Java Catch Multiple Exceptions
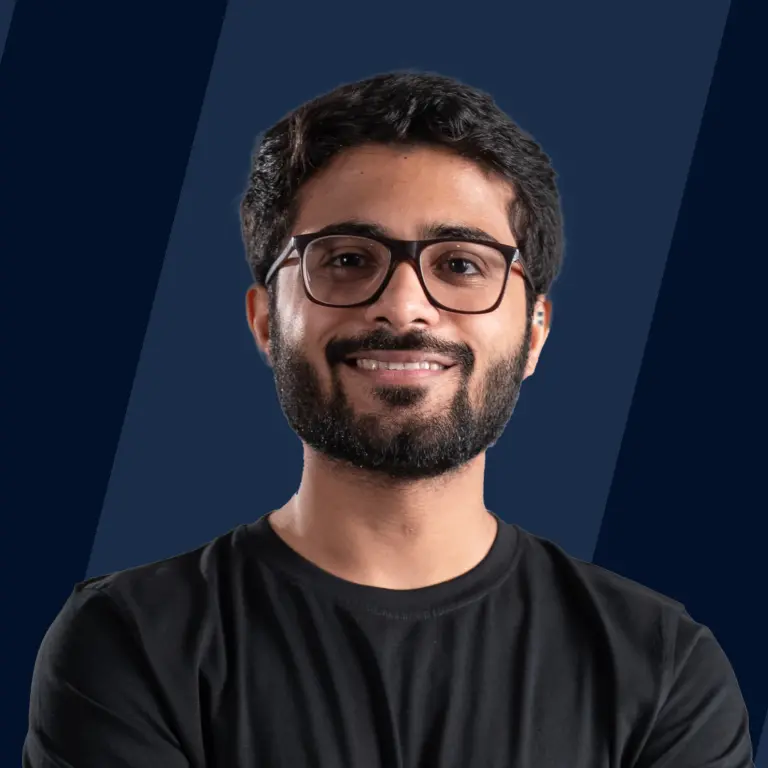
Overview
Java offers a variety of catch blocks to handle different exception types. Before Java 7 was published, we needed a unique catch block to handle a specific exception. As a result, there were extraneous code blocks and a poor plan of action. We can see that a single catch block can handle many exception types as of Java SE 7 and beyond. This feature can limit the temptation to catch an excessively broad exception and reduce code duplication.
Introduction
You must be familiar with the fundamentals of Java exception handling before learning about catching numerous exceptions. Let's look at this briefly.
One effective method for handling runtime problems in Java is called Exception Handling. By using this method, the application's normal flow can be preserved.
The root class of the Java Exception hierarchy is the java.lang.Throwable class, which is inherited by the two subclasses Exception and Error. Below is a list of the Java Exception classes in ascending order:
Checked and unchecked exceptions are the two main categories. Errors are regarded as unchecked exceptions. Oracle claims that there are three different categories of exceptions, specifically:
1) Checked Exception: Checked exceptions are any classes, excluding RuntimeException and Error, that directly inherit the Throwable class. IOException, SQLException, etc., are a few examples. Compile-time checks are performed on checked exceptions.
2) Unchecked Exception: Unchecked exceptions are classes that inherit the RuntimeException. For instance, ArrayIndexOutOfBoundsException, NullPointerException, and so forth. Unchecked exceptions are checked at runtime rather than during compilation.
3) Error Error cannot be corrected. OutOfMemoryError, VirtualMachineError, AssertionError, and other problems are examples of errors.
Five keywords are available in Java that is used to manage exceptions. Each is described in the following table.
Keyword | Description |
---|---|
try | The "try" keyword is used to designate a block in which an exception code should be placed. That means try block cannot be used by itself. The catch or final block must come after the try block. |
catch | The exception is dealt with using the "catch" block. It must be preceded by a try block which means we can't use a catch block alone. It can be followed by finally block later. |
finally | The program's necessary code is executed using the "finally" block. Whether or whether an exception is handled, it is still executed. |
throw | The "throw" keyword is used to throw an exception. |
throws | Declaring exceptions requires the use of the keyword "throws." It makes clear that an exception could happen while using the procedure. It doesn't raise an error. It always goes along with the method signature. |
There are a few examples mentioned where unchecked exceptions might happen. These are what they are:
1) A scenario where ArithmeticException occurs Any number divided by zero results in an ArithmeticException.
2) A scenario where NullPointerException occurs Any operation on a variable that contains a null value will result in a NullPointerException.
3) A scenario where NumberFormatException occurs Any inappropriate formatting of a variable or number may cause a NumberFormatException. Consider a string variable that contains characters; converting it to a digit will result in a NumberFormatException.
4) A scenario where ArrayIndexOutOfBoundsException occurs The ArrayIndexOutOfBoundsException is raised when an array becomes larger than it should. Other causes for ArrayIndexOutOfBoundsException could exist. Take into account the following claims.
Now that you know about exception handling in Java, let's get back to catching multiple exceptions.
Flowchart of Multi-catch Block
Java Program to Handle Multiple Exceptions in a Catch Block
In Java 7, we can catch both these exceptions in a single catch block as:
The pipe symbol (|) can be used to divide up a catch block that handles numerous exceptions. In this example, the exception argument (ex) is final, meaning that it cannot be changed. This feature produces less code repetition and smaller byte code.
Its syntax is:
Example
Output
There are two possible exceptions in this instance:
- We are attempting to divide an integer by 0, which results in an ArithmeticException.
- ArrayIndexOutOfBoundsException because we are attempting to assign a value to index 10 while declaring a new integer array with array bounds of 0 to 9. We are using duplicate code by publishing the exception message in both catch blocks.
