the boolean types). The instance of ByteOrder classes is used to represent specific byte orders. The initial order of a byte buffer is always BIG_ENDIAN.
For access to heterogeneous binary data, that is, sequences of values of different types, this class defines a family of absolute and relative get and put methods for each type.
The java bytebuffer class is used to define a family of relative and absolute put and get methods for access to each type of heterogeneous binary data.
For 32-bit floating-point values, for example, this class defines:
The given methods are defined for primitive data types int, long, short, double, and char.
Invocation Chaining
Methods in this class that do not otherwise have a value to return are specified to return the buffer upon which they are invoked. This allows method invocations to be chained. The sequence of statements
The java bytebuffer allows method invocations to be chained. This happens because methods in this class otherwise do not have a value to return, thus they are specified to return the buffer upon which they are invoked.
The following is the sequence of terms:
Here 'exp' is a java bytebuffer.
Method Summary of Java ByteBuffer
Modifier and Type | Method | Description |
---|---|---|
static ByteBuffer | allocate(int capacity) | Allocates a new byte buffer |
static ByteBuffer | allocateDirect(int capacity) | Allocates a new direct byte buffer |
byte[] | array() | Returns the byte array that backs this buffer (optional operation). |
int | arrayOffset() | Returns the offset within this buffer's backing array of the first element of the buffer (optional operation). |
abstract CharBuffer | asCharBuffer() | Creates a view of this byte buffer as a char buffer. |
abstract DoubleBuffer | asDoubleBuffer() | Creates a view of this byte buffer as a double buffer. |
abstract FloatBuffer | asFloatBuffer() | Creates a view of this byte buffer as a float buffer |
abstract IntBuffer | asIntBuffer() | Creates a view of this byte buffer as an int buffer. |
abstract LongBuffer | asLongBuffer() | Creates a view of this byte buffer as a long buffer. |
abstract ByteBuffer | asReadOnlyBuffer() | Creates a new, read-only byte buffer that shares this buffer's content. |
abstract ShortBuffer | asShortBuffer() | Creates a view of this byte buffer as a short buffer. |
abstract ByteBuffer | compact() | Compacts this buffer (optional operation). |
int | compareTo(ByteBuffer that) | Compares this buffer to another |
abstract ByteBuffer | duplicate() | Creates a new byte buffer that shares this buffer's content. |
boolean | equals(Object ob) | Tells whether or not this buffer is equal to another object. |
abstract byte | get() | Relative get method. |
ByteBuffer | get(byte[] dst) | Relative bulk get method. |
ByteBuffer | get(byte[] dst, int offset, int length) | Relative bulk get method. |
abstract byte | get(int index) | Absolute get method |
abstract char | getChar() | Relative get method for reading a char value. |
abstract char | getChar(int index) | Absolute get method for reading a char value. |
abstract double | getDouble() | Relative get method for reading a double value |
abstract double | getDouble(int index) | Absolute get method for reading a double value. |
abstract float | getFloat() | Relative get method for reading a float value. |
abstract float | getFloat(int index) | Absolute get method for reading a float value |
abstract int | getInt() | Relative get method for reading an int value. |
abstract int | getInt(int index) | Absolute get method for reading an int value. |
abstract long | getLong() | Relative get method for reading a long value |
abstract long | getLong(int index) | Absolute get method for reading a long value |
abstract short | getShort() | Relative get method for reading a short value |
abstract short | getShort(int index) | Absolute get method for reading a short value. |
boolean | hasArray() | Tells whether or not this buffer is backed by an accessible byte array. |
int | hashCode() | Returns the current hash code of this buffer. |
abstract boolean | isDirect() | Tells whether or not this byte buffer is direct |
ByteOrder | order() | Retrieves this buffer's byte order. |
ByteBuffer | order(ByteOrder bo) | Modifies this buffer's byte order. |
abstract ByteBuffer | put(byte b) | Relative put method (optional operation). |
ByteBuffer | put(byte[] src) | Relative bulk put method (optional operation) |
ByteBuffer | put(byte[] src, int offset, int length) | Relative bulk put method (optional operation). |
ByteBuffer | put(ByteBuffer src) | Relative bulk put method (optional operation). |
abstract ByteBuffer | put(int index, byte b) | Absolute put method (optional operation). |
abstract ByteBuffer | putChar(char value) | Relative put method for writing a char value (optional operation). |
abstract ByteBuffer | putChar(int index, char value) | Absolute put method for writing a char value (optional operation) |
abstract ByteBuffer | putDouble(double value) | Relative put method for writing a double value (optional operation). |
abstract ByteBuffer | putDouble(int index, double value) | Absolute put method for writing a double value (optional operation). |
abstract ByteBuffer | putFloat(float value) | Relative put method for writing a float value (optional operation). |
abstract ByteBuffer | putFloat(int index, float value) | Absolute put method for writing a float value (optional operation). |
abstract ByteBuffer | putInt(int value) | Relative put method for writing an int value (optional operation). |
abstract ByteBuffer | putInt(int index, int value) | Absolute put method for writing an int value (optional operation). |
abstract ByteBuffer | putLong(int index, long value) | Absolute put method for writing a long value (optional operation). |
abstract ByteBuffer | putLong(long value) | Relative put method for writing a long value (optional operation). |
abstract ByteBuffer | putShort(int index, short value) | Absolute put method for writing a short value (optional operation). |
abstract ByteBuffer | putShort(short value) | Relative put method for writing a short value (optional operation). |
abstract ByteBuffer | slice() | Creates a new byte buffer whose content is a shared subsequence of this buffer's content. |
String | toString() | Returns a string summarizing the state of this buffer. |
static ByteBuffer | wrap(byte[] array) | Wraps a byte array into a buffer. |
static ByteBuffer | wrap(byte[] array, int offset, int length) | Wraps a byte array into a buffer. |
Java ByteBuffer Examples
In this section, we will go through different examples to learn the working of java bytebuffer.
Example 1
In this example, we will try to understand the working of java bytebuffer by creating and displaying a java bytebuffer of a certain size that contains numeric values.
Code:
Output:
Explanation of the example:
In the above example, we are defining the size of the java bytebuffer. After that, we are creating a try-catch block and within the try block, we are defining the bytebuffer object exp and allocating it to the buffer size. Then we are inserting the typecast values using the put method. After that, the buffer is displayed which outputs [10, 20, 30, 40]. The catch block is to check the possibilities of errors and to handle them.
Example 2
In this example, we will try to understand the working of java bytebuffer by creating and displaying a java bytebuffer of a certain size that contains a string.
code:
Output:
Explanation of the example:
In the above example, we are defining the size of the java bytebuffer. After that, we are creating a try-catch block and within the try block, we are defining the bytebuffer object exp and allocating it to the buffer size. Then we are setting the bytebuffer to char inserting a string value using the put method. After that, the buffer is displayed which outputs H e l l o W o r l d. The catch block is to check the possibilities of errors and to handle them.
Conclusion
- The java bytebuffer holds a sequence of integer values for input/output operations.
- The java bytebuffer is a part of the Java Buffer class.
- The java bytebuffer class provides methods to create short buffers and long buffers.
- The java bytebuffer can be categorized as either a direct buffer or a non-direct buffer.