Java Cheat Sheet
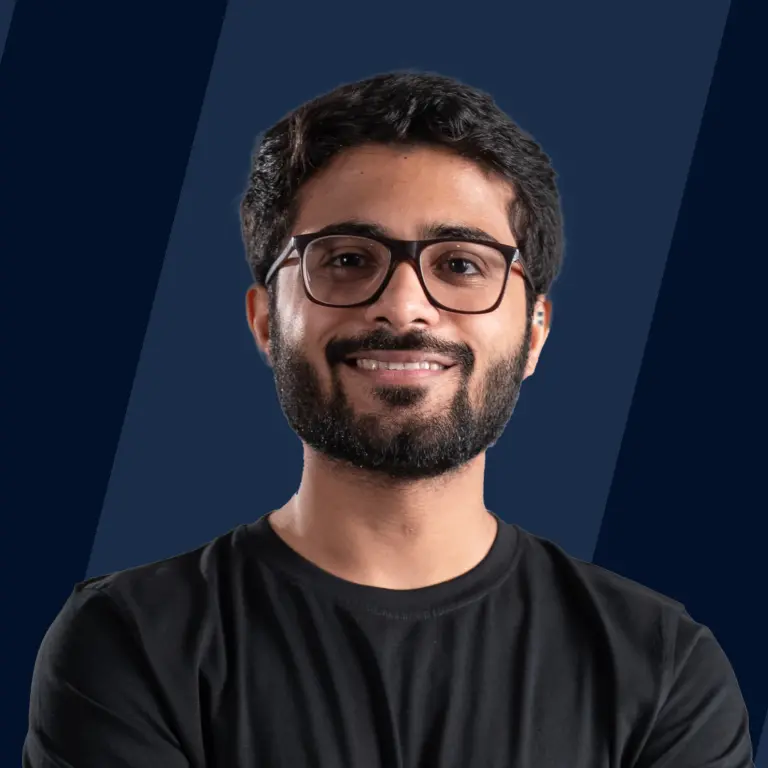
Overview
Java is a high-level programming language well-known for its dependability, object-orientedness, improved security, and simple-to-learn characteristics. Originally, it was called by the name Oak. Java is an object-oriented language that emphasizes eliminating dependencies.
Java Cheat Sheet
Java Terminology
In this part, we'll look at the most important terms, related to Java.
1. JVM: JVM is an abbreviation for Java Virtual Machine. The execution of a program is broken down into three phases: a program is created, and then it is built and executed.
- A Java program is made by a programmer.
- The code is compiled using the JAVAC compiler, a primary Java compiler included in the Java development kit (JDK).
- During the running phase of the program, JVM executes the bytecode created by the compiler.
2. Bytecode: After the source code has been produced, the compiler produces a sort of intermediate code called bytecode. This intermediary code makes Java a cross-platform language.
3. JRE: The JDK comes with the Java Runtime Environment (JRE). We can run the Java software on our PCs thanks to the JRE installation. JRE comes with a browser, a JVM, applet support, and plugins.
4. Java Development Kit (JDK): It is a full Java development kit whthatomes with everything from the compiler to the Java Runtime Environment (JRE), debuggers, and documentation. Installing JDK on a computer is a must for designing, compiling, and running Java applications.
Java Basics
- Classes and objects
- Keywords
We will learn about the above two topics in detail, further in the article.
- Constructors A Java constructor is a section of code similar to a method. The constructor is called whenever a new instance of the class is created. Memory for the object is not allocated until the constructor is called.
There are two types of constructors in Java:
(i) Default Constructor: A constructor with no parameters is referred to as a default constructor. The compiler creates a default constructor for the class with no arguments if we don't declare a constructor for it.
Syntax:
(ii) Parameterised Constructor: A constructor with parameters is referred to as a parameterized constructor. Use a parameterized constructor if you want to initialize the fields of the class with your values.
Syntax:
Java Variables
Variables in Java are containers that hold values. These variables are used to reference the values they hold during the execution of a program. Also, variables need to be initialised or defined, before they can be used.
Syntax:
Variables are of three types:
1. Local Variables: Variables that can only be used inside the method, block, or constructor's body, where they are declared and initialized.
2. Global/Instance Variables: Variables that are declared inside the class but outside of the method, block or constructor.
3. Static Variables: Declared using the "static" keyword, the static variables are created after the program is first executed. They are shared by all objects of the class and are destroyed as the program terminates.
Java Datatypes
There are two data types, namely primitive and non-primitive.
1. Primitive data types: Primitive data types are those data types that are pre-built into a programming language and are the foundation for more complex data types.