Since the assignment operator = has right-to-left associativity, an ArithmeticException is first raised with the message / by zero.
Coding duplication is decreased and efficiency is increased when numerous exceptions are caught in a single catch block.
As there is no code repetition, the bytecode produced when this program is compiled will be smaller than the bytecode produced when the program has numerous catch blocks.
The catch parameter is implicitly final if a catch block manages several exceptions. This means that we are unable to give the catch parameters and values.
Java Program Using Separate Catch Blocks
Let's see how we can use separate catch blocks in Java
Output
As you can see, in the example above, when an exception is thrown, a different block is run. Using the same block of code to capture exceptions of various sorts is a more effective technique to handle multiple exceptions.
Java Program Using Multiple Catch Blocks
Before Java 7, we used to catch multiple exceptions one by one as shown below.
Let's take an example to understand how to handle multiple exceptions in Java
Output
Because of the code we wrote in the try block, which throws an ArithmeticException, the first catch block in the example above was performed (because we divided the number by zero).
Let's alter the code a little bit now to see how the output changes:
Output
In this instance, the code raises an ArrayIndexOutOfBoundsException, which causes the second catch block to be activated. In the program above, we are attempting to access the array's eleventh element, although the array's size is only seven.
What can we learn from the two situations above?
- It is evident that the specific catch block (that declares the exception) gets executed when an exception occurs. This explains why the first block in the first example is executed and the second catch in the second.
- Although I did not present it to you above, the final generic catch handler would be called if the code above encountered an error that was not ArrayIndexOutOfBounds or Arithmetic.
Let's alter the code once more to observe the results:
Output:
Why did we encounter this error?
This is so that none of the catch blocks placed after the general exception catch block, which was placed initially, may be reached. This block should always come after any other particular exception catch blocks.
More Examples of Catching Multiple Exceptions
At a time only one exception occurs and at a time only one catch block is executed. All catch blocks must be ordered from most specific to most general, i.e. catch for ArithmeticException must come before catching for Exception.
- If all the exceptions belong to the same class hierarchy, we should be catching the base exception type. However, to catch each exception, it needs to be done separately in their catch blocks.
- Single catch block can handle more than one type of exception. However, the base (or ancestor) class and subclass (or descendant) exceptions can not be caught in one statement. For Example
- All the exceptions must be separated by vertical bar pipe "|".
Example 1
Let's see a simple example of a java multi-catch block.
Output:
As we can see here the try block is followed by three catch blocks that contain a different exception handler, the first catch block handles ArithmeticException, the second one handles ArrayIndexOutOfBoundsException and finally, the third one handles Exception We can use multi-catch block in cases where we are required to perform multiple different tasks at the occurrence of different exceptions. Here is the output, we can see this program catches the arithmetic exception since we did
in the try block which is undefined
Example 2
Let's see another example in Java
Output:
Two exceptions are present in the try block in this example. However, only one exception happens at a time, and its associated catch block is performed Here we can see the array's size is 5 and despite that, we're doing
and so we get ArrayIndexOutOfBounds Exception
Let's now see an example of what happens when we have multiple errors in our program
Output:
Here, in this program we can see that despite the fact we're printing a[10] when array a's value was 5, we're not getting the ArrayOutOfBounds exception here this is because the arithmetic exception a[5]=30/0; occurs right before it and the catch block catches it and doesn't execute the code after it in the try block
Example 3
Let's see another example where we'll see where and how the Parent exception class will come into the picture
Output
In this example, we generated the exception type NullPointerException, but we didn't supply it. The catch block containing the parent exception class Exception will be called in this situation
Example 4
Let's see an example, to handle the exception without maintaining the order of exceptions (i.e. from most specific to most general).
Output:
Java Program to Catch the Base Exception
The rule changes from general to specialized when catching multiple exceptions in a single catch block.
This means that rather than catching numerous specialized exceptions, we can only catch the base exception if there is a hierarchy of exceptions in the catch block.
Let’s take an example.
Example: Catching Base Exception Class Only
Output
We are aware that the class Exception is a subclass of all exception classes. Therefore, we only need to catch the Exception class rather than many customized exceptions.
Use of child exception classes within the same catch block is not permitted if the base exception class has already been provided. Otherwise, a compilation error will occur.
Let’s take an example.
Example: Catching Base and Child Exception Classes
Output
In this example, ArithmeticException and ArrayIndexOutOfBoundsException are both subclasses of the Exception class. So, we get a compilation error
Java Program to Rethrow Exceptions
Additionally enhanced is the compiler's evaluation of rethrown exceptions. The Java rethrow exception allows you to specify more specific exception types in the throws section of a method declaration. We'll provide a clear example to demonstrate this:
As you can see, the catch block in the rethrow method is catching Exceptions but is not included in the throws clause. To determine what kinds of exceptions are thrown and subsequently rethrown from the catch block, the Java 7 compiler examines the entire try block. Keep in mind that if you modify the catch block parameter, this analysis is removed
Conclusion
- A single catch block can handle many exception types as of Java SE 7 and beyond. This feature can limit the temptation to catch an excessively broad exception and reduce code duplication
- One effective method for handling runtime problems in Java is called Exception Handling. By using this method, the application's normal flow can be preserved
- The root class of the Java Exception hierarchy is the java.lang.Throwable class, which is inherited by the two subclasses Exception and Error
- The pipe symbol (|) can be used to divide up a catch block that handles numerous exceptions
- The Java rethrow exception allows you to specify more specific exception types in the throws section of a method declaration
- attempting to divide an integer by 0, which results in an ArithmeticException
- Errors are regarded as unchecked exceptions.
- The ArrayIndexOutOfBoundsException is raised when an array becomes larger than it should