There are 8 primitive data types in Java:
Data Type | Size | Description |
---|---|---|
int | 4 bytes | Used to store numeric values. Its values vary from -2^31^ to (2^31^ -1). |
char | 2 bytes | A character using Unicode encoding is represented by the char data type. Its range lies from 0 to 65,535. |
boolean | 1 bit | It is a very commonly used data type that comes in handy in regulating the flow of a program. It has only two possible values, true and false. |
double | 8 bytes | The double data type is used to represent fractional numbers in Java and can store both positive and negative values. This decimal number has double precision. Its values vary from 4.9406564584124654 x 10^-324^ to 1.7976931348623157 x 10^308^. |
float | 4 bytes | It's similar to double data type, except the decimal number has single precision. Its values range from 1.40239846 x 10^-45^ to 3.40282347 x 10^38^. |
long | 8 bytes | Long is quite similar to int but can hold a larger set of values. Its range varies from -2^63^ to (2^63^ – 1) |
byte | 1 byte | It's similar to int, except a byte can only hold the values from -2^7^ to (2^7^ – 1). |
short | 2 bytes | The type short, which sits in between byte and int, can be used if we wish to save memory and the byte is too little. Its range varies from -2^15^ to (2^15^ – 1). |
2. Non-primitive data types: They are created by the programmer and are used to invoke certain operations. Classes, interfaces, and arrays are the common non-primitive data types.
You can learn more about Primitive and Non-Primitive Data types from these articles.
Typecasting in Java
When you assign a value from one basic data type to another, that is known as type casting. There are two types of typecasting in Java: widening and narrowing.
1. Widening type casting: Conversion of a smaller data type to a larger one. 2. Narrowing type casting: Conversion of a larger data type to a smaller one.
You can learn more about typecasting in Java from Typcasting in Java article.
Keywords in Java
Keywords are Java predefined words, which have special functions. So, they cannot be used as variables, objects, or class names. Some commonly used keywords in Java include abstract, boolean, class, char, default, double, final, enum, and other words that have some specific predefined usage in Java.
Please follow this article - What is Keyword in Java? to find the list of all keywords in Java.
Comments in Java
Java comments are the parts of a program that the compiler and interpreter do not execute. Java code can be made more comprehensible and readable by using comments. By providing the specifics of the code involved, comments in a program help to make it more human-readable, and good use of comments facilitates maintenance and bug discovery.
Comments can be single-line or multi-line.
Single-line comment
Multi-line comment
Javadoc (Because it helps produce a documentation page, this kind of comment is frequently used while writing code for a project or software product.)
Java Access Modifiers
The accessibility or scope of a field, function, constructor, or class is specified by the access modifiers in Java. By using the access modifier, we can modify the access level of fields, constructors, methods, and classes. Here is a description of the four access modifiers in Java:
1. Private: A private modifier has class-specific access levels. It is inaccessible to users outside the class.
2. Default: A default modifier's access level is restricted to the package. It is inaccessible from the outside of the box. If you don't choose an access level, the default will be used.
3. Protected: A protected modifier has access both inside the package and outside the package through a child class. The child class cannot be accessed from outside the package if it is not created.
4. Public: A public modifier's access level can be found everywhere. It is accessible from both inside and outside of the class as well as from both inside and outside of the package.
Please follow this article Access Modifiers in Java to learn about access modifiers in Java in depth.
Identifiers in Java
Identifiers are used to identify things. An identifier in Java could be a variable name, class name, method name, or label.
Example:
In the above example, there are 5 identifiers. They are:
- Car : class name.
- main : method name.
- String : predefined class name.
- args : variable name.
- car1 : variable name.
Operators in Java
The symbols used to perform various operations in Java are called operators.
Let's understand each of these types in brief:
Operator | Description | Symbols |
---|---|---|
Unary | Only one operand is needed for the Java unary operators. Unary operators are used to carry out a variety of operations, including adding or subtracting one from a value, negating an expression, and inverting a boolean value. | ++, --, +, -, ! |
Arithmetic | To accomplish addition, subtraction, multiplication, and division, Java arithmetic operators are utilized. They serve as fundamental operations in mathematics. | +, – , *, / , % |
Assignment | The assignment operator in Java is among the most popular operators. The operand on its left is used to assign the value on its right to the operand. | =, +=, -=, *=, /=, %=, &=, ^= |
Relational | These operators are employed to check for greater-than, less-than, and equality relationships. They commonly appear in looping and conditional if-else expressions and, after comparison, return a boolean result. | >, <, >=, <=, ==, != |
Logical | The "logical AND" and "logical OR" operations carried out by these operators are comparable to the AND and OR gates used in digital electronics. One thing to keep in mind is that the second condition is not looked at if the first condition is false. | &&, || |
Ternary | In Java programming, the ternary operator is frequently used as a one-line if-then-else statement substitute. The only conditional operator that requires three operands is this one. | ?: |
Bitwise | These operators are used to individually alter a number's bits. Any integer type is amenable to their use. They are employed for querying and updating Binary indexed trees. | &, | |
Shift | These operations multiply or divide an integer by two by shifting its bits to the left or right, respectively. They can be used to multiply or divide a number by two. | <<, >>, >>> |
Methods in Java
A method is a section of code that only executes when invoked. Data can be passed into a method as parameters. Methods usually referred to as functions, are used to carry out specific actions.
Syntax:
Control Flow in Java
Java has statements that can be used to regulate how Java code is written. These sentences are referred to as control flow statements. There are three types of control statements:
1. Decision-Making statements Decision-making statements determine which statement to run and when. Depending on the outcome of the condition given, decision-making statements assess the boolean expression and influence the program flow. Following are the two types of decision-making statements:
- if statements
- switch statement
2. Loop statements A set of instructions can be carried out repeatedly using loop statements. A specific circumstance must exist for loop statements to work. Every time the loop's state is tested, a condition is checked. Until the condition is false, execution keeps going. Either true or false must be returned as a boolean value. It's an optional requirement. There are three types of loops in Java:
- do while loop
- while loop
- for loop
3. Jump statements Jump statements are used to move program control to the targeted statements. Jump statements effectively move execution control to another area of the program. There are two types of jump statements in Java:
- break statement
- continue statement
Object and Class in Java
A class serves as a model or blueprint from which objects can be created. In other words, it is a group of objects with similar characteristics.
An object is a representation of a real-world entity. In a typical Java application, numerous objects are created, and as you are aware, these objects interact by calling methods.
To learn more about classes and objects in Java, check out our dedicated article here.
Packages in Java
A Java package is a collection of sub-packages, interfaces, and classes of a common type. In Java, there are two types of packages: built-in packages and user-defined packages.
Java Polymorphism
Polymorphism in Java is a feature that enables us to carry out a single operation in a variety of ways. The Greek words poly and morphism denoting "many" and "forms" respectively, combine to form polymorphism. Therefore, polymorphism indicates the existence of multiple types. Compile-time polymorphism and runtime polymorphism are the two categories of polymorphism in Java.
Runtime Polymorphism:
A call to an overridden method is resolved at runtime rather than at compile time using a process known as runtime polymorphism or dynamic method dispatch.
Example:
Output:
Compile-time Polymorphism:
Compile-time polymorphism is achieved using function overloading in Java. It is also known as static polymorphism.
Function Overloading: Overloaded functions are those that have numerous instances of the same name but distinct parameters. A change in the number of arguments or/and a change in the type of arguments might cause a function to be overloaded.
Example:
Output:
Inheritance in Java
Inheritance is a crucial pillar of OOP (Object-Oriented Programming). The process in Java that allows one class to inherit the characteristics (fields and methods) of another class is known as inheritance.
Important Terms:
Superclass: A superclass is a class from which features are inherited (or a base class or a parent class).
Subclass: A subclass is a class that inherits from another class (or a derived class, extended class, or child class). In addition to the attributes and methods of the superclass, the subclass may also add additional fields and methods.
Reusability: The idea of "reusability" is supported by inheritance; for example, if we want to construct a new class but an existing class already contains some of the code we need, we can derive our new class from the old class. We are utilizing the fields and methods of the pre-existing class by doing this.
Syntax:
You can follow this article - Java Inheritance to learn more about Inheritance in Java.
Data Abstraction and Encapsulation
Abstraction is the technique of hiding implementation specifics from the user and only displaying functionality. In other words, it hides the internal information and just displays what is necessary to the user. In Java, data abstraction can be achieved in two ways:
- Abstract class
- Interface
The process by which data and methods are combined into a single entity in Java is known as encapsulation. The variables of a class are protected from access by another class by encapsulation and only the class's methods have access to the variables. A good example of encapsulation would be a capsule, which is made up of a lot of medicines, but the consumer is concerned with just the capsule as a whole.
Abstract Class and Interfaces in Java
An abstract class is declared using the abstract keyword. It can contain both abstract as well as non-abstract methods. It cannot be instantiated and needs to be extended.
Syntax:
Interface in Java is a tool to achieve abstraction. It can only include abstract methods, and method bodies are not allowed. It is used to achieve multiple inheritance and abstraction.
Syntax:
To accomplish abstraction, where we can declare the abstract methods, both abstract classes and interfaces are employed. Both abstract classes and interfaces are non-instantiable.
Math Class in Java
The Java Math class contains numerous methods for performing various mathematical computations. It offers numerous ways to perform mathematical operations, including min(), max(), avg(), sin(), cos(), tan(), round(), ceil(), floor(), abs(), and more.
Java Arrays
Instead of defining different variables for every value, arrays are used to hold many values in a single variable. Put square brackets around the variable type to declare it as an array. Java uses dynamic allocation for all arrays. Each variable in the array is sorted, with indexing beginning at 0.
Syntax:
Java Strings
A group of characters enclosed in double quotations form a String variable. In Java, a string is an object with methods that allow for certain string operations. Some commonly used string methods are length(), toUpperCase(), toLowerCase(), etc.
Syntax:
Java Regex
A string of characters that creates a search pattern is called a regular expression. You can use this search pattern to specify your search criteria when looking for information in a text. A regular expression might be as simple as one character or as complex as a more intricate pattern. Regex is widely used to define the bounds for strings in a variety of contexts, such as passwords and email validation. Regular expressions are contained in the Java.util.regex package.
There are three classes in this package:
- Pattern Class: A pattern's definition (example: String[] split(), String pattern(), etc)
- Matcher Class: A tool for looking for patterns. (example: boolean matches(), boolean finds(), String group(), etc)
- PatternSyntaxException class: Used for indicating pattern syntax problems in regular expressions.
Example: The example below shows two easy ways to use regex in Java:
Output:
In the above example, we put the pattern to search in the compile() method and the string in the matcher() method as shown in method 1. We can also use method 2, where we just have to provide both the pattern to be matched as well as the string inside the matches() method.
Exception Handling in Java
Java code execution may encounter various problems, including programming faults caused by the programmer, errors brought on by incorrect input, and other unforeseen circumstances. Java typically stops and produces an error message when an error occurs. This is referred to as throwing an exception in Java.
Exception handling:
- You can specify a section of code to be tested for errors as it is being performed using the try statement.
- You can specify a block of code to be executed if an error occurs in the try block using the catch statement.
- Whether or not an exception is handled, code in the finally block is executed.
Note that the terms "try and catch" are paired.
Syntax:
Commands in Java
1. Java -version: One of the most basic Java commands for determining the version of Java that is currently installed on your computer is Java -version. This is also used to ensure that the PATH variable settings and installation are accurate.
2. javac -version: The compiler's current version is shown by this command, which is used to build source code. Additionally, this is a part of the Java Development Kit or JDK.
3. whereis: This Java command looks through the directory for a specific component.
4. echo: Java's echo command enables you to view the contents of a particular file. This is typically used to check the PATH variables.
4. javap: To deconstruct one or more class files, use the javap command. The javap command prints all of the methods and both protected and public fields of the classes it is given as input when no options are given.
5. javah: You may automatically generate c header and source files from a Java class by using this utility. The generated c headers and source files are used to implement native methods and make references to an object's instance variable in the native source code.
6. javadoc: A group of Java source files can be seamlessly converted into HTML pages or API documentation using the Javadoc command and its arguments.
Collections in Java
Java's Collection framework offers an architecture for storing and managing a collection of objects. All data actions, including searching, sorting, insertion, manipulation, and deletion, are possible with Java Collections.
A collection in Java is a group of similar objects. The Java Collection Framework has numerous classes and interfaces such as Set, List, Queue, ArrayList, Vector, LinkedList, PriorityQueue, HashSet, LinkedHashSet, and TreeSet.
Java Generics
Generics are types that have parameters. The goal is to make it possible for methods, classes, and interfaces to take type (Integer, String, etc., and user-defined types) as a parameter. Generics can be used to build classes that interact with various data types. Generics are commonly utilized in classes such as HashSet, ArrayList, HashMap, etc.
Properties
- We can only store one type of object in generics. The storing of objects of various types is not permitted.
- We don't have to typecast the object when we access it while using generics.
- To make sure that a problem does not occur during runtime, generics are tested at the time of compilation.
To create objects of a generic class, use the following syntax:
Multithreading in Java
The smallest processing unit is a thread, which is a lightweight process. Multithreading in Java is the technique of running many threads together at the same time. Context-switching between threads is faster than processing because threads share memory and do not allocate separate memory space.
Explore Scaler Topics Java Tutorial and enhance your Java skills with Reading Tracks and Challenges.
Conclusion
Now, we shall sum up what we learned from the article. We discussed:
- The basics of Java terminology.
- The basic concepts like variables, classes, objects, constructors, data types, and more.
- Focused on important topics like OOPS in Java by exploring its concepts in brief including the four important pillars (inheritance, polymorphism, encapsulation, and abstraction), access modifiers, etc.
- The concept of Java classes like Java.Math class, arrays, strings, and more.
- Some advanced concepts like Java regex, collections, multithreading, generics, etc